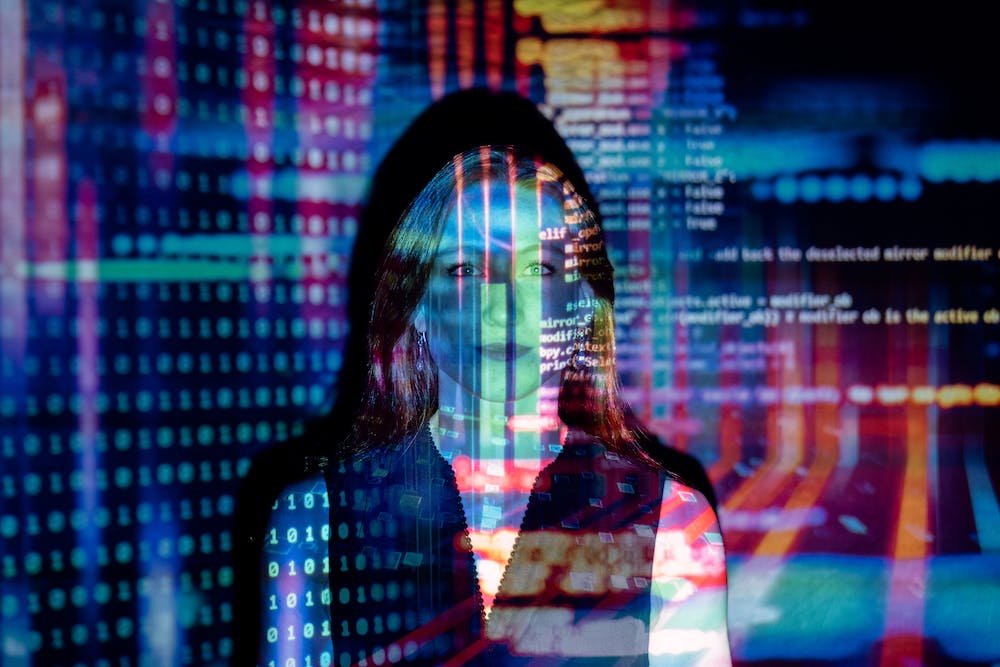
Welcome to this beginner’s guide on getting started with Node.js. If you’re new to Node.js or are still exploring its possibilities, then you’ve come to the right place. In this article, we will cover the basics of Node.js, from installation to setting up your first server, and dive into some commonly asked questions along the way. By the end, you’ll have a solid foundation to start building powerful and scalable applications using Node.js.
What is Node.js?
Node.js is an open-source, cross-platform JavaScript runtime environment built on Chrome’s V8 JavaScript engine. IT allows developers to execute JavaScript outside the browser, on the server-side, and build scalable network applications with ease. Node.js uses an event-driven, non-blocking I/O model, making IT lightweight and efficient for handling concurrent requests.
Installing Node.js
To get started with Node.js, you’ll need to install IT on your machine. Head over to the official Node.js Website and download the installer for your operating system. Once downloaded, run the installer and follow the installation wizard to complete the setup. After successful installation, you can verify if Node.js is properly installed by opening your terminal or Command Prompt and typing the command node -v
. If IT displays the version number, then Node.js is installed correctly.
Setting Up Your First Node.js Server
Now that Node.js is installed, let’s create our first server. Open a new file with a .js extension, for example, server.js
, and let’s start by requiring the ‘http’ module:
const http = require('http');
Next, we can create a new server using the http.createServer()
method and define how IT handles incoming requests. Here’s an example of a basic server that sends a ‘Hello, World!’ response to every request:
const server = http.createServer((req, res) => {
res.writeHead(200, {'content-Type': 'text/plain'});
res.end('Hello, World!');
});
server.listen(3000, 'localhost', () => {
console.log('Server running at http://localhost:3000/');
});
To start the server, save the file and execute IT using Node.js. If everything goes well, you should see a message in the console indicating that the server is running. You can access the server by opening your browser and visiting http://localhost:3000/. You should see the ‘Hello, World!’ message displayed.
Frequently Asked Questions (FAQs)
1. What is the difference between Node.js and JavaScript?
Node.js is not a programming language itself; IT is a runtime environment for executing JavaScript code on the server-side. JavaScript, on the other hand, is a programming language that can run both on the client-side (in web browsers) and the server-side (with Node.js).
2. Can I use Node.js with other programming languages?
Yes, Node.js can interact with other programming languages through its built-in C/C++ bindings or by making use of various libraries and modules available in the Node.js ecosystem.
3. Can Node.js handle concurrent requests?
Yes, Node.js excels at handling concurrent requests due to its event-driven, non-blocking I/O model. IT can efficiently handle multiple requests without blocking other incoming requests.
4. Is Node.js suitable for building real-time applications?
Absolutely! Node.js is well-suited for building real-time applications such as chat apps, collaborative tools, or even multiplayer games. Its event-driven architecture allows handling real-time data streams with ease.
Now that you have a solid understanding of Node.js basics and have answered some frequently asked questions, you’re ready to explore and build exciting applications using this powerful runtime environment. Happy coding!