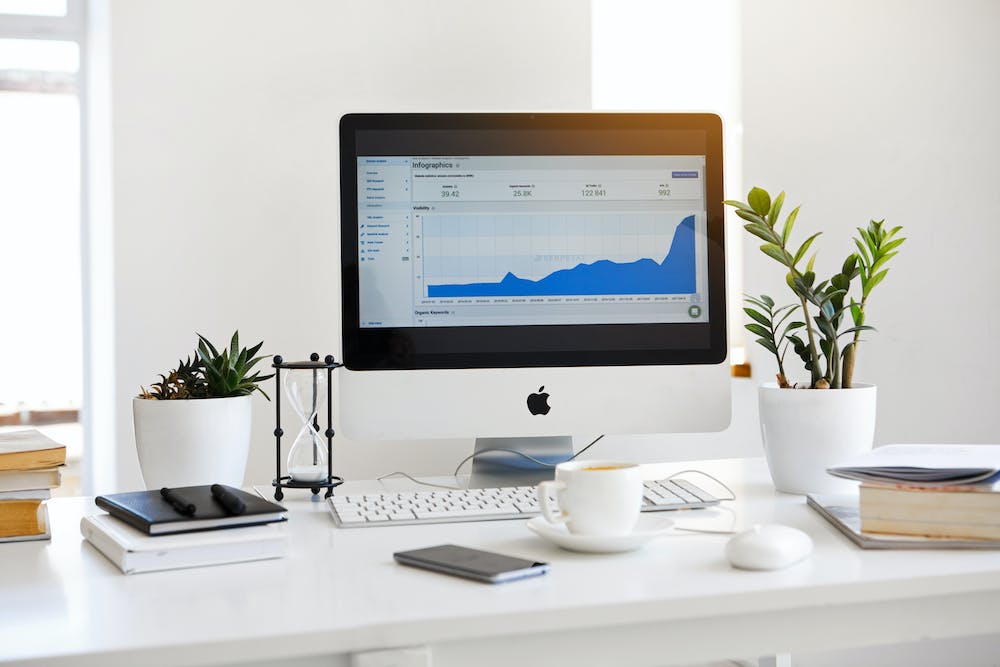
In today’s digital age, the importance of strong passwords cannot be overstated. With the increasing number of cyber threats and data breaches, creating and using strong, random passwords is crucial for protecting sensitive information. In this article, we will explore how to generate random passwords in PHP, a widely used server-side scripting language for web development.
Why Generate Random Passwords?
Random passwords are much more secure than manually created or easily guessable passwords. When you use a random password generator, you ensure that the password is not based on any existing words, phrases, or patterns, making IT harder for hackers to crack. Randomly generated passwords typically contain a combination of uppercase and lowercase letters, numbers, and special characters, further enhancing their complexity.
By generating random passwords, you can significantly reduce the risk of unauthorized access to your systems, accounts, and data. Whether you are developing a web application, managing user authentication, or simply creating a new user account, implementing a random password generator in PHP can greatly enhance the security of your system.
Generating Random Passwords in PHP
PHP provides several built-in functions for generating random passwords. One of the most commonly used functions is random_bytes()
, which creates a cryptographically secure string of random bytes. The following example demonstrates how to use random_bytes()
to generate a random password:
“`php
$password_length = 12;
$random_bytes = random_bytes($password_length);
$password = base64_encode($random_bytes);
echo $password;
?>
“`
In this example, we specify the length of the password as 12 characters and use random_bytes()
to generate a sequence of random bytes. We then encode the random bytes using base64_encode()
to produce a human-readable password. This method ensures that the generated password is cryptographically secure and suitable for use in a wide range of applications.
Additional Considerations
While random_bytes()
is an effective method for generating random passwords, it requires PHP 7 or later. If you need to support older versions of PHP, you can use the openssl_random_pseudo_bytes()
function, which provides a similar functionality. Additionally, you can combine the output of these functions with character set manipulation to customize the generated passwords according to your specific requirements.
When generating random passwords, it is important to consider the length and complexity of the passwords. Typically, passwords should be at least 12-16 characters long and contain a mix of uppercase and lowercase letters, numbers, and special characters. Avoid using easily guessable sequences or common words, as these can compromise the security of the passwords.
Best Practices for Using Random Passwords
Once you have generated random passwords in PHP, there are several best practices to consider when using those passwords:
- Store passwords securely: Always store passwords in hashed and salted form using industry-standard algorithms such as bcrypt or Argon2. Never store passwords in plain text or reversible encryption.
- Enforce password complexity: Implement password policies that require users to create strong, random passwords when setting up their accounts. Consider using a password strength meter to provide real-time feedback on password complexity.
- Rotate passwords regularly: Encourage users to change their passwords periodically, and consider implementing password expiration policies to ensure that old passwords are not reused indefinitely.
- Use multi-factor authentication: Where possible, implement multi-factor authentication to provide an additional layer of security beyond just a password.
Conclusion
Generating random passwords in PHP is a critical step in strengthening the security of your applications, websites, and user accounts. By leveraging the built-in functions for generating random bytes, you can create cryptographically secure passwords that are resistant to both brute-force and dictionary attacks. Remember to consider password length, complexity, and storage best practices to further enhance the security of your system.
FAQs
1. Is it safe to use random passwords generated in PHP?
Yes, random passwords generated in PHP using the provided functions are considered secure and suitable for use in a wide range of applications. However, it is important to ensure that passwords are stored securely and that appropriate password policies are in place to enforce complexity and rotation.
2. How often should I rotate random passwords?
It is recommended to rotate passwords regularly, typically every 60-90 days. This practice helps to mitigate the risk of compromised passwords and ensures that users are regularly updating their credentials.
3. Can I customize the character set for generated passwords?
Yes, you can customize the character set for generated passwords by manipulating the output of the random byte generation functions. This allows you to tailor the passwords to meet specific requirements, such as including or excluding certain characters.
4. Are there any third-party libraries or tools for generating random passwords in PHP?
Yes, there are several third-party libraries and tools available for generating random passwords in PHP. However, it is important to carefully review and vet these tools to ensure that they provide a secure and reliable method for generating random passwords.
Overall, generating random passwords in PHP is a fundamental aspect of ensuring the security of your applications and user accounts. By utilizing the built-in functions for random byte generation and following best practices for password management, you can greatly enhance the resilience of your system against unauthorized access and data breaches.