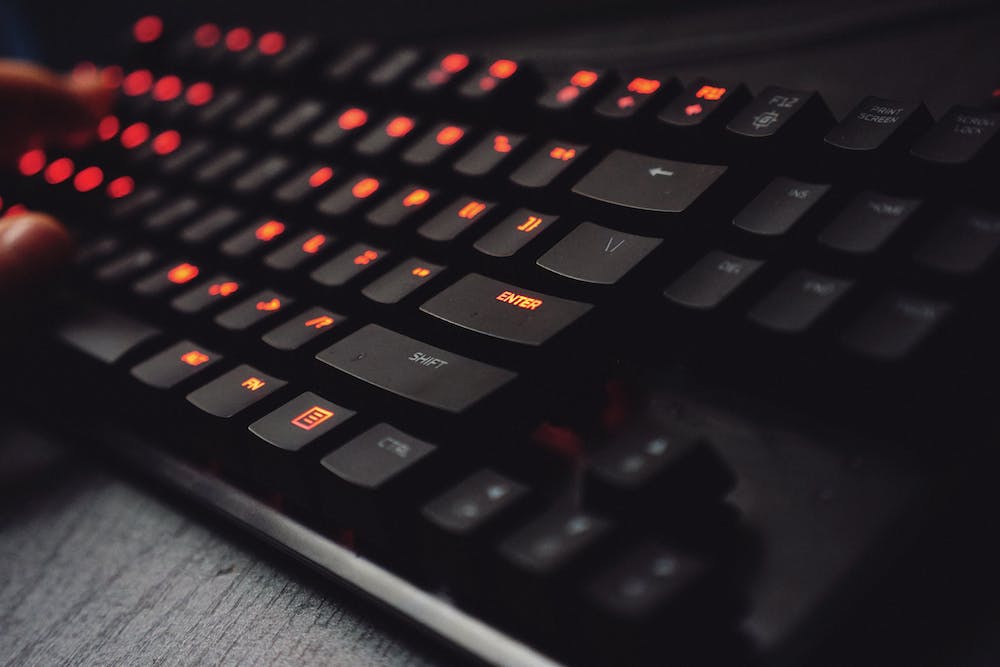
Swagger PHP is a powerful tool that allows developers to design, build, and document RESTful APIs using the OpenAPI specification. IT simplifies the development process by providing a user-friendly interface to define API endpoints, request and response payloads, and other important details. Whether you are a beginner or an experienced developer, this beginner’s guide will help you get started with Swagger PHP and start building robust APIs.
Installation
Before we dive into using Swagger PHP, we need to set up our development environment. Swagger PHP can be installed using Composer, the popular dependency management tool for PHP. Open your terminal and navigate to your project directory. If you don’t have Composer installed, you can download IT from the official Website.
$ composer require zircote/swagger-php
Configuration
Once Swagger PHP is installed, we need to configure IT to work with our project. Create a new file named swagger.php
in your project’s root directory. Open the file and add the following code:
<?php
require './vendor/autoload.php';
$options = [
'swagger' => "2.0",
'schemes' => ['http'],
'basePath' => '/api',
'host' => 'localhost',
'info' => [
'title' => 'My API',
'description' => 'API documentation using Swagger PHP',
'version' => '1.0.0',
]
];
$swagger = \Swagger\Swagger::scan('./src');
header('content-Type: application/json');
echo $swagger;
In this configuration file, we set the basic information about our API, such as the title, description, and version. We also define the basePath
and host
, which will be used in generating the correct URLs for our API endpoints. Finally, we use the \Swagger\Swagger::scan()
method to scan our project’s source code for API annotations and generate the Swagger specification.
Decorating Your API
Swagger PHP uses annotations to define API endpoints, request and response payloads, and other details. Let’s dive into some basic annotations that you can use to decorate your API controllers.
