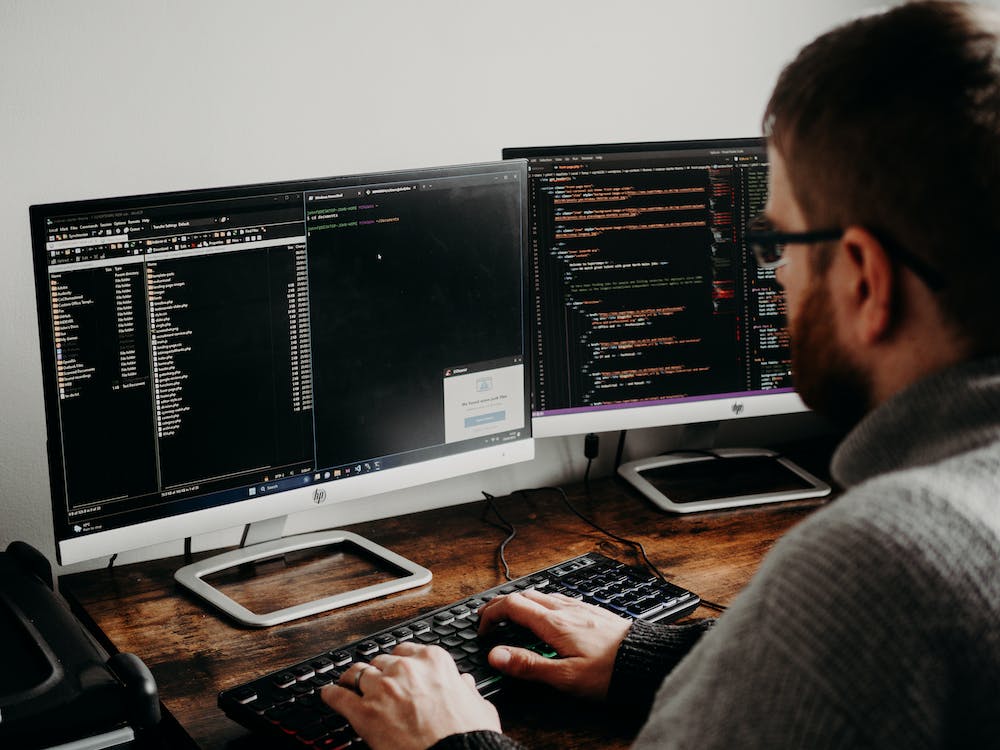
Prototype.js is a powerful JavaScript framework that simplifies the process of creating rich web applications. IT provides a wide range of features and utilities, making IT easier for developers to handle DOM manipulations, Ajax calls, animation effects, and much more. In this comprehensive guide, we will walk you through the basics of getting started with Prototype.js, including installation, syntax, and working with different components. Whether you are a beginner or an experienced developer, this guide will help you understand the fundamental concepts and techniques of using Prototype.js in your web development projects.
Installation:
To start using Prototype.js, you first need to add the library to your project. There are a few ways to do this, but the easiest way is to include IT directly from a CDN (content Delivery Network). Simply add the following script tag to the head section of your HTML file:
“`html
“`
Alternatively, you can download the latest version of Prototype.js from the official Website and include IT in your project manually.
Syntax:
Prototype.js extends the native JavaScript objects with additional methods and properties, providing a more intuitive syntax for handling common tasks. Let’s take a look at a few examples:
Selecting Elements:
Prototype.js provides a robust set of methods for selecting elements from the DOM. The most basic method is `$()`, which is similar to `document.getElementById()`. IT returns a reference to the first element with the specified ID. For example:
“`javascript
var element = $(‘myElement’);
“`
You can also use CSS selectors to select elements. Prototype.js provides a method called `$$()` that accepts CSS selectors and returns an array of matching elements. For example:
“`javascript
var elements = $$(‘.myClass’);
“`
Creating Elements:
Prototype.js allows you to create new HTML elements dynamically. The `new Element()` method takes the tag name of the element you want to create as the argument. You can then use various methods to set attributes, styles, and add content to the element. For example:
“`javascript
var newDiv = new Element(‘div’);
newDiv.addClass(‘myClass’);
newDiv.update(‘This is a new div element.’);
“`
Working with Ajax:
Prototype.js simplifies the process of making asynchronous requests using Ajax. The `new Ajax.Request()` method allows you to create a new Ajax request, specify the URL and the HTTP method (GET, POST, etc.), and handle the response. For example:
“`javascript
new Ajax.Request(‘/api/data’, {
method: ‘get’,
onSuccess: function(response) {
console.log(response.responseText);
},
onFailure: function(response) {
console.error(‘Request failed!’);
}
});
“`
Working with Effects:
Prototype.js provides a set of built-in animation effects that you can apply to elements on your page. The `Element#morph()` method allows you to smoothly transition between CSS styles. For example:
“`javascript
var myElement = $(‘myElement’);
myElement.morph(‘background-color: #ff0000; font-size: 20px;’, {
duration: 0.5,
delay: 1
});
“`
FAQs:
Q: What are the advantages of using Prototype.js?
A: Prototype.js simplifies JavaScript development by providing an intuitive syntax and a wide range of features. IT makes IT easier to handle DOM manipulations, Ajax calls, animations, and more.
Q: Is Prototype.js compatible with other JavaScript frameworks?
A: Prototype.js can coexist with other frameworks, but there may be conflicts if they both extend native JavaScript objects. IT is recommended to avoid using multiple frameworks on the same page.
Q: Can I use Prototype.js with modern JavaScript modules?
A: Prototype.js is not designed to work as a module. If you are using a module bundler like Webpack or Rollup, you can import individual functions or components from Prototype.js instead of including the entire library.
Q: Is Prototype.js actively maintained?
A: Prototype.js is no longer actively maintained or updated. The last official release (version 1.7.3) was in 2013. IT is recommended to consider using alternative frameworks like jQuery, React, or Vue.js for modern web development.
In conclusion, Prototype.js is a comprehensive JavaScript framework that provides a convenient and powerful set of tools for web development. By understanding the basics of installation, syntax, and working with different components, you can leverage the capabilities of Prototype.js to build dynamic and interactive web applications.