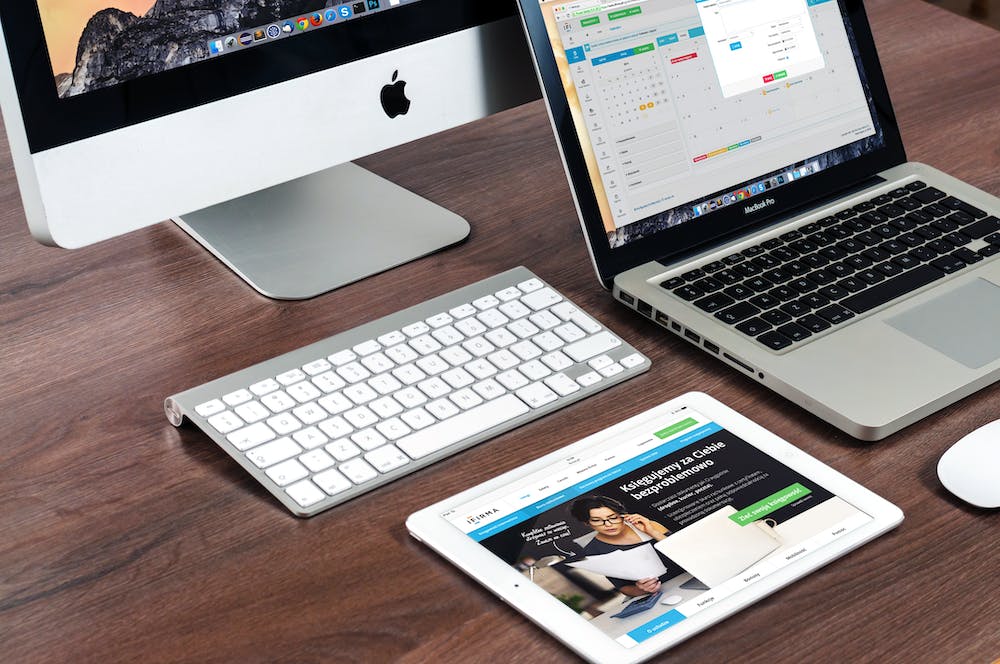
SOAP (Simple Object Access Protocol) is a protocol used for exchanging structured information in the implementation of web services in computer networks. In this article, we will discuss how to create a SOAP client in PHP to interact with SOAP-based web services.
1. What is a SOAP Client?
A SOAP client is a piece of software that makes use of the SOAP protocol to send requests to a SOAP server (also known as a web service) and receives responses from IT. In PHP, you can create a SOAP client to consume web services provided by other systems.
2. Setting Up the Environment
Before we start creating a SOAP client in PHP, we need to ensure that our environment is set up properly. You will need to have PHP installed on your system, as well as a web server such as Apache, Nginx, or any other web server that supports PHP.
Additionally, you will need access to a SOAP-based web service that you can interact with. For the purpose of this tutorial, we will use a public SOAP service provided by backlink works for demonstration. This service is a simple currency conversion web service that we can use to create our SOAP client.
3. Creating the SOAP Client in PHP
To create a SOAP client in PHP, we will use the built-in SoapClient
class, which provides a simple and effective way to interact with SOAP-based web services. The SoapClient
class takes the URL of the SOAP server as a parameter and provides methods to invoke the web service’s operations.
Let’s start by creating a new PHP file called soap_client.php
and writing the following code to create a SOAP client for the currency conversion web service provided by Backlink Works:
// Create a new SoapClient instance
$soapClient = new SoapClient('https://www.backlinkworks.com/currency-converter?wsdl');
// Call the web service's operations
$result = $soapClient->convertCurrency(array('fromCurrency' => 'USD', 'toCurrency' => 'EUR', 'amount' => 100));
// Output the result
echo $result;
?>
Let’s break down the code above:
- We create a new instance of the
SoapClient
class and pass the URL of the SOAP server’s WSDL (Web Services Description Language) file as the parameter. - We then call the
convertCurrency
method of the SOAP client, passing an array of parameters such as the source currency, target currency, and the amount to convert. - We store the result of the web service’s operation in the
$result
variable and output it to the browser.
4. Handling Errors and Exceptions
When working with SOAP clients in PHP, it’s important to handle errors and exceptions that may occur during the interaction with the web service. The SoapClient
class provides methods to catch SOAP faults, handle errors, and debug communication with the SOAP server.
In the code snippet below, we use a try-catch block to handle any exceptions that may be thrown by the SOAP client:
try {
// Create a new SoapClient instance
$soapClient = new SoapClient('https://www.backlinkworks.com/currency-converter?wsdl');
// Call the web service's operations
$result = $soapClient->convertCurrency(array('fromCurrency' => 'USD', 'toCurrency' => 'EUR', 'amount' => 100));
// Output the result
echo $result;
} catch (SoapFault $e) {
// Handle SOAP faults
echo $e->getMessage();
} catch (Exception $e) {
// Handle other exceptions
echo $e->getMessage();
}
?>
In the code above, we use a try-catch block to catch instances of the SoapFault
and Exception
classes. If the SOAP client encounters a fault or exception, we can handle it accordingly by echoing the error message to the browser.
5. Conclusion
In this article, we have discussed how to create a SOAP client in PHP to interact with SOAP-based web services. We have covered the process of setting up the environment, creating a SOAP client using the SoapClient
class, and handling errors and exceptions that may occur during communication with the SOAP server.
Creating a SOAP client in PHP is a powerful way to consume web services provided by other systems and integrate them into your applications. With the built-in SoapClient
class, interacting with SOAP-based web services becomes a straightforward task.
6. FAQs
Q: Can I use the SoapClient
class to interact with any SOAP-based web service?
A: Yes, the SoapClient
class provides a generic way to interact with SOAP-based web services. As long as you have access to the SOAP server’s WSDL file, you can create a SOAP client to consume the web service’s operations.
Q: What are the alternative ways to interact with SOAP-based web services in PHP?
A: Apart from using the SoapClient
class, you can also use the SOAPServer
class to create a SOAP server in PHP. Additionally, you can use PHP’s native curl
library to send SOAP requests and parse SOAP responses.
Q: Is it possible to secure communication between a SOAP client and server?
A: Yes, you can secure communication between a SOAP client and server using HTTPS (SSL/TLS) to encrypt the data transmitted over the network. Additionally, you can use SOAP headers to add security tokens and authentication credentials to SOAP requests.
Q: Can I create a SOAP server in PHP to provide web services to other systems?
A: Yes, you can create a SOAP server in PHP using the SOAPServer
class to expose your application’s functionalities as web services to other systems. This allows other systems to consume your web services using their own SOAP clients.
Thank you for reading our article about creating a SOAP client in PHP. We hope that it has provided you with a solid understanding of the process and has equipped you with the knowledge to start interacting with SOAP-based web services in your PHP applications.