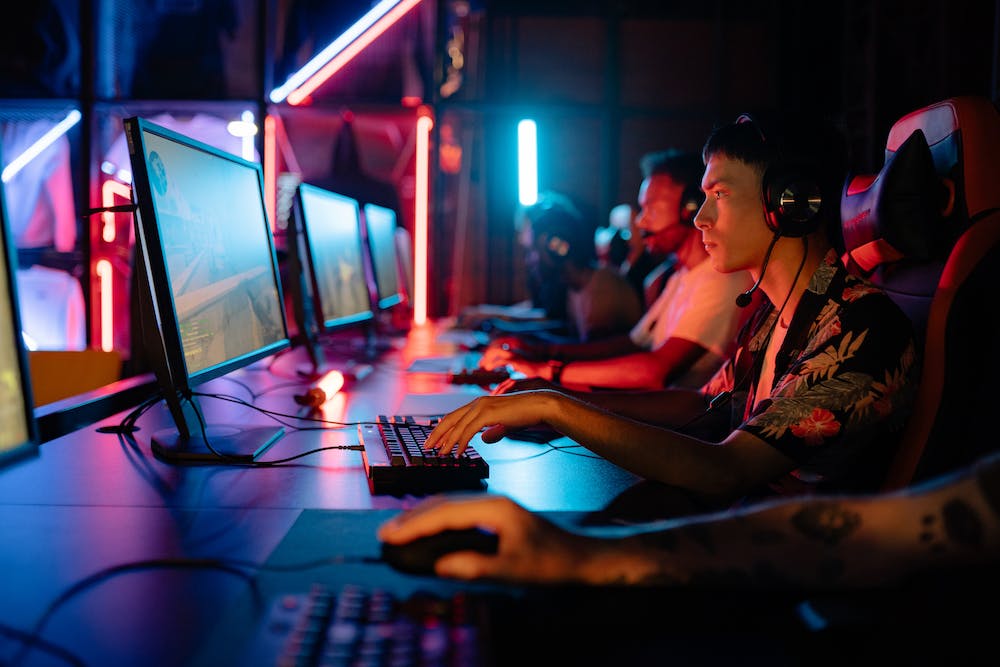
Building a RESTful API with Laravel allows developers to create robust and scalable web services that can be used by a variety of clients, including web, mobile, and IoT applications. In this article, we will explore the steps to building a RESTful API with Laravel and provide examples and best practices along the way.
Getting Started
Before we dive into the technical details of building a RESTful API with Laravel, IT‘s important to have a basic understanding of what RESTful APIs are and why they are important. REST (Representational State Transfer) is an architectural style for designing networked applications. RESTful APIs are designed to be simple, scalable, and stateless, making them ideal for modern web development.
Prerequisites
To build a RESTful API with Laravel, you will need to have the following prerequisites:
- Basic knowledge of PHP and Laravel framework
- Laravel installed on your local machine
- Composer installed for package management
- A database (e.g., MySQL) for storing data
Setting Up the Laravel Project
The first step in building a RESTful API with Laravel is to set up a new Laravel project. You can do this by running the following command in your terminal:
composer create-project --prefer-dist laravel/laravel restful-api
Once the project is set up, you can navigate to the project directory and start building your API.
Creating Routes
In Laravel, routes are defined in the routes/api.php
file. To create routes for your RESTful API, you can use the Route
facade to define various HTTP methods such as GET
, POST
, PUT
, and DELETE
. For example:
Route::get('/posts', 'PostController@index');
Route::post('/posts', 'PostController@store');
Route::put('/posts/{id}', 'PostController@update');
Route::delete('/posts/{id}', 'PostController@destroy');
In the above example, we have defined routes for retrieving all posts, creating a new post, updating a post by its ID, and deleting a post by its ID.
Creating Controllers
Next, you will need to create controllers to handle the logic for your API endpoints. You can create a new controller by running the following command in your terminal:
php artisan make:controller PostController
Once the controller is created, you can define the methods for handling the various HTTP requests. For example:
public function index() {
$posts = Post::all();
return response()->json($posts);
}
In the index
method, we retrieve all posts from the database and return them as JSON. Similarly, you can define methods for creating, updating, and deleting posts.
Handling Authentication
Authentication is an important aspect of building a secure RESTful API. In Laravel, you can use the built-in passport
package to handle API authentication. First, you will need to install the passport
package by running the following command:
composer require laravel/passport
After installing the package, you can run the migration command to create the necessary tables in your database:
php artisan migrate
Next, you can generate the encryption keys used for secure access:
php artisan passport:install
Finally, you can add the necessary middleware to your routes to require authentication for certain endpoints:
Route::middleware('auth:api')->get('/user', function (Request $request) {
return $request->user();
});
Testing the API
Once you have set up your routes, controllers, and authentication, you can begin testing your API using tools such as Postman
or Insomnia
. These tools allow you to send HTTP requests to your API endpoints and validate the responses.
Conclusion
Building a RESTful API with Laravel is a powerful and efficient way to create scalable web services. By following the steps outlined in this article, you can create a robust API that can be used by a variety of clients. With proper authentication and testing, you can ensure that your API is secure and functional.
FAQs
Q: Can I use Laravel to build a RESTful API?
A: Yes, Laravel is a popular PHP framework that provides all the necessary tools and features to build a RESTful API.
Q: What is the role of authentication in a RESTful API?
A: Authentication is important for securing your API and ensuring that only authorized users can access certain endpoints.
Q: How can I test my RESTful API?
A: Tools like Postman and Insomnia allow you to send HTTP requests to your API endpoints and validate the responses.
Q: Can I add custom validation to my API endpoints?
A: Yes, you can use Laravel’s built-in validation features to add custom validation to your API endpoints.
Q: Is IT necessary to use a database with a RESTful API?
A: While IT is not necessary, most RESTful APIs use a database to store and retrieve data.