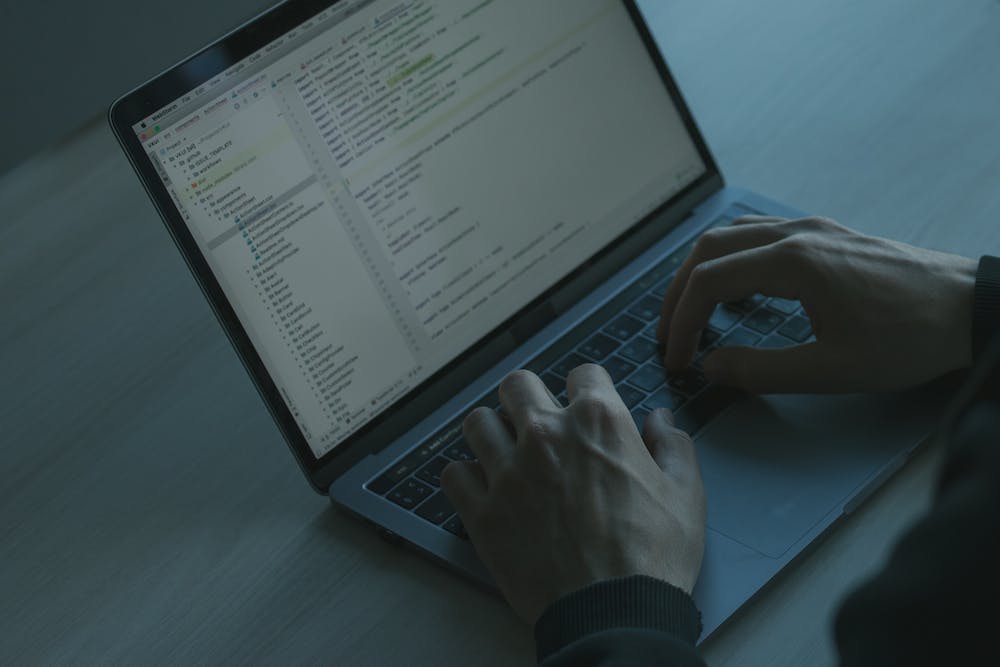
PHP is a popular server-side scripting language widely used for web development. One of the useful functions PHP provides is the random function. The random function generates a random number or a random string, allowing developers to add randomness to their applications. In this article, we will explore the PHP random function in depth, covering its usage, parameters, and different ways to generate random values. Whether you are a beginner or an experienced PHP developer, this comprehensive guide will provide you with all the information you need.
Before we dive into the details, let’s first understand what randomness means in the context of computer programming. Randomness refers to the lack of predictability in a sequence of values. In other words, a random value cannot be easily guessed or determined in advance. The PHP random function enables developers to incorporate randomness into their applications, which is particularly useful in scenarios such as generating random numbers for games, creating unique identifiers or passwords, shuffling content, or conducting randomized experiments.
Generating Random Numbers
The random function in PHP allows you to generate random numbers within a specified range. Let’s look at an example:
“`php
$randomNumber = rand(1, 100);
echo “Random number between 1 and 100: ” . $randomNumber;
“`
In the example above, the `rand` function generates a random number between 1 and 100, inclusive. The generated number is then stored in the `$randomNumber` variable and displayed using the `echo` statement.
Alternatively, you can use the `mt_rand` function for generating random numbers:
“`php
$randomNumber = mt_rand(1, 100);
echo “Random number between 1 and 100: ” . $randomNumber;
“`
The `mt_rand` function is generally faster than `rand`, especially when generating a large number of random values.
Generating Random Strings
In addition to generating random numbers, PHP also provides functions for generating random strings. You can use the `str_shuffle` function to shuffle the characters of a string:
“`php
$string = “Hello, World!”;
$shuffledString = str_shuffle($string);
echo “Shuffled string: ” . $shuffledString;
“`
The `str_shuffle` function shuffles the characters of the input string, producing a randomized version. You can use this function to generate random passwords, randomize the display order of items, or add randomness to text-based games.
Another way to generate random strings is by using the `random_bytes` function, which returns random binary data:
“`php
$randomString = bin2hex(random_bytes(10));
echo “Random string: ” . $randomString;
“`
In this example, `random_bytes` generates 10 random bytes of data, which are then converted to a hexadecimal representation using `bin2hex`. This results in a random string of 20 characters in length.
IT is important to note that the randomness of these functions depends on the quality of the underlying random number generator (RNG) used by PHP. PHP uses the system’s default RNG, so the actual randomness may vary depending on the operating system and PHP configuration.
Frequently Asked Questions
-
Q: Can I generate random numbers without a specified range?
A: Yes, you can generate random numbers without specifying a range by omitting the second parameter in the `rand` or `mt_rand` functions. For example:
$randomNumber = rand();
-
Q: How can I set the seed for the random number generator?
A: PHP automatically seeds the random number generator with a value obtained from the system time. If you need to set a specific seed, you can use the `srand` function. For example:
srand(12345);
-
Q: Can I generate cryptographically secure random values?
A: Yes, PHP provides the `random_int` and `random_bytes` functions for generating cryptographically secure random integers and bytes, respectively. These functions use the underlying operating system’s cryptographically secure RNG.
With the PHP random function and its various capabilities, you have the power to introduce randomness and unpredictability into your applications. Whether you need to generate random numbers or random strings, PHP has got you covered. So go ahead and leverage the random function to make your applications more dynamic and exciting!