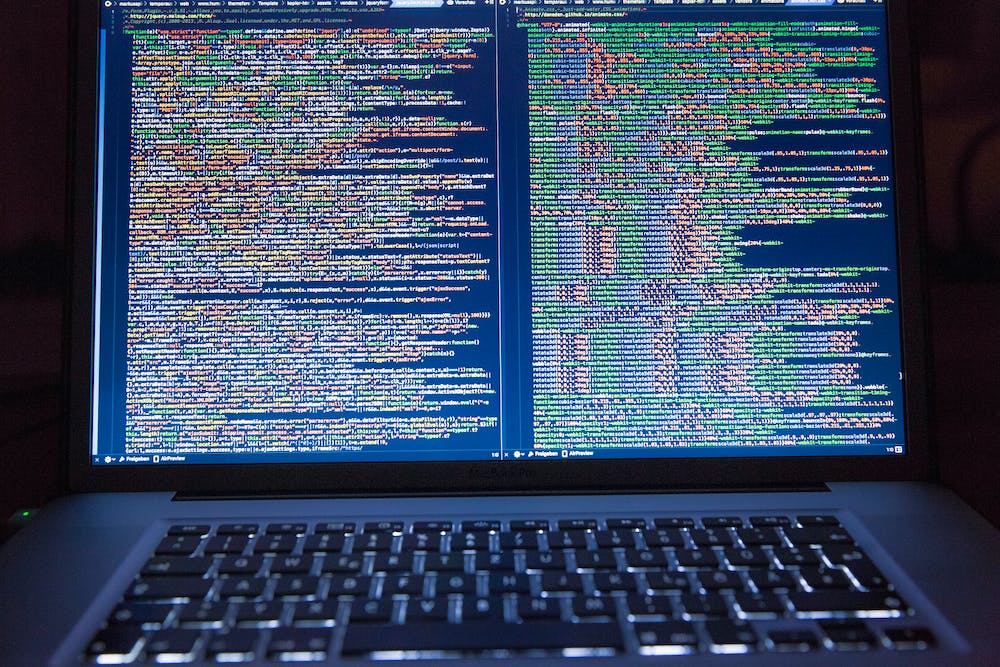
If you’re new to PHP development and looking for a powerful framework to build your web applications, Symfony PHP might be the perfect choice for you. Symfony is a robust, scalable, and high-performance framework that enables developers to create web applications with ease. In this beginner’s guide, we will walk you through the basics of getting started with Symfony PHP, including installation, project structure, routing, controllers, and more. Let’s get started!
Installation
Before we dive into developing with Symfony PHP, let’s first install the framework. Symfony can be installed via several methods, including Composer, Symfony Installer, or by downloading a ZIP archive. However, using Composer is the recommended approach.
To install Symfony PHP via Composer, open up your command line or terminal and run the following command:
composer create-project symfony/skeleton my_project_name
This command will create a new Symfony project with the given name. Once the installation is complete, navigate to the project directory using the “cd” command.
Project Structure
Symfony follows the standard directory structure that is commonly used in PHP applications. Here is a brief overview of the main directories and files you will come across while developing with Symfony PHP:
- bin/: Contains executable files used to interact with the project.
- config/: Holds all the configuration files for various aspects of the project, such as routes, services, and security.
- public/: This directory is the document root for your web server. IT contains the front controller (index.php) and any publicly accessible assets like images, CSS, and JavaScript files.
- src/: This directory is where your application’s PHP source code lives, including controllers, entities, and other classes.
- templates/: Contains the Twig templates used for rendering views.
Routing
Routing is a fundamental concept in Symfony PHP that maps incoming HTTP requests to specific actions in a controller. By convention, routes are defined in the “config/routes.yaml” file. Let’s create a simple route to get started.
Open up the “config/routes.yaml” file and add the following code:
home:
path: /
controller: App\Controller\HomeController::index
This route maps the root URL (“/”) to the “index” action in the “HomeController” class. The controller class can be found under the “src/Controller” directory. Let’s create our first controller now.
Controllers
Controllers are responsible for handling request logic and returning a response to the user. In Symfony, controllers are PHP classes that extend the base Controller class provided by the framework. Let’s create a simple controller to handle our home route.
Create a new file called “HomeController.php” under the “src/Controller” directory and add the following code:
namespace App\Controller;
use Symfony\Bundle\FrameworkBundle\Controller\AbstractController;
use Symfony\Component\HttpFoundation\Response;
class HomeController extends AbstractController
{
public function index(): Response
{
return $this->render('home/index.html.twig');
}
}
The “HomeController” class extends the base AbstractController class and contains a single method named “index”. This method uses the “render” function to render the “index.html.twig” template, which we will create shortly.
Views with Twig
Symfony PHP uses Twig as its default templating engine to separate the presentation logic from the application logic. Let’s create a simple Twig template for our home page.
Create a new file called “index.html.twig” under the “templates/home” directory and add the following code:
{% extends 'base.html.twig' %}
{% block body %}
Welcome to my Symfony PHP app!
{% endblock %}
In this template, we are extending the “base.html.twig” template provided by Symfony and including a simple heading in the “body” block. We can now access our home page by navigating to the root URL in our browser.
FAQs
Q: How can I pass data from a controller to a Twig template?
A: You can pass data to a Twig template by passing an associative array as the second argument to the “render” function in the controller. The keys in the array will be used as variable names in the template, and their values can be accessed within the template. For example: return $this->render('template.html.twig', ['name' => 'John']);
Q: How do I use database connections in Symfony PHP?
A: Symfony provides a powerful database abstraction layer called Doctrine. You can configure your database connection details in the “config/packages/doctrine.yaml” file and then use the Doctrine ORM or DBAL to interact with the database in your controllers or services.
Q: Is Symfony PHP suitable for small projects?
A: While Symfony is often associated with enterprise-level projects, IT can be used for projects of any size. IT offers flexibility and scalability, allowing developers to start small and gradually add features as the project grows.
Q: Are there any Symfony PHP tutorials or documentation available?
A: Yes, Symfony has comprehensive documentation available on its official Website – symfony.com. Additionally, there are numerous tutorials and video courses available online that cover various aspects of Symfony development.
Now that you have a basic understanding of Symfony PHP and how to get started with IT, you can explore more advanced topics such as authentication, form handling, and caching. Symfony offers a wide range of features and components that can greatly simplify your PHP development workflow. Happy coding!