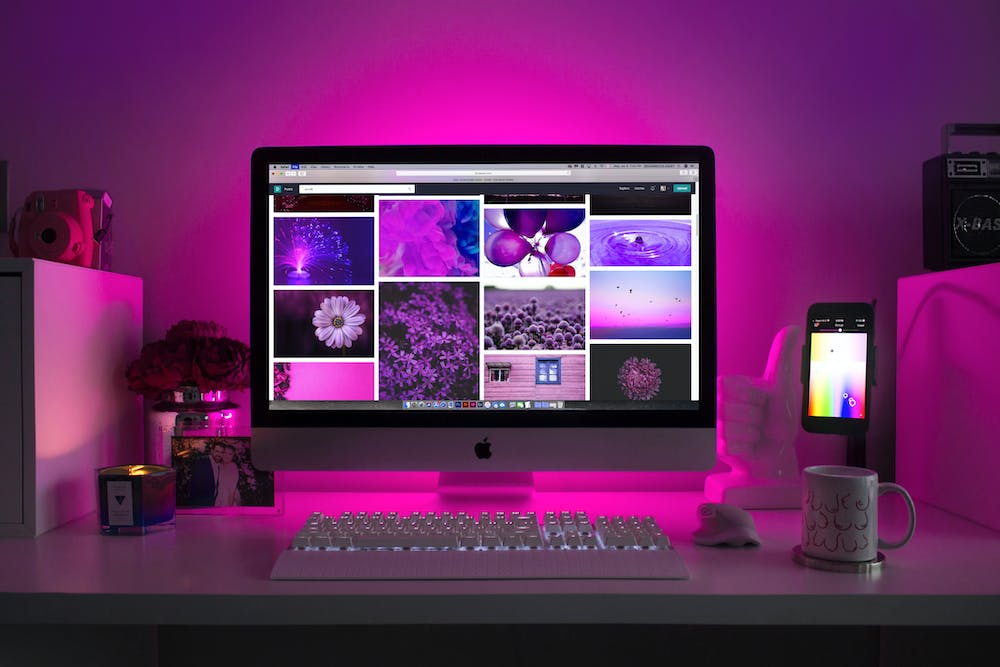
Laravel and Vue.js are two popular tools used for web development. Laravel is a PHP framework that provides an elegant syntax and a robust set of tools to build web applications, while Vue.js is a JavaScript framework that focuses on building user interfaces. When combined, Laravel and Vue.js create a powerful duo for web development, allowing developers to build dynamic and interactive web applications with ease.
Getting started with Laravel and Vue.js is fairly straightforward. Before diving into the details, make sure you have a basic understanding of PHP and JavaScript. Once you have that, follow the steps below to get started:
Step 1: Install Laravel
The first step is to install Laravel on your local machine. Laravel provides a convenient installer that can be accessed via Composer, a dependency manager for PHP. Open your terminal and run the following command:
composer global require laravel/installer
This will install the Laravel installer globally on your system. Once the installation is complete, you can create a new Laravel project by running the following command:
laravel new project-name
Replace “project-name” with the desired name of your project. This command will create a new Laravel project in a directory with the specified name.
Step 2: Set Up the Database
Next, navigate to the project directory and open the “.env” file. This file contains the configuration settings for your Laravel project. Locate the following lines:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=your-database
DB_USERNAME=root
DB_PASSWORD=
Modify the “DB_DATABASE”, “DB_USERNAME”, and “DB_PASSWORD” fields to match your local database settings. Save the changes and close the file.
Step 3: Create Routes and Controllers
Laravel follows the Model-View-Controller (MVC) architectural pattern. To create routes and controllers, open the “routes/web.php” file. Here, you can define routes and their associated controller methods. For example, to create a route that maps to a controller method named “index”, add the following code:
Route::get('/', 'ControllerName@index');
Replace “ControllerName” with the actual name of your controller class.
Step 4: Install Vue.js
To install Vue.js, navigate to your project directory and run the following command:
npm install
This command will install all the necessary dependencies defined in the “package.json” file, including Vue.js.
Step 5: Create Vue Components
In Vue.js, components are reusable elements that encapsulate HTML, CSS, and JavaScript. To create a Vue component, create a new “.vue” file in the “resources/js/components” directory. For example, create a file named “ExampleComponent.vue” and add the following code:
<template>
<div>
<h1>Hello World!</h1>
</div>
</template>
<script>
export default {
name: 'ExampleComponent'
}
</script>
In the above code, we define a template with a simple heading. We also specify the name of the component using the “name” property. This component can now be used within your Laravel application.
Step 6: Include Vue Component in Blade Template
To include the Vue component in your Laravel blade templates, open a blade template file, such as “resources/views/welcome.blade.php”, and add the following code within the body of the HTML document:
<example-component></example-component>
Replace “example-component” with the appropriate name of your Vue component.
Step 7: Compile Assets
Finally, compile the assets by running the following command in your terminal:
npm run dev
This command will compile the JavaScript and CSS files into a format that can be understood by the browser.
Once you have followed these steps, you will have a basic setup of Laravel and Vue.js. You can now start building your web application by creating more routes, controllers, and Vue components.
FAQs:
1. Can I use other JavaScript frameworks with Laravel?
Yes, Laravel is flexible and allows you to use other JavaScript frameworks like React or Angular alongside Vue.js. You can choose the framework that best suits your project requirements.
2. Do I need to be proficient in PHP to use Laravel?
While IT is beneficial to have a basic understanding of PHP, Laravel provides a clean and expressive syntax that makes development easier even for beginners.
3. Can I use Laravel and Vue.js for mobile app development?
No, Laravel and Vue.js are primarily designed for web development. If you want to build a mobile app, you should consider using frameworks like React Native or Flutter.
4. Are there any alternatives to Laravel for PHP web development?
Yes, there are other PHP frameworks like Symfony, CodeIgniter, and Yii that you can choose from. Each framework has its own set of features and advantages, so IT‘s worth exploring different options to find the one that suits your needs.
In conclusion, Laravel and Vue.js provide a powerful duo for web development. By following the steps outlined above, you can quickly get started with building dynamic and interactive web applications. Don’t hesitate to explore the vast Laravel and Vue.js ecosystems to take your development skills to the next level!