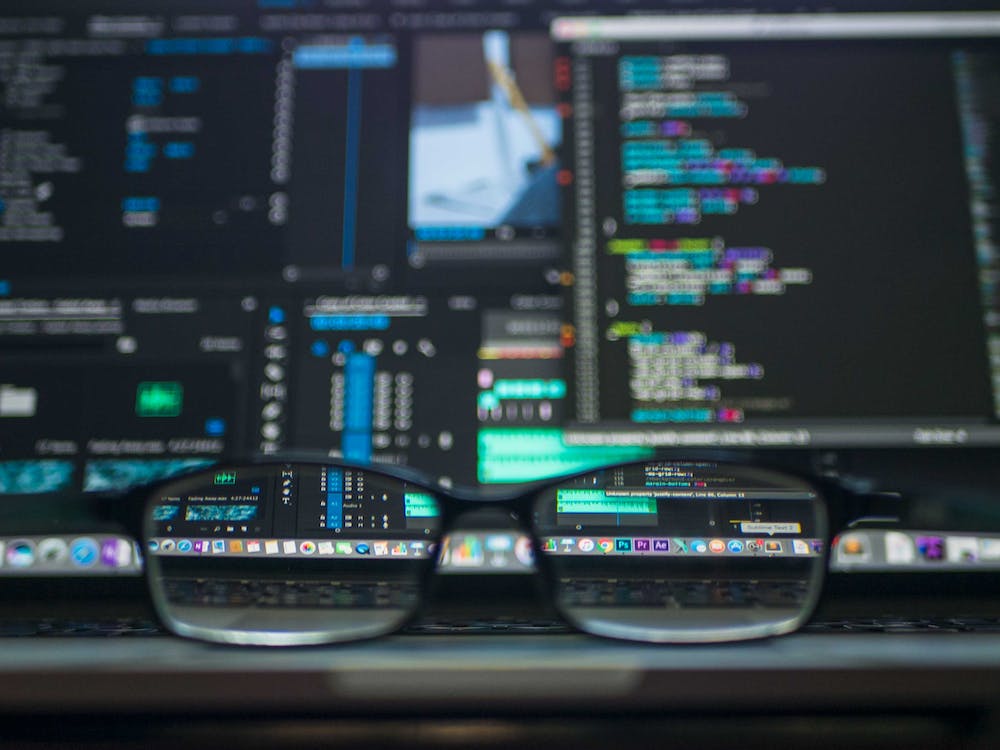
JavaScript, the programming language of the web, is constantly evolving to meet the demands of modern web development. In this comprehensive guide, we will explore the latest features that have been introduced in JavaScript, highlighting their benefits and providing examples of how to use them effectively. Whether you are a seasoned developer or just starting out, staying up-to-date with these latest advancements is vital to enhance your coding skills and deliver innovative web applications.
1. Arrow Functions
Arrow functions are a concise syntax introduced in JavaScript ES6 that provides a more streamlined way of writing functions. They offer a shorter syntax compared to traditional function expressions, reducing code clutter and improving readability. An arrow function does not bind its own ‘this’ value, resulting in a cleaner and more predictable code structure.
2. Destructuring Assignment
Destructuring assignment allows developers to extract elements from arrays or objects and assign them to variables in a single line. This feature simplifies code and enhances readability by eliminating the need for repetitive variable assignments. With destructuring assignment, you can access nested data structures quickly and efficiently.
3. Template Literals
Template literals, also known as template strings, provide an elegant way to concatenate strings in JavaScript. With template literals, you can embed expressions within backticks (`) and easily interpolate variables or expressions into strings. This feature allows for better readability and more flexible string manipulation, making your code more maintainable.
4. Async/Await
The introduction of async/await in JavaScript ES8 brought significant improvements to asynchronous programming. Async functions, marked with the ‘async’ keyword, enable developers to write asynchronous code that looks and behaves like synchronous code. Paired with the ‘await’ keyword, which waits for a promise to resolve or reject, async/await simplifies error handling and enhances the overall readability of asynchronous code.
5. Modules
Prior to JavaScript ES6, developers used various module systems like CommonJS and AMD to organize their code. With the inclusion of native modules in ES6, JavaScript now has built-in support for modules. Modules allow developers to encapsulate code into separate files, promoting modularity and reusability. With imports and exports, modules enable better code organization and reduce the chances of naming conflicts.
6. Optional Chaining
The optional chaining operator (‘?.’) provides a graceful way of accessing properties or calling functions that may be undefined or null. By using optional chaining, you can avoid multiple conditional statements or nested if checks, leading to more concise and readable code. This feature is particularly useful when dealing with complex data structures or when accessing properties of an object that may not exist.
7. Promises
Introduced in JavaScript ES6, promises provide a cleaner alternative to working with asynchronous code compared to callbacks. Promises represent the eventual completion or failure of an asynchronous operation, allowing better control over asynchronous workflows. By chaining ‘then()’ and ‘catch()’ methods, you can handle success and error cases respectively, offering a more structured and maintainable approach to asynchronous programming.
8. FAQs
Q: How can I check if a JavaScript feature is supported by a specific browser?
A: You can check if a specific JavaScript feature is supported by a browser using feature detection techniques. Popular libraries like Modernizr provide easy-to-use methods for detecting the availability of certain features, allowing you to provide fallbacks or alternative solutions when necessary.
Q: Are these new JavaScript features available in all browsers?
A: While most modern browsers support the latest JavaScript features, older versions may lack support for some of the newer functionalities. To ensure cross-browser compatibility, you can use tools like Babel to transpile your code into the widely supported ECMAScript 5 (ES5) version.
Q: Can I start using these features in my current projects?
A: Yes, you can start using these latest JavaScript features in your projects, especially if you target modern browsers or use transpilers like Babel. However, IT‘s important to consider the browser compatibility requirements of your project and implement fallback strategies if needed.
Q: Where can I learn more about advanced JavaScript concepts and features?
A: There are many online resources available to learn about advanced JavaScript concepts and the latest features. Websites like MDN Web Docs, JavaScript.info, and various online courses and tutorials provide comprehensive guides and examples to expand your knowledge and skills.