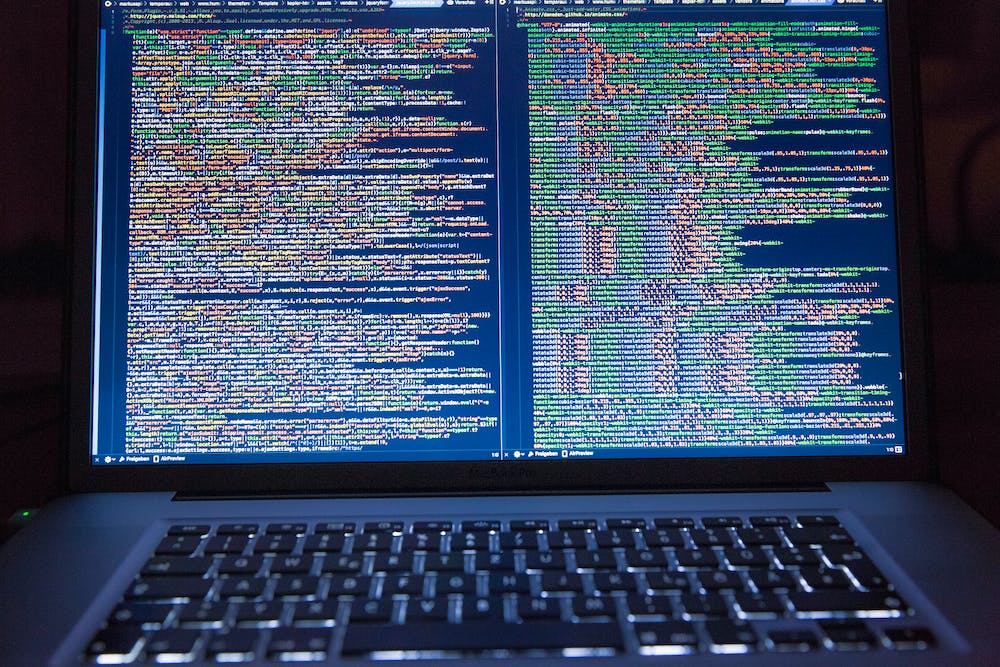
PHP is a popular scripting language for web development, and IT comes with a wide range of built-in functions and libraries that make it a versatile tool for processing and manipulating XML data. One such function is xml2array
, which allows developers to convert XML data into an array structure that can be easily manipulated and processed. In this article, we will explore the power of PHP’s xml2array
function and how it can be used to streamline XML data processing in web development.
Understanding XML Data
XML, or Extensible Markup Language, is a popular format for storing and sharing structured data. It is commonly used for representing hierarchical data in a human-readable format, and it is widely used in web development for tasks such as data interchange and configuration settings. XML data is structured using nested elements, which are defined by start and end tags.
For example, consider the following XML data:
<bookstore>
<book category="fiction">
<title>Harry Potter</title>
<author>J.K. Rowling</author>
</book>
<book category="non-fiction">
<title>The Lean Startup</title>
<author>Eric Ries</author>
</book>
</bookstore>
This XML data represents a bookstore with two books, each with a category, title, and author. The data is structured hierarchically, with the bookstore
element at the top level, and nested book
elements representing individual books.
Introducing PHP’s xml2array Function
PHP’s xml2array
function is a powerful tool for parsing XML data and converting it into a multidimensional array structure. This makes it easier for developers to manipulate and process XML data using familiar array manipulation techniques.
The xml2array
function is part of the SimpleXML
extension, which is enabled by default in most PHP installations. It takes an XML string as input and returns an array that represents the hierarchical structure of the XML data. This allows developers to access and manipulate the XML data using array indexing and iteration.
