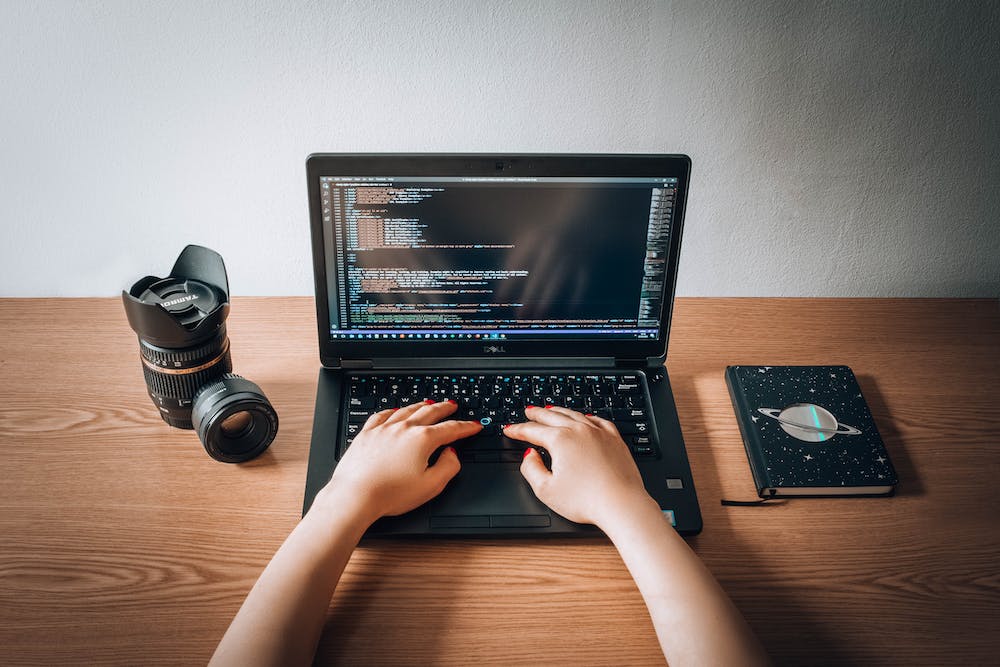
Redis is an open-source, in-memory data structure store that is used as a database, cache, and message broker. IT supports various data structures such as strings, hashes, lists, sets, and more. In this guide, we will walk you through the process of setting up and configuring PHP Redis.
Setting Up Redis Server
The first step in using PHP Redis is to set up a Redis server. You can install Redis on your server using package managers such as apt or yum. Once the installation is complete, you can start the Redis server by running the following command:
redis-server
This will start the Redis server on the default port 6379. You can also specify a configuration file using the --config
option.
Installing PHP Redis Extension
Once the Redis server is set up, you need to install the PHP Redis extension to interact with the server from your PHP code. You can install the extension using the pecl command.
pecl install redis
After the installation is complete, you can add the following line to your php.ini file to enable the extension:
extension = redis.so
Connecting to Redis Server
Now that you have installed the PHP Redis extension, you can start interacting with the Redis server from your PHP code. The first step is to establish a connection to the server using the Redis
class:
$redis = new Redis();
$redis->connect('127.0.0.1', 6379);
In the above code, we are creating a new instance of the Redis
class and connecting to the Redis server running on localhost on the default port 6379. You can also specify a different host and port if your Redis server is running on a different machine or port.
Storing and Retrieving Data
Once you have established a connection to the Redis server, you can start storing and retrieving data using various data structures supported by Redis. Here are a few examples:
Strings
To store a string value in Redis, you can use the set
method:
$redis->set('name', 'John Doe');
To retrieve the value, you can use the get
method:
$name = $redis->get('name');
echo $name; // Output: John Doe
Lists
You can store a list of values in Redis using the lpush
method:
$redis->lpush('logs', 'log1');
$redis->lpush('logs', 'log2');
To retrieve the values, you can use the lrange
method:
$logs = $redis->lrange('logs', 0, -1);
print_r($logs); // Output: Array ( [0] => log2 [1] => log1 )
Conclusion
In this guide, we have covered the basic steps to set up and configure PHP Redis. We have also demonstrated how to establish a connection to the Redis server, store and retrieve data using various data structures supported by Redis. With this knowledge, you can start incorporating Redis into your PHP applications to take advantage of its high-performance data storage and caching capabilities.
FAQs
What is Redis?
Redis is an open-source, in-memory data structure store that is used as a database, cache, and message broker.
How do I install Redis server?
You can install Redis server using package managers such as apt or yum. Once the installation is complete, you can start the Redis server by running the redis-server
command.
How do I install PHP Redis extension?
You can install the PHP Redis extension using the pecl install redis
command. After the installation is complete, you can enable the extension by adding a line to your php.ini file.
How do I connect to Redis server from PHP?
You can establish a connection to the Redis server using the Redis
class and the connect
method.
What data structures are supported by Redis?
Redis supports various data structures such as strings, hashes, lists, sets, and more.
Can I use Redis for caching?
Yes, Redis is commonly used as a caching solution due to its high-performance in-memory storage.