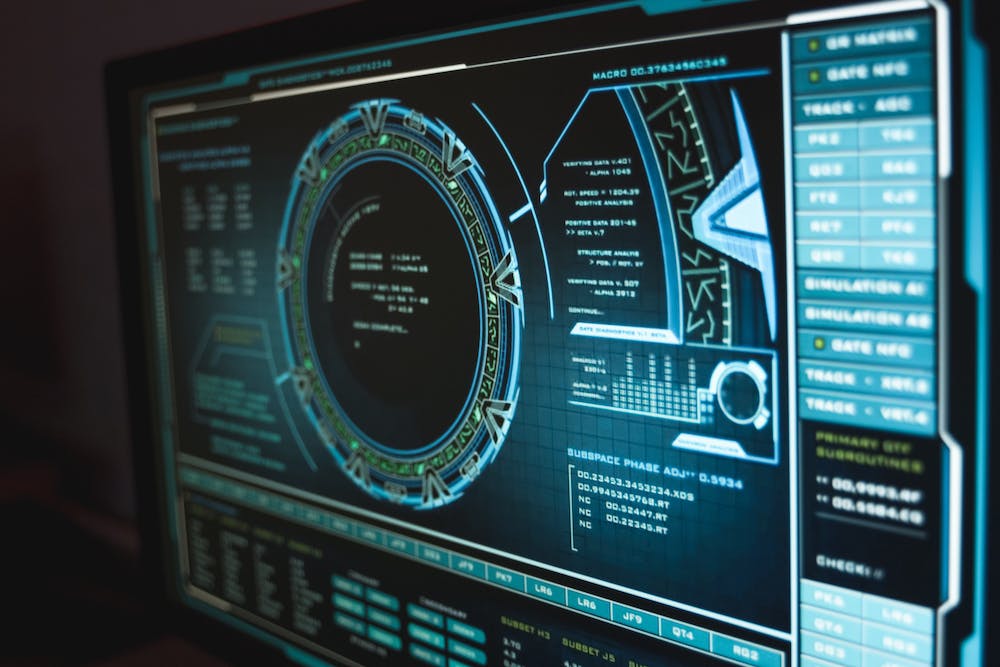
Kafka is a distributed event streaming platform that is widely used for building real-time data pipelines and streaming applications. KafkaJS is a modern and feature-rich JavaScript client for Apache Kafka, which enables you to effortlessly build Kafka consumers and producers using Node.js.
In this article, we will guide you through the process of setting up a Kafka consumer and producer using KafkaJS in a Node.js environment. By the end of this tutorial, you will have a strong foundational understanding of how KafkaJS works and how to use IT to build robust Kafka applications.
Prerequisites
Before we begin, make sure you have the following prerequisites installed:
- Node.js and npm: You can download and install Node.js from the official Website.
- Apache Kafka: You will need a running Kafka cluster to connect to. You can follow the official documentation to set up Kafka locally or use a cloud-based Kafka service.
Setting up KafkaJS
First, we need to install the KafkaJS package using npm. Open your terminal and run the following command:
npm install kafka-node
Once the installation is complete, you can start creating your Kafka consumer and producer.
Creating a Kafka Consumer
To create a Kafka consumer using KafkaJS, you will need to define a Kafka client and subscribe to a topic. Let’s go through the steps:
- Import the required KafkaJS modules:
- Create a Kafka client:
- Create a consumer:
- Subscribe to the topic:
const { Kafka } = require('kafka-node');
const client = new Kafka.Client("kafka-broker-url");
const consumer = new Kafka.SimpleConsumer(client, [{ topic: 'your-topic' }]);
consumer.on('message', function (message) {
console.log(message);
});
With these steps, you have successfully created a Kafka consumer using KafkaJS. The consumer will listen for incoming messages from the specified topic and log them to the console.
Creating a Kafka Producer
Creating a Kafka producer using KafkaJS is similarly straightforward. Let’s walk through the process:
- Import the required KafkaJS modules:
- Create a Kafka client:
- Create a producer:
- Send a message to a topic:
const { Kafka } = require('kafka-node');
const client = new Kafka.Client("kafka-broker-url");
const producer = new Kafka.Producer(client);
const payload = [{ topic: 'your-topic', messages: 'your-message' }];
producer.send(payload, function (error, data) {
console.log(data);
});
By following these steps, you have successfully created a Kafka producer using KafkaJS. The producer will send messages to the specified topic, and you will receive a confirmation once the message is sent.
Conclusion
Congratulations! You have learned how to set up a Kafka consumer and producer using KafkaJS in a Node.js environment. KafkaJS offers a simple and powerful way to interact with Apache Kafka, making it suitable for building robust and efficient event streaming applications.
As you continue to work with KafkaJS, you will discover its vast range of features and customization options that allow you to tailor your Kafka applications to your specific requirements. Whether you are building real-time data pipelines, event-driven microservices, or stream processing applications, KafkaJS provides a solid foundation for handling Kafka integration in Node.js.
FAQs
Q: Can I use KafkaJS with other programming languages?
A: KafkaJS is specifically designed for Node.js, so it is not directly compatible with other programming languages. However, Kafka itself is language-agnostic, and you can use the official Kafka clients for other programming languages to achieve similar functionality.
Q: What are the advantages of using KafkaJS over other Kafka clients?
A: KafkaJS offers a modern and idiomatic JavaScript interface for working with Kafka, leveraging async/await and other modern language features. It also provides strong support for TypeScript, making it a natural choice for Node.js applications built with TypeScript.
Moreover, KafkaJS is actively maintained and has a thriving community, ensuring that you have access to ongoing support and updates. If you are already working with Node.js, using KafkaJS can streamline your development process and provide a seamless integration with Kafka.
Q: How can I handle error handling in KafkaJS?
A: KafkaJS provides robust error handling mechanisms, allowing you to catch and handle various error scenarios that may occur during Kafka communication. You can use native JavaScript error handling techniques, such as try/catch blocks and error event listeners, to manage errors and ensure the reliability of your Kafka applications.
Q: Is KafkaJS suitable for production use?
A: Yes, KafkaJS is suitable for production use and is used by many organizations for building scalable and reliable Kafka applications. It is important to follow best practices for Kafka deployment and configuration, as well as ensuring the proper monitoring and maintenance of your Kafka clusters. Additionally, staying updated with KafkaJS releases and applying security patches will help ensure a secure and stable production environment.
Q: How can I optimize the performance of KafkaJS consumers and producers?
A: To optimize the performance of KafkaJS consumers and producers, you can fine-tune various configuration parameters, such as batch sizes, linger times, and compression settings. Additionally, utilizing multi-partition consumption and parallel message processing can help distribute the workload and improve throughput. Monitoring and profiling your Kafka applications can also help identify potential bottlenecks and areas for optimization.
Q: Are there any known limitations or trade-offs when using KafkaJS?
A: While KafkaJS is a powerful and feature-rich client for Kafka, it is important to be aware of its limitations and trade-offs. For example, KafkaJS may have slightly higher overhead compared to native Kafka clients, as it operates within the Node.js runtime environment. Additionally, certain advanced Kafka features or performance optimizations may require more intricate setup and configuration. It is essential to carefully evaluate your specific use case and consider the trade-offs when choosing KafkaJS for your Kafka integration.