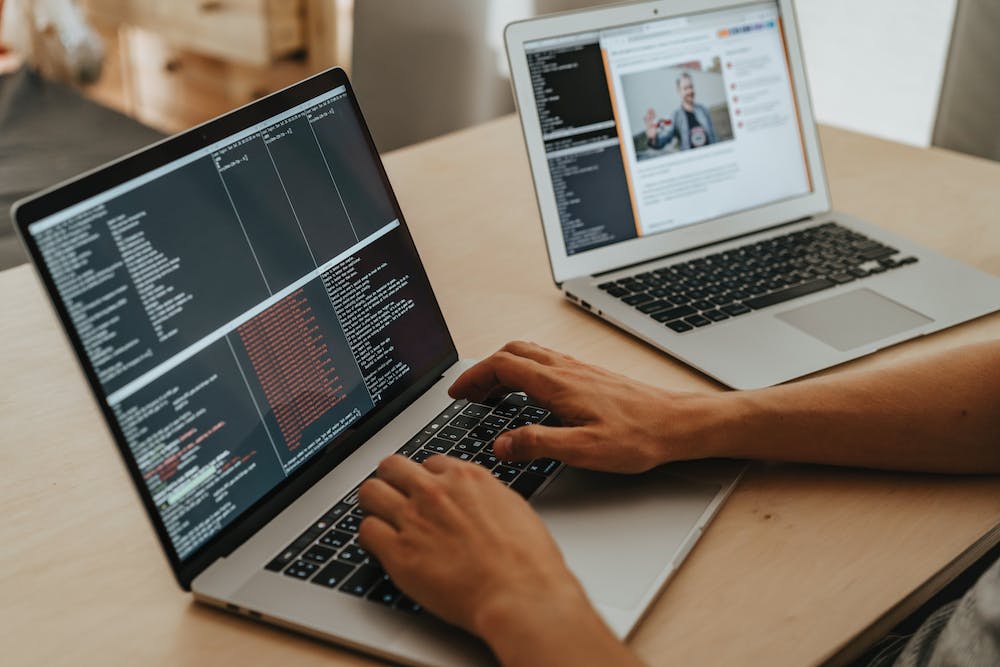
Facebook PHP is a powerful tool that allows developers to integrate Facebook’s features into their applications. Whether you are building a Website or a mobile app, using Facebook PHP can provide numerous benefits such as connecting with users, retrieving data, and sharing content. In this beginner’s guide, we will walk you through the necessary steps to get started with Facebook PHP.
Step 1: Set Up a Facebook Developer Account
The first step in getting started with Facebook PHP is to create a Facebook Developer account. You can do this by visiting the Facebook Developers Website and signing up. Once you have successfully signed up, you will have access to the Facebook Developer dashboard, where you can create and manage your Facebook apps.
Step 2: Create a New Facebook App
After setting up your developer account, IT‘s time to create a new Facebook app. By creating an app, you will obtain an App ID and App Secret, which are required for integrating Facebook PHP into your application. In the Facebook Developer dashboard, click on the “My Apps” section and then the “Create App” button. Provide a name for your app and select the desired category (e.g., Website or mobile app).
Step 3: Set Up Facebook PHP SDK
To start using Facebook PHP, you need to download and set up the official Facebook PHP SDK (software Development Kit). The SDK provides a collection of libraries and tools that simplify the integration process. You can find the latest version of the SDK on GitHub. Download the SDK package and extract IT to a directory in your project.
Step 4: Configure Your Facebook App
Before you can integrate your app with Facebook PHP, you need to configure your Facebook app settings. In the Facebook Developer dashboard, navigate to your app’s settings and provide the necessary details, including the App Domains and Site URL. These settings ensure that Facebook can authenticate and authorize your app.
Step 5: Include Facebook PHP SDK in Your Project
To start using Facebook PHP in your application, you need to include the Facebook PHP SDK files. This can be done by adding the following line of code at the top of your PHP script:
“`php
require_once ‘path/to/facebook-php-sdk/autoload.php’;
“`
Make sure to replace ‘path/to/facebook-php-sdk’ with the actual path where you extracted the SDK files in Step 3.
Step 6: Initialize Facebook PHP SDK
After including the SDK, you need to initialize IT with your App ID and App Secret. Add the following code below the previous include statement:
“`php
$fb = new \Facebook\Facebook([
‘app_id’ => ‘your-app-id’,
‘app_secret’ => ‘your-app-secret’,
‘default_graph_version’ => ‘v11.0’,
]);
“`
Replace ‘your-app-id’ and ‘your-app-secret’ with your actual App ID and App Secret obtained in Step 2. Also, note the ‘default_graph_version’ setting, which specifies the version of the Facebook Graph API to use.
Step 7: Make Your First Facebook API Call
Now that you have set up the SDK, you can start making API calls to retrieve data from Facebook. For example, to fetch the basic information of the currently logged-in user, you can use the following code:
“`php
try {
$response = $fb->get(‘/me’);
$user = $response->getGraphUser();
echo ‘Hello, ‘ . $user->getName();
} catch(\Facebook\Exceptions\FacebookResponseException $e) {
echo ‘Graph returned an error: ‘ . $e->getMessage();
} catch(\Facebook\Exceptions\FacebookSDKException $e) {
echo ‘Facebook SDK returned an error: ‘ . $e->getMessage();
}
“`
This code sends a GET request to the ‘/me’ endpoint of the Facebook Graph API and retrieves the user’s basic information. If the request is successful, IT then prints a greeting message with the user’s name. If an error occurs, the appropriate exception will be caught and an error message will be displayed.
FAQs (Frequently Asked Questions)
Q: Do I need to have a Facebook account to use Facebook PHP?
No, you don’t need a personal Facebook account. However, you do need to create a Facebook Developer account to access the necessary tools and resources.
Q: Can I use Facebook PHP with any programming language?
Yes, Facebook PHP can be used with any programming language that supports making HTTP requests and handling JSON responses. However, the official Facebook PHP SDK is specifically designed for PHP applications.
Q: Are there any restrictions on using Facebook PHP in my application?
Yes, there are certain restrictions and guidelines provided by Facebook that you need to follow when using Facebook PHP. These include respecting user privacy, obtaining necessary permissions, and complying with Facebook’s terms and policies.
Q: Can I customize the data I retrieve from Facebook?
Yes, the Facebook Graph API allows you to specify the fields and parameters when making API calls. This enables you to retrieve only the required data and customize the response according to your application’s needs.
Q: Are there any rate limits or quotas for using Facebook PHP?
Yes, Facebook imposes certain rate limits and quotas to prevent abuse and ensure fair usage. The specific limits depend on various factors such as the type of API call, user permissions, and the number of active users using your app.
With this beginner’s guide, you now have a solid foundation to start integrating Facebook PHP into your applications. Explore the extensive capabilities of Facebook PHP and leverage the power of Facebook’s social platform to enhance your application’s user experience and engagement.