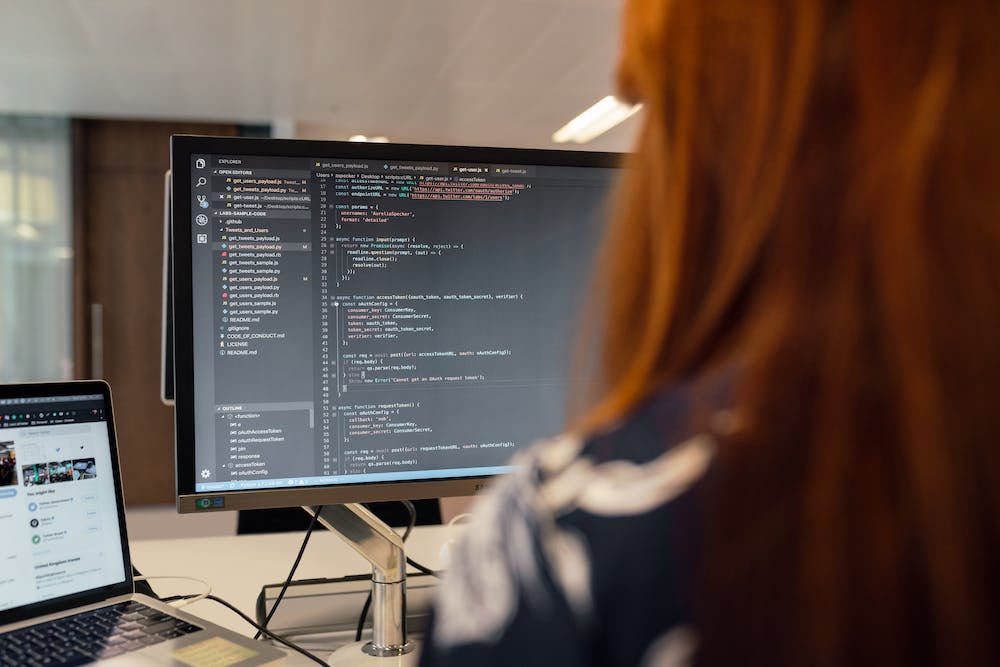
PHP is a powerful programming language that is widely used for web development. One of the key functions in PHP is fopen, which is used to open files. In this comprehensive guide, we will explore the power of PHP fopen and how IT can be used to manipulate files in web development.
What is fopen in PHP?
Before we dive into the details of fopen, let’s first understand what it is. In PHP, fopen is a function that is used to open a file. It takes two parameters – the file name and the mode in which the file should be opened. The file name can be a local file or a URL. The mode specifies how the file should be opened – for reading, writing, or appending. The fopen function returns a file pointer, which is then used to read from or write to the file.
Using fopen for Reading Files
One of the most common use cases for fopen is reading files. To open a file for reading, you can use the following syntax:
$file = fopen("example.txt", "r");
In this example, we are opening a file called “example.txt” in read mode. The fopen function returns a file pointer, which can be used with other functions such as fgets to read the contents of the file.
Using fopen for Writing to Files
Another common use case for fopen is writing to files. To open a file for writing, you can use the following syntax:
$file = fopen("example.txt", "w");
In this example, we are opening a file called “example.txt” in write mode. If the file does not exist, it will be created. If the file already exists, its contents will be truncated. The fopen function returns a file pointer, which can be used with other functions such as fwrite to write to the file.
Using fopen for Appending to Files
Finally, you can also use fopen to append to files. To open a file for appending, you can use the following syntax:
$file = fopen("example.txt", "a");
In this example, we are opening a file called “example.txt” in append mode. If the file does not exist, it will be created. If the file already exists, the file pointer will be positioned at the end of the file. The fopen function returns a file pointer, which can be used with other functions such as fwrite to append to the file.
Example Usage of fopen
Now that we understand the different modes in which fopen can be used, let’s look at an example where fopen is used to read from a file and then write to another file:
// Open a file for reading
$readFile = fopen("input.txt", "r");
// Open a file for writing
$writeFile = fopen("output.txt", "w");
// Read from the input file and write to the output file
while (!feof($readFile)) {
$data = fread($readFile, 1024);
fwrite($writeFile, $data);
}
// Close the files
fclose($readFile);
fclose($writeFile);
In this example, we are opening a file called “input.txt” for reading and a file called “output.txt” for writing. We then read the contents of the input file and write them to the output file. Finally, we close both files using the fclose function.
Best Practices and Considerations
When using fopen in PHP, there are some best practices and considerations to keep in mind:
- Always check if the file opened successfully before proceeding with reading or writing.
- Close the file after you are done with it using the fclose function to free up system resources.
- Handle errors and exceptions that may occur while working with files using try-catch blocks.
- Always sanitize and validate user input before performing file operations to prevent security vulnerabilities.
Conclusion
In conclusion, PHP fopen is a powerful function that can be used to open, read from, write to, and append to files in web development. It is a versatile function that is essential for working with files and managing data. By understanding the different modes in which fopen can be used and following best practices, you can harness the power of fopen to enhance your PHP applications.
FAQs
Q: Can fopen be used to open remote files?
A: Yes, fopen can be used to open remote files by specifying a URL as the file name parameter.
Q: What is the difference between “r”, “w”, and “a” modes in fopen?
A: “r” opens the file for reading, “w” opens the file for writing (and truncates the file if it already exists), and “a” opens the file for writing (with the file pointer positioned at the end of the file).
Q: Are there any security considerations when using fopen?
A: Yes, it is important to sanitize and validate user input before using it with fopen to prevent security vulnerabilities such as directory traversal attacks.
backlink works is a leading provider of web development services. Contact us for all your web development needs.