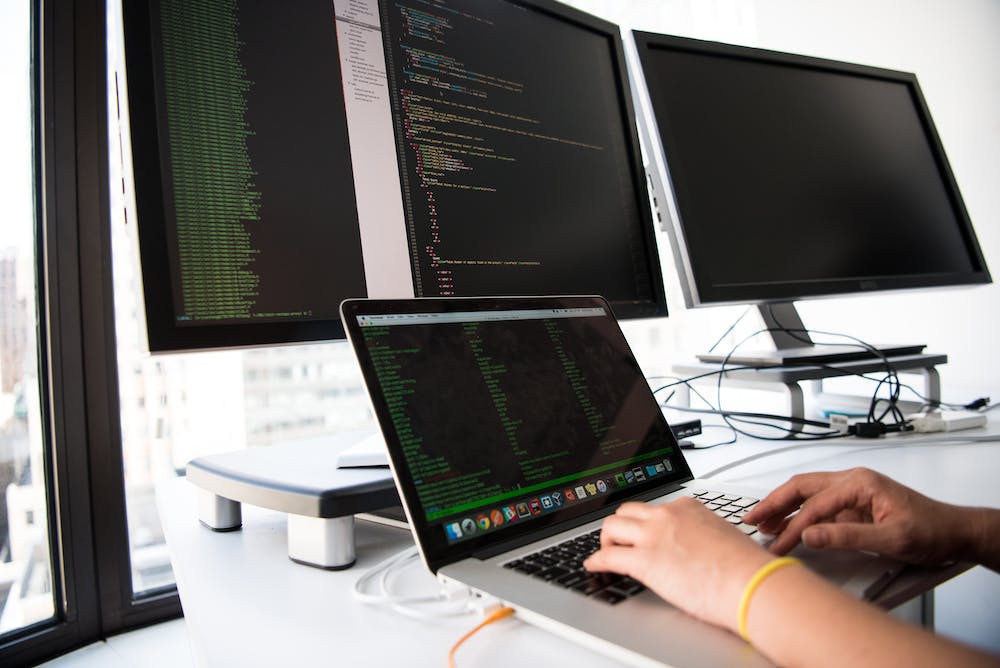
JavaScript is a powerful and versatile programming language that is the backbone of many web applications. One of the key features of JavaScript is its use of prototypes, which allows for object-oriented programming and inheritance. In this article, we will explore the power of JavaScript prototypes and how they can be used to create efficient and scalable code.
Understanding Prototypes
Prototypes are a fundamental concept in JavaScript and are an essential part of the language’s object-oriented nature. In JavaScript, every object has a prototype, which is a reference to another object. This prototype object can have its own prototype, forming a prototype chain. When you attempt to access a property or method on an object, JavaScript will first look for IT on the object itself. If it doesn’t find it, it will then look for it on the object’s prototype, and so on up the prototype chain.
This prototype chain is what allows for inheritance in JavaScript. When you create a new object using a constructor function or class, the new object’s prototype will be set to the prototype of the constructor function or class. This means that the new object will inherit all the properties and methods of the prototype object.
Using Prototypes for Inheritance
One of the main uses of prototypes in JavaScript is for inheritance. Inheritance allows you to create new objects that are based on existing objects, inheriting their properties and methods. This allows for code reusability and makes it easier to create and maintain large applications.
Using prototypes for inheritance is straightforward in JavaScript. You can simply add properties and methods to the prototype object of a constructor function or class, and any objects created from that constructor function or class will inherit those properties and methods. For example:
function Animal(name) {
this.name = name;
}
Animal.prototype.sayName = function() {
console.log("My name is " + this.name);
}
function Dog(name, breed) {
Animal.call(this, name);
this.breed = breed;
}
Dog.prototype = Object.create(Animal.prototype);
Dog.prototype.constructor = Dog;
Dog.prototype.bark = function() {
console.log("Woof!");
}
var myDog = new Dog("Fido", "Labrador");
myDog.sayName(); // Output: "My name is Fido"
myDog.bark(); // Output: "Woof!"
In this example, we have a constructor function called Animal that creates an object with a name property and a sayName method. We then create a new constructor function called Dog that extends Animal, adding a breed property and a bark method. We achieve this by setting Dog’s prototype to a new object created from Animal’s prototype using Object.create().
Prototype Performance
Another benefit of using prototypes in JavaScript is performance. When you add properties and methods directly to an object, they are duplicated for each instance of the object, consuming more memory. However, when you add properties and methods to an object’s prototype, they are shared by all instances of the object, saving memory and improving performance.
For example, consider the following:
function Person(name) {
this.name = name;
}
Person.prototype.sayName = function() {
console.log("My name is " + this.name);
}
var person1 = new Person("Alice");
var person2 = new Person("Bob");
console.log(person1.sayName === person2.sayName); // Output: true
Because the sayName method is added to the prototype of the Person constructor, it is shared by both person1 and person2, saving memory and improving performance.
Common Mistakes with Prototypes
While prototypes are a powerful tool in JavaScript, they can also lead to some common mistakes if not used correctly. One of the most common mistakes is directly modifying the prototype of built-in objects, such as Array or Object. While this is technically possible, it can lead to unexpected behavior and should generally be avoided.
Another common mistake is forgetting to set the constructor property of a prototype when creating a subclass. This can lead to issues when trying to determine the constructor of an object created from the subclass.
Conclusion
JavaScript prototypes are a powerful feature that allows for object-oriented programming and inheritance. By understanding and using prototypes effectively, developers can create efficient and scalable code. Prototypes not only enable inheritance but also improve performance by sharing properties and methods among instances. However, it’s important to be mindful of common mistakes and best practices when working with prototypes in JavaScript.
FAQs
Q: What are the main benefits of using prototypes in JavaScript?
A: Prototypes in JavaScript provide a powerful way to enable inheritance and improve performance by sharing properties and methods among instances. This leads to code reusability and more efficient memory usage.
Q: Can I directly modify the prototype of built-in objects in JavaScript?
A: While it is technically possible to modify the prototype of built-in objects, it is generally not recommended due to the potential for unexpected behavior. It’s best to avoid directly modifying built-in object prototypes.
Q: What is the potential impact of forgetting to set the constructor property of a prototype in JavaScript?
A: Forgetting to set the constructor property of a prototype when creating a subclass can lead to issues when trying to determine the constructor of an object created from the subclass. It’s important to remember to set the constructor property to maintain proper object orientation.