Using xml2array to Parse XML Data
Let’s take a look at how we can use the xml2array
function to parse the example XML data from earlier:
$xmlString = '
<bookstore>
<book category="fiction">
<title>Harry Potter</title>
<author>J.K. Rowling</author>
</book>
<book category="non-fiction">
<title>The Lean Startup</title>
<author>Eric Ries</author>
</book>
</bookstore>
';
$xml = simplexml_load_string($xmlString);
$array = json_decode(json_encode($xml), true);
print_r($array);
When we run this code, we will see the following output:
Array
(
[book] => Array
(
[0] => Array
(
[category] => fiction
[title] => Harry Potter
[author] => J.K. Rowling
)
[1] => Array
(
[category] => non-fiction
[title] => The Lean Startup
[author] => Eric Ries
)
)
)
As we can see, the xml2array
function has successfully converted the XML data into a multidimensional array structure. This allows us to access and manipulate the data using array indexing, which can be much more convenient than working directly with XML elements.
Advantages of Using xml2array in PHP
There are several compelling reasons to use the xml2array
function in PHP for processing XML data:
- Simplicity: Working with arrays is often more intuitive and simpler than working directly with XML elements, especially for developers who are more familiar with array manipulation than XML processing.
- Compatibility: Arrays are a fundamental data structure in PHP, and using arrays to represent XML data can make it easier to integrate XML processing into existing PHP codebases.
- Flexibility: Arrays can be easily manipulated and transformed using PHP’s array functions, providing a high degree of flexibility when working with XML data.
- Performance: In some cases, processing XML data as an array can be more efficient than working with XML elements directly, especially for large datasets.
Best Practices for Using xml2array in PHP
While the xml2array
function can be a powerful tool for XML data processing, it is important to follow best practices to ensure that your code is robust and maintainable. Here are a few tips for using xml2array
effectively:
- Sanitize input: Always validate and sanitize XML input before passing it to the
xml2array
function to prevent security vulnerabilities such as XML injection attacks. - Handle errors gracefully: XML data may not always be well-formed or valid, so it is important to handle parsing errors gracefully and provide informative error messages to users or log files.
- Optimize performance: If you are working with large XML datasets, consider optimizing your code for performance by using streaming XML parsing or caching parsed data to reduce the overhead of repeated parsing.
- Use appropriate data structures: When converting XML data to an array, consider the best way to represent the hierarchical structure of the XML data as a multidimensional array, taking into account the specific requirements of your application.
Conclusion
PHP’s xml2array
function is a valuable tool for developers working with XML data in web development. By converting XML data into a multidimensional array structure, xml2array
simplifies the process of manipulating and processing XML data using familiar array manipulation techniques. When used effectively and following best practices, xml2array
can streamline XML data processing and enhance the performance and maintainability of PHP applications.
Frequently Asked Questions (FAQs)
Q: Can the xml2array function handle complex XML structures?
A: Yes, the xml2array function can handle complex XML structures with nested elements and attributes, converting them into a corresponding multidimensional array structure.
Q: Are there any limitations or drawbacks to using xml2array?
A: While xml2array is a powerful tool, it’s important to be aware of potential limitations such as memory usage and performance overhead when working with large XML datasets. Additionally, xml2array may not always preserve the exact structure and ordering of the original XML data, so it’s important to test and validate the results in your specific use case.
Q: Are there alternative approaches to parsing and processing XML data in PHP?
A: Yes, PHP offers several other options for parsing and processing XML data, such as the DOMDocument and SimpleXML extensions. Each approach has its own strengths and weaknesses, so it’s important to choose the best tool for your specific requirements and performance considerations.
Q: Can xml2array be used for generating XML data from arrays?
A: No, xml2array is specifically designed for parsing XML data into arrays. To generate XML data from arrays, developers can use the array2xml function or consider using the SimpleXMLElement class for creating XML documents from arrays.
Q: Is xml2array compatible with all versions of PHP?
A: Yes, xml2array is part of the SimpleXML extension, which is enabled by default in PHP 5 and later versions. However, it’s always a good practice to check the PHP documentation for the specific version compatibility of any built-in functions or extensions.
Q: Where can I find more information and examples of using xml2array in PHP?
A: The official PHP documentation provides comprehensive information and examples for using the xml2array function, along with user-contributed notes and examples that can provide valuable insights and best practices for working with XML data in PHP.