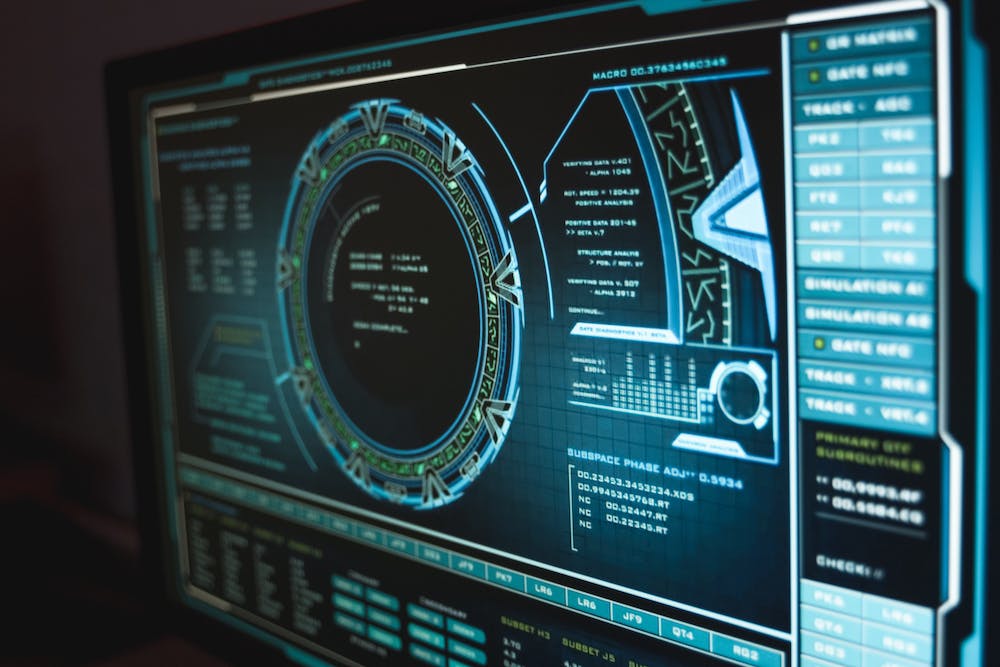
content=”width=device-width, initial-scale=1.0″ />
JavaScript is a versatile programming language that powers the web.
While its dynamic nature allows for flexibility, IT can sometimes lead
to unexpected errors and bugs. To mitigate these issues, TypeScript
was introduced—a statically-typed superset of JavaScript that offers
additional features and type checking. This comprehensive guide will
help you get started with typed JavaScript and enhance your overall
development experience. So, let’s dive in!
1. Setting up TypeScript
To start using TypeScript, you’ll first need to install IT. You can
install TypeScript globally using npm or yarn by running the following
command:
$ npm install -g typescript
Once installed, you can check the installed TypeScript version using:
$ tsc --version
You can now create a new TypeScript file with the
.ts
extension and begin writing typed JavaScript code.
2. Basic TypeScript Syntax
TypeScript introduces type annotations, allowing you to define the
types for variables, function parameters, and return values. For
example:
let message: string;
message = "Hello, TypeScript!";
In the above code snippet, we declare a variable “message” of type
“string” and assign IT the value “Hello, TypeScript!”.
3. Type Inference
TypeScript can infer types based on the assigned values. This means you
don’t always have to explicitly mention the types. For instance, in the
previous example, TypeScript inferred the type of the “message”
variable as “string” because of the assigned value.
4. Working with Functions
TypeScript allows you to define the types for function parameters and
return values. Consider the following example:
function multiply(a: number, b: number): number {
return a * b;
}
In this example, the function “multiply” takes in two parameters of type
“number” and returns their product, also of type “number”.
5. TypeScript and JavaScript Libraries
TypeScript offers great compatibility with existing JavaScript
libraries and frameworks. You can install type definitions for these
libraries using npm, enabling TypeScript to understand their APIs and
provide accurate type checking during development. This greatly
enhances the overall developer experience.
FAQs
Q: Why should I use TypeScript?
A: TypeScript brings type safety and improved tooling to JavaScript,
making IT easier to catch errors and write more maintainable code.
Additionally, IT provides better editor support and improves overall
development productivity.
Q: How does TypeScript integrate with existing JavaScript code?
A: Existing JavaScript code can be gradually converted to TypeScript by
renaming files with the .ts
extension and incrementally
adding type annotations. TypeScript understands JavaScript code and
allows for seamless integration, providing benefits like static type
checking.
Q: Can TypeScript be used for both front-end and back-end development?
A: Absolutely! TypeScript is versatile and can be used for developing
both front-end and back-end applications. IT can be used in conjunction
with popular frameworks like Angular, React, Node.js, and more.
Q: Is TypeScript backward compatible with JavaScript?
A: Yes, TypeScript is fully backward compatible with JavaScript. This
means any JavaScript code is also valid TypeScript code. You can choose
to incrementally introduce type annotations and other TypeScript
features into your existing JavaScript codebase without any issues.
Q: Can I use TypeScript in my browser directly?
A: No, TypeScript code needs to be compiled into JavaScript before IT can
run in the browser. The TypeScript compiler (tsc) transpiles your
TypeScript code into JavaScript, which can then be included directly in
your HTML files.