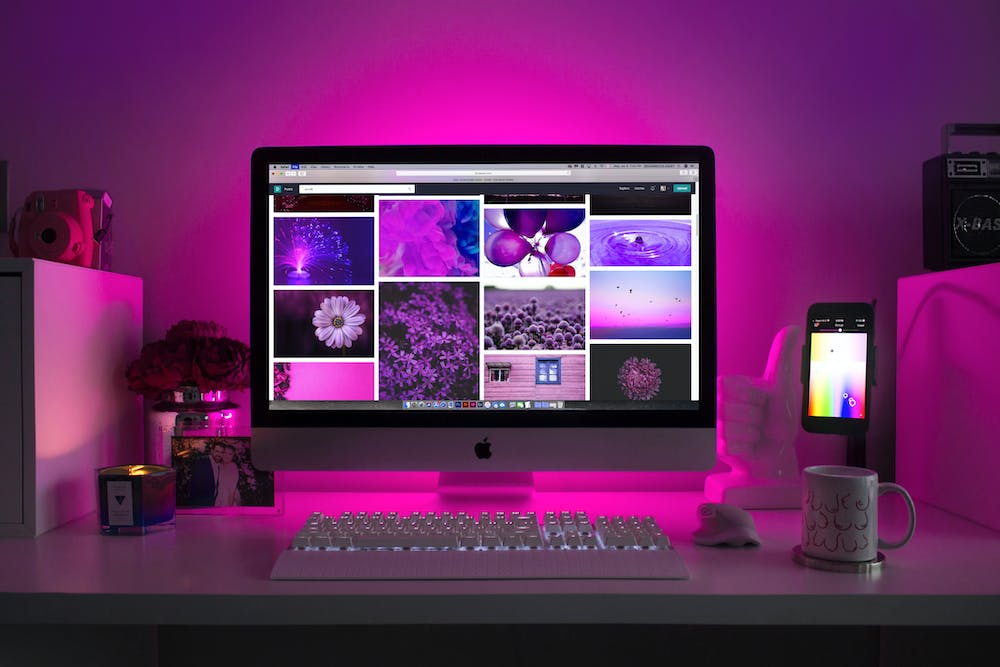
JavaScript is a powerful language that allows developers to create and manipulate lists in various ways. Lists, also known as arrays, are a fundamental data structure in JavaScript and are used to store a collection of elements. In this article, we will explore how to create and manipulate lists in JavaScript, covering topics such as adding and removing elements, iterating over lists, and performing common operations on lists. By the end of this article, you will have a solid understanding of how to work with lists in JavaScript and be able to apply this knowledge to your own projects.
Creating Lists in JavaScript
There are several ways to create lists in JavaScript. The most common method is to use the array literal notation, which involves enclosing a list of elements within square brackets.
“`javascript
let myList = [1, 2, 3, 4, 5];
“`
In the above example, we have created a list called `myList` containing the elements 1, 2, 3, 4, and 5. Lists in JavaScript can contain elements of different data types, including numbers, strings, objects, and even other lists.
Another way to create a list is by using the `Array` constructor function.
“`javascript
let anotherList = new Array(1, 2, 3, 4, 5);
“`
Both of these methods will result in the creation of a list with the same elements. IT is important to note that the index of elements in a list starts from 0, so the first element is accessed using the index 0, the second element with the index 1, and so on.
Adding and Removing Elements from Lists
Once a list has been created, we can add or remove elements from it using various methods. To add elements to the end of a list, we can use the `push` method.
“`javascript
myList.push(6);
“`
After executing the above code, the list `myList` will now contain the element 6 at the end.
To add elements to the beginning of a list, we can use the `unshift` method.
“`javascript
myList.unshift(0);
“`
After executing the above code, the list `myList` will now contain the element 0 at the beginning.
Conversely, to remove elements from the end of a list, we can use the `pop` method.
“`javascript
myList.pop();
“`
After executing the above code, the last element of the list `myList` will be removed.
Similarly, to remove elements from the beginning of a list, we can use the `shift` method.
“`javascript
myList.shift();
“`
After executing the above code, the first element of the list `myList` will be removed.
Iterating Over Lists
Iterating over a list is a common operation in JavaScript, and there are several ways to accomplish this. One of the most common methods is to use a for loop.
“`javascript
for (let i = 0; i < myList.length; i++) {
console.log(myList[i]);
}
“`
In the above code, we use a for loop to iterate over the elements of `myList` and print each element to the console. The `length` property of a list can be used to determine the number of elements it contains, and this value is often used as the stopping condition for the loop.
Another method for iterating over a list is to use the `forEach` method.
“`javascript
myList.forEach(function(element) {
console.log(element);
});
“`
The `forEach` method is a higher-order function that takes a callback function as an argument and calls this function once for each element in the list. This method is often preferred for its simplicity and readability.
Performing Operations on Lists
JavaScript provides a wide range of methods for performing common operations on lists. These methods can be used to search for elements, sort lists, map elements to a new list, and more.
For example, to check if a list contains a specific element, we can use the `includes` method.
“`javascript
console.log(myList.includes(3));
“`
The above code will output `true` if the element 3 is present in the list `myList`, and `false` otherwise.
To sort the elements of a list in ascending order, we can use the `sort` method.
“`javascript
myList.sort((a, b) => a – b);
“`
After executing the above code, the elements of `myList` will be sorted in ascending order.
To map the elements of a list to a new list, we can use the `map` method.
“`javascript
let squaredList = myList.map(element => element * element);
“`
After executing the above code, the list `squaredList` will contain the square of each element in `myList`.
Conclusion
In this article, we have covered various aspects of creating and manipulating lists in JavaScript. We have discussed how to create lists, add and remove elements, iterate over lists, and perform common operations on lists. By understanding these fundamental concepts, you will be well-equipped to work with lists in JavaScript and leverage their power in your own projects. Lists are a versatile and powerful data structure, and mastering them is essential for any JavaScript developer.
FAQs
Q: What is the difference between an array and a list in JavaScript?
A: In JavaScript, the terms “array” and “list” are often used interchangeably to refer to a collection of elements. Technically, however, JavaScript only has arrays, which are a special type of object used to store a collection of elements. The term “list” is more commonly associated with other programming languages, such as Python, where it specifically refers to a linked list or similar data structure.
Q: Can I store different data types in a single list in JavaScript?
A: Yes, JavaScript arrays can store elements of different data types, including numbers, strings, objects, and even other arrays. This flexibility makes arrays a versatile choice for storing complex data structures in JavaScript.
Q: Are there any limitations to the size of a list in JavaScript?
A: In theory, JavaScript arrays can grow to accommodate a large number of elements. However, in practice, the size of an array may be limited by the amount of available memory in the system. It is important to be mindful of memory usage when working with large arrays to avoid performance issues.