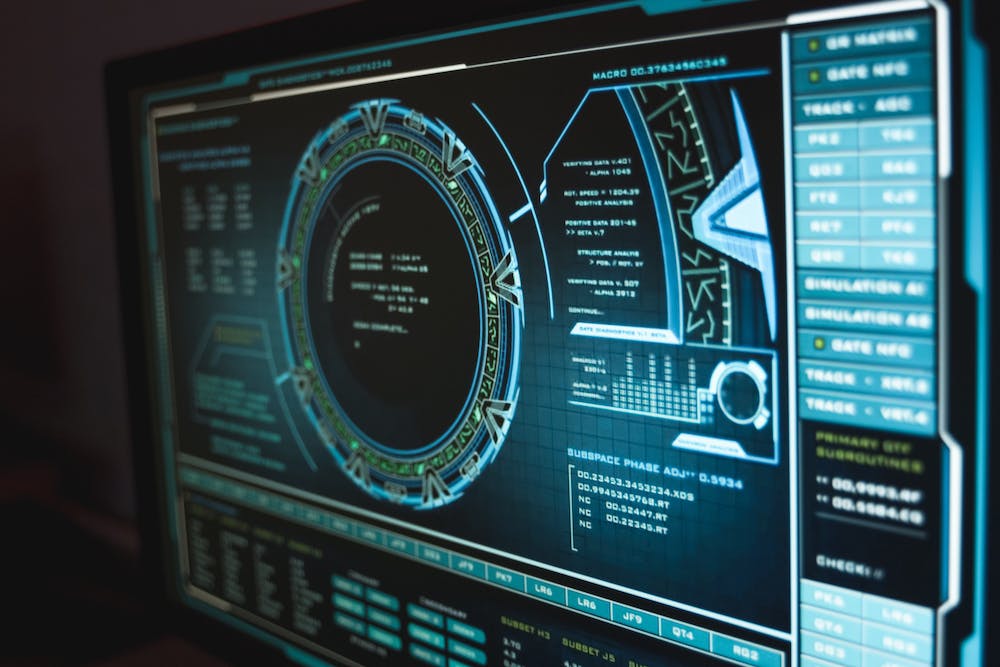
Introduction
Laravel has gained immense popularity among developers due to its elegant syntax, robust features, and comprehensive documentation. If you’re new to Laravel, this beginner’s guide will walk you through the key concepts and provide a step-by-step approach to get started with Laravel development.
What is Laravel?
Laravel is an open-source PHP framework that follows the Model-View-Controller (MVC) architectural pattern. IT provides a clean and expressive syntax, making IT easier for developers to build web applications rapidly. Laravel aims to make development pleasant without sacrificing application functionality or performance.
Installation and Configuration
The first step to getting started with Laravel is installing IT. Laravel utilizes Composer, a PHP dependency management tool, to handle framework installation and dependency management. Let’s go through the installation process:
- Ensure you have PHP and Composer installed on your machine.
- Open your terminal and navigate to the desired directory where you want to create your Laravel project.
- Run the following command to create a new Laravel project:
composer create-project --prefer-dist laravel/laravel <project-name>
Replace <project-name> with the desired name for your project.
Once the project is created, navigate into the project folder using the terminal:
cd <project-name>
Next, you can use the built-in PHP development server or serve your Laravel application with a local development environment such as XAMPP, WAMP, or Laravel Valet.
To serve your Laravel application using the built-in PHP development server, run the following command in your terminal:
php artisan serve
This command will start the development server, enabling you to access your Laravel application via http://localhost:8000
in your web browser.
Configuration and Environment Setup
Laravel comes with a configuration file that enables you to customize various aspects of your application. The configuration file can be found in the config
directory of your project.
Additionally, Laravel uses an environment file to manage your application’s environment-specific settings. The environment file is named .env
and is located in the root directory of your Laravel project. This file holds sensitive information such as database credentials, mail configurations, and application-specific variables.
Creating Routes and Views
Routes play a vital role in Laravel applications as they allow you to map URLs to corresponding controller actions. Laravel provides a clean and expressive way to define routes using the routes/web.php
file.
To define a route, open the routes/web.php
file and add the following code:
Route::get('/hello', function () {
return view('hello');
});
The code snippet above defines a GET route for the /hello
URL, which returns the hello
view. Views in Laravel are customizable templates that allow you to present your application’s data to the user.
To create a new view, navigate to the resources/views
directory of your project and create a new file named hello.blade.php
. Add the desired HTML and content to this file. For example:
<html>
<head>
<title>Hello World</title>
</head>
<body>
<h1>Hello, World!</h1>
<p>Welcome to my Laravel application.</p>
</body>
</html>
Now, when you access http://localhost:8000/hello
in your web browser, you will see the “Hello, World!” message displayed.
Working with Databases
Laravel provides an intuitive ActiveRecord implementation called Eloquent, making database interactions efficient and straightforward.
To create a new model and migration, use the following Artisan command:
php artisan make:model <ModelName> -m
Replace <ModelName> with the desired name for your model. This command will generate a new model file and a corresponding migration file in the database/migrations
directory.
In the migration file, you can define the structure of your database table using the provided schema builder. After defining the table structure, run the following command to migrate the changes to the database:
php artisan migrate
Laravel also provides a convenient way to interact with the database through the Artisan command-line interface. You can enter the interactive database shell using the following command:
php artisan tinker
Once inside the Tinker shell, you can use Eloquent’s expressive syntax to perform various database operations.
Conclusion
Congratulations! You’ve successfully covered the basics of getting started with Laravel. From installation and configuration to route creation, view rendering, and working with databases, you now have the foundational knowledge to build web applications using Laravel. As you continue your Laravel journey, don’t forget to explore the comprehensive Laravel documentation and join the vibrant Laravel community for further assistance and support.
Frequently Asked Questions
1. What is Laravel?
Laravel is an open-source PHP framework that follows the Model-View-Controller (MVC) architectural pattern. IT provides a clean and expressive syntax for rapid web application development.
2. How do I install Laravel?
To install Laravel, make sure you have PHP and Composer installed on your machine. Then, run the command composer create-project --prefer-dist laravel/laravel <project-name>
in your terminal.
3. How do I create routes in Laravel?
To create routes in Laravel, open the routes/web.php
file and define your routes using the provided syntax. Routes map URLs to corresponding controller actions.
4. How do I work with databases in Laravel?
Laravel provides an intuitive database abstraction layer called Eloquent. You can define models and migrations to interact with the database using ActiveRecord-style syntax. Laravel’s Artisan CLI also provides a convenient way to perform database operations.