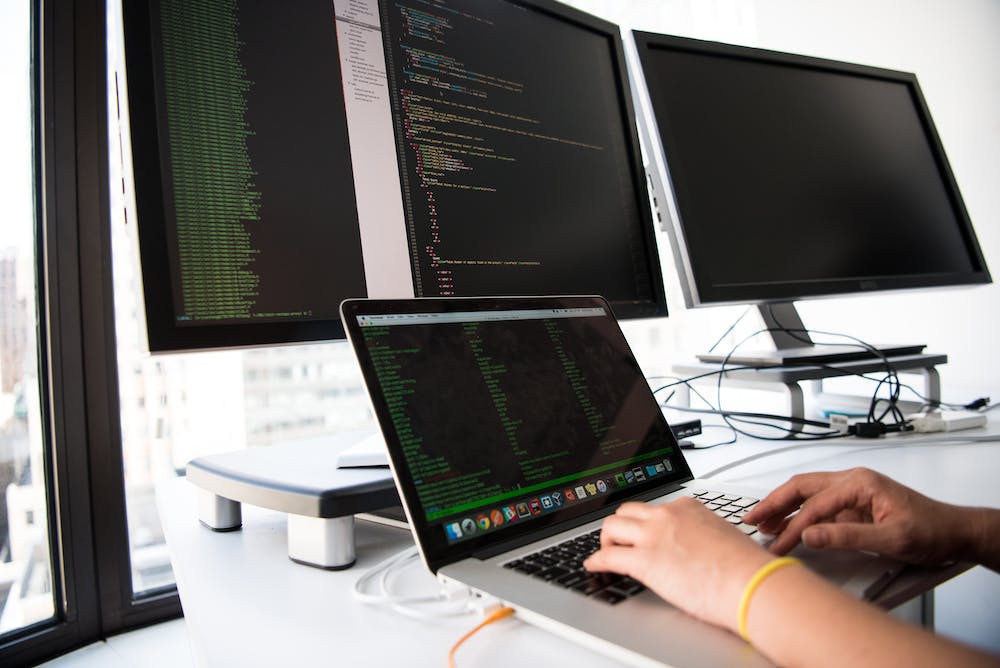
PHP and MySQL are two powerful tools used in web development. PHP is a server-side scripting language, while MySQL is a relational database management system. Together, they provide developers with the ability to create dynamic websites and applications. If you are new to PHP and MySQL, this beginner’s guide will help you get started with the basics and understand how they work together.
Setting up the Environment
Before you can start working with PHP and MySQL, you need to set up your development environment. You will need a web server with PHP support, such as Apache or Nginx, and a MySQL server. You can install them individually, or use pre-configured packages like XAMPP or WAMP that include both. Once you have your environment set up, you’re ready to start coding.
writing PHP Code
PHP code is usually embedded in HTML files, helping to create dynamic content. To start writing PHP code, simply open your text editor and create a new file with a .php extension. You can then use the opening and closing PHP tags <?php and ?> to delimit your PHP code. Any code within these tags will be executed on the server before the HTML is sent to the client’s browser.
Here’s a basic example that demonstrates how PHP works:
<?php
$name = "John Doe";
echo "Hello, " . $name . "!";
?>
In the above example, we declare a variable named ‘name’ and assign IT the value “John Doe.” Then, we use the echo statement to output a greeting to the user using the value of the ‘name’ variable. The output will be “Hello, John Doe!” You can run this PHP code on your web server, and the result will be displayed in your web browser.
Connecting to MySQL
MySQL is used to manage databases in your web applications. To connect to a MySQL database from PHP, you need to establish a connection using the mysqli or PDO extension, both of which come bundled with PHP. Here’s an example of connecting to a MySQL database using the mysqli extension:
<?php
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$database = "your_database";
$conn = new mysqli($servername, $username, $password, $database);
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
echo "Connected successfully";
?>
In the above example, you need to replace ‘your_username’, ‘your_password’, and ‘your_database’ with your MySQL credentials. If the connection is established successfully, the output will be “Connected successfully.”
Executing SQL Queries
Once you have established a connection to the MySQL database, you can execute SQL queries using PHP. For example, if you want to retrieve data from a table named ‘users’, you can use the following code:
<?php
$sql = "SELECT * FROM users";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
while ($row = $result->fetch_assoc()) {
echo "Name: " . $row["name"]. " - Email: " . $row["email"]. "<br>";
}
} else {
echo "0 results";
}
?>
In the above example, we first define an SQL query to select all rows from the ‘users’ table. Then, we use the query() method to execute the query and store the result in the $result variable. If there are rows returned, we loop through each row using fetch_assoc() and print the name and email fields. Otherwise, if there are no results, we print “0 results.”
FAQs
Q: Is PHP a beginner-friendly language?
A: Yes, PHP is considered to be relatively easy to learn for beginners due to its simple syntax and extensive documentation.
Q: Do I need to be an expert in SQL to work with MySQL?
A: While having some SQL knowledge is beneficial, basic SQL operations can be easily learned and performed using PHP.
Q: Can I use PHP with other databases besides MySQL?
A: Yes, PHP supports a variety of databases, including PostgreSQL, SQLite, Oracle, and more.
Q: Is IT necessary to use a web server for PHP development?
A: Yes, PHP is a server-side scripting language, so IT needs a web server to interpret and execute the PHP code.
Q: Are there any popular PHP frameworks that I should know about?
A: Yes, some popular PHP frameworks include Laravel, Symfony, and CodeIgniter. These frameworks provide additional functionalities and make PHP development more efficient.
This beginner’s guide has covered the basics of getting started with PHP and MySQL. From setting up the environment to executing SQL queries, you should now have a good foundation to explore and build dynamic web applications. Don’t forget to leverage the vast online resources and communities available to further enhance your learning and development journey.