@SWG\Get
<?php
/**
* @SWG\Get(
* path="/user/{id}",
* summary="Get a user by ID",
* tags={"user"},
* @SWG\Parameter(
* name="id",
* in="path",
* description="ID of the user",
* required=true,
* type="integer"
* ),
* @SWG\Response(
* response="200",
* description="User details",
* @SWG\Schema(ref="#/definitions/User")
* ),
* @SWG\Response(
* response="404",
* description="User not found"
* )
* )
*/
In this example, we use the @SWG\Get
annotation to define a GET endpoint for retrieving a user by ID. The path
property specifies the URL path for this endpoint, with {id}
being a placeholder for the user’s ID. The summary
property provides a brief description of what the endpoint does.
We also use the @SWG\Parameter
annotation to define a path parameter named id
. This parameter is required and has a type of integer
. Additionally, we define two possible responses using the @SWG\Response
annotation. One for a successful response with status code 200 and a User
object schema, and another for a not found response with status code 404.
@SWG\Post
<?php
/**
* @SWG\Post(
* path="/user",
* summary="Create a new user",
* tags={"user"},
* @SWG\Parameter(
* name="user",
* in="body",
* required=true,
* @SWG\Schema(ref="#/definitions/User")
* ),
* @SWG\Response(
* response="201",
* description="User created successfully"
* ),
* @SWG\Response(
* response="400",
* description="Invalid request data"
* )
* )
*/
This example shows how to use the @SWG\Post
annotation to define a POST endpoint for creating a new user. The path
property specifies the URL path for this endpoint. We use the @SWG\Parameter
annotation to define a body parameter named user
. This parameter is required and contains the User
object schema.
Generating Swagger Documentation
Now that we have decorated our API with Swagger annotations, we can generate the Swagger documentation. Simply run the swagger.php
file we created earlier:
$ php swagger.php > swagger.json
This command will generate a swagger.json
file in the current directory. This file contains the Swagger specification in JSON format.
Viewing the Documentation
Swagger provides a user-friendly interface to explore and test your API. There are multiple ways to view the documentation, depending on your needs and preferences.
Swagger UI
Swagger UI is a popular tool for visualizing and interacting with API documentation. You can use IT to render the swagger.json
file we generated earlier. To do this, download the Swagger UI distribution from the official GitHub repository and copy the contents of the dist
folder to your project’s public directory.
<!-- index.html -->
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>API Documentation</title>
<link rel="stylesheet" type="text/css" href="swagger-ui.css">
<style>html { box-sizing: border-box; overflow: -moz-scrollbars-vertical; overflow-y: scroll; } *, *:before, *:after { box-sizing: inherit; } body { margin:0; background: #fafafa; }</style>
</head>
<body>
<div id="swagger-ui"></div>
<script src="swagger-ui-bundle.js"></script>
<script src="swagger-ui-standalone-preset.js"></script>
<script>
window.onload = function() {
SwaggerUIBundle({
url: "swagger.json",
dom_id: '#swagger-ui'
})
}
</script>
</body>
</html>
This code snippet shows a simple HTML file that includes the necessary CSS and JavaScript files for rendering Swagger UI. Make sure to update the paths to the CSS and JavaScript files accordingly. Open this file in your web browser to view the Swagger documentation.
FAQs
Q: Can I use Swagger PHP with any PHP framework?
A: Yes, Swagger PHP is framework-agnostic and can be used with any PHP framework or even without a framework.
Q: Is IT possible to generate client SDKs using Swagger PHP?
A: Yes, Swagger PHP provides a command-line tool called swagger-codegen
that allows you to generate client SDKs in various languages based on your Swagger specification.
Q: Can I secure my API using Swagger PHP?
A: Swagger PHP focuses on generating API documentation and does not provide built-in security features. However, you can use other libraries or techniques to secure your API endpoints.
Q: How can I test my API using Swagger PHP?
A: Swagger PHP itself does not provide testing capabilities. However, you can use tools like Postman or PHPUnit to write and execute tests against your API endpoints.
Now that you have a basic understanding of Swagger PHP, IT‘s time to unleash its power and start building your own APIs with confidence. Happy coding!