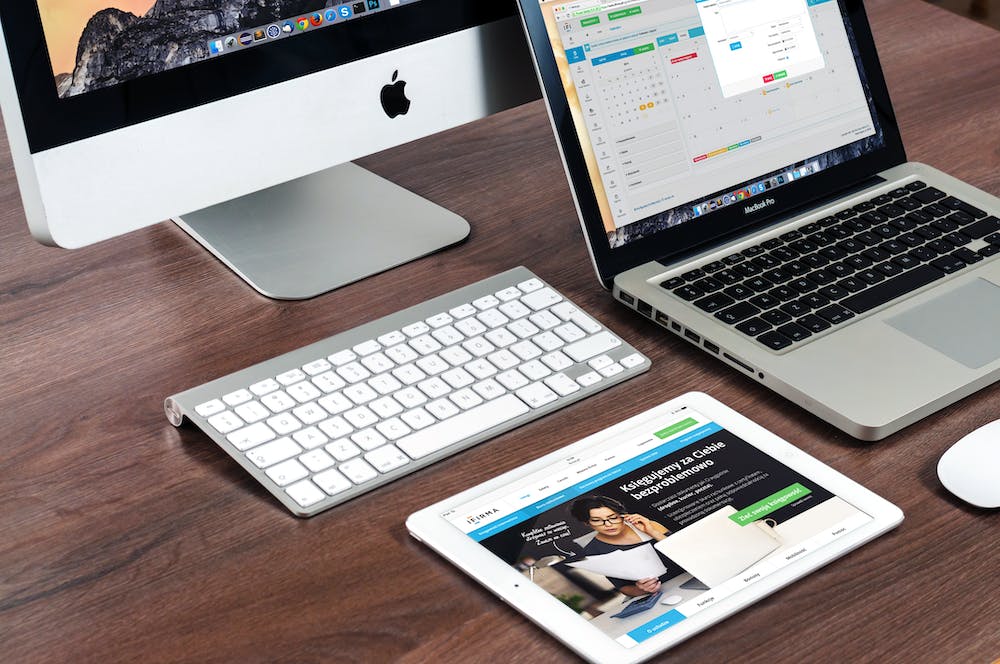
PHP is a powerful programming language that allows developers to manipulate arrays in various ways. One common task when working with arrays is filtering them based on specific criteria. In this comprehensive guide, we will explore different methods for filtering arrays in PHP, along with examples and best practices.
Filtering Arrays using array_filter() function
The array_filter()
function is a built-in PHP function that allows developers to filter an array using a callback function. The callback function is applied to each element of the array, and the elements for which the callback function returns true are retained in the filtered array.
Here’s a simple example of using array_filter()
to filter an array of numbers:
“`php
$numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
$filteredNumbers = array_filter($numbers, function($num) {
return $num % 2 == 0;
});
print_r($filteredNumbers); // Output: [2, 4, 6, 8, 10]
?>
“`
In this example, we use an anonymous function as the callback function to filter out even numbers from the original array. The resulting $filteredNumbers
array contains only the even numbers.
Filtering Associative Arrays
When working with associative arrays, developers often need to filter the array based on the values of specific keys. PHP provides a versatile way to achieve this using the array_filter()
function along with custom callback functions.
Let’s consider an example where we have an associative array of products and we want to filter IT based on the price:
“`php
$products = [
[‘name’ => ‘Product A’, ‘price’ => 100],
[‘name’ => ‘Product B’, ‘price’ => 150],
[‘name’ => ‘Product C’, ‘price’ => 200],
[‘name’ => ‘Product D’, ‘price’ => 120],
];
$filteredProducts = array_filter($products, function($product) {
return $product[‘price’] > 150;
});
print_r($filteredProducts);
?>
“`
In this example, the callback function filters the products based on their price, retaining only the products with a price higher than 150.
Filtering Arrays using array_walk() function
Another approach to filtering arrays in PHP is by using the array_walk()
function. Unlike array_filter()
, array_walk()
operates on the original array without creating a new array for the filtered elements.
Here’s an example of using array_walk()
to filter an array of strings based on the length of the strings:
“`php
$strings = [‘apple’, ‘banana’, ‘orange’, ‘kiwi’, ‘pineapple’];
$filteredStrings = [];
array_walk($strings, function($str) use (&$filteredStrings) {
if (strlen($str) > 5) {
$filteredStrings[] = $str;
}
});
print_r($filteredStrings); // Output: [‘orange’, ‘pineapple’]
?>
“`
In this example, the array_walk()
function is used to filter out strings with a length greater than 5, and the filtered strings are added to the $filteredStrings
array.
Filtering Arrays using custom callback functions
In some cases, developers may need to apply more complex filtering logic to arrays. PHP allows for the creation of custom callback functions to handle such scenarios.
Here’s an example of a custom callback function that filters an array of user objects based on their age:
“`php
class User {
public $name;
public $age;
public function __construct($name, $age) {
$this->name = $name;
$this->age = $age;
}
}
$users = [
new User(‘John’, 25),
new User(‘Alice’, 30),
new User(‘Bob’, 20),
new User(‘Eve’, 28),
];
function filterUsersByAge($users, $minAge) {
$filteredUsers = [];
foreach ($users as $user) {
if ($user->age >= $minAge) {
$filteredUsers[] = $user;
}
}
return $filteredUsers;
}
$filteredUsers = filterUsersByAge($users, 25);
print_r($filteredUsers);
?>
“`
In this example, we define a custom callback function filterUsersByAge()
that filters the array of user objects based on their age. The function returns a new array containing only the users that meet the specified age criteria.
Conclusion
Filtering arrays in PHP is a common task that developers encounter when working with arrays. PHP provides several built-in functions, such as array_filter()
and array_walk()
, as well as the ability to create custom callback functions for more complex filtering logic. By mastering these techniques, developers can efficiently filter arrays based on specific criteria, resulting in cleaner and more organized code.
FAQs
Q: What is the difference between array_filter() and array_walk()?
A: The array_filter()
function creates a new array containing only the elements that satisfy the specified criteria, while array_walk()
operates on the original array without creating a new array.
Q: Can I use array_filter() on multidimensional arrays?
A: Yes, you can use array_filter()
on multidimensional arrays by providing a custom callback function to handle the filtering logic for nested arrays.
Q: Are custom callback functions for array filtering reusable?
A: Yes, custom callback functions for array filtering are reusable and can be applied to different arrays as long as the filtering criteria remain consistent.