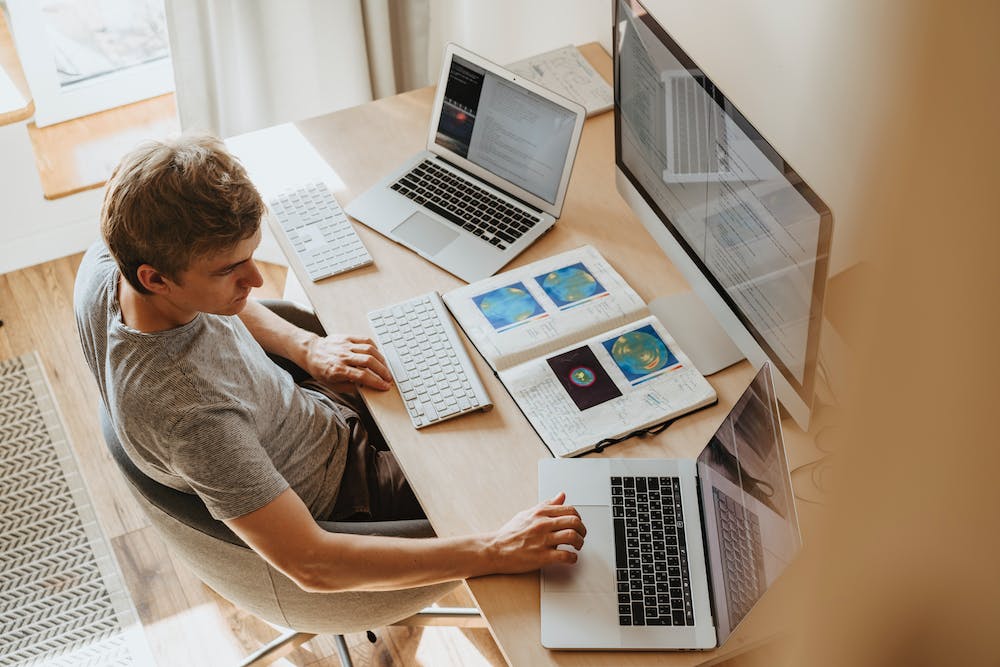
When IT comes to efficient database querying, developers need to utilize the right tools and functions to ensure that their applications are running smoothly and quickly. One such tool in the PHP programming language is the mysqli_fetch_row
function, which provides a way to efficiently retrieve data from a MySQL database.
In this article, we will explore the functionality of mysqli_fetch_row
and how it can be used to streamline database querying for better performance and user experience.
Understanding mysqli_fetch_row
Before diving into the specifics of mysqli_fetch_row
, it’s important to have a basic understanding of the MySQLi extension for PHP. MySQLi, which stands for MySQL Improved, is a set of functions and classes that provide a way to interact with a MySQL database. It offers a more robust and feature-rich interface compared to the older MySQL extension.
The mysqli_fetch_row
function is used to fetch a single row from the result set returned by a SELECT query. It returns an array that contains the values of the columns in the result set. Each call to mysqli_fetch_row
advances the result set pointer to the next row, allowing developers to iterate through the result set and retrieve the necessary data.
Efficient Database Querying with mysqli_fetch_row
One of the key benefits of using mysqli_fetch_row
for database querying is its efficiency. By fetching only a single row at a time, developers can conserve memory and reduce the overhead associated with retrieving large result sets. This can lead to improved performance and responsiveness in applications that rely on database queries to retrieve and display data.
Furthermore, mysqli_fetch_row
can be particularly useful when dealing with large result sets that would consume a significant amount of memory if fetched all at once. Instead, developers can iterate through the result set using mysqli_fetch_row
to retrieve and process the data one row at a time, thereby minimizing memory usage and optimizing the overall performance of the application.
Example Usage of mysqli_fetch_row
Let’s consider an example to demonstrate how mysqli_fetch_row
can be used in a real-world scenario. Suppose we have a table named users
in a MySQL database, and we want to retrieve and display the names of all the users in the table.
<?php
// Connect to the database
$conn = new mysqli('localhost', 'username', 'password', 'dbname');
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// Construct the query
$query = "SELECT name FROM users";
// Execute the query
$result = $conn->query($query);
// Fetch and display the results
while ($row = $result->fetch_row()) {
echo $row[0] . " <br>";
}
// Close the connection
$conn->close();
?>
In this example, we establish a connection to the database using the mysqli
class, construct a SELECT query to retrieve the names of all users from the users
table, and then use mysqli_fetch_row
in a loop to fetch and display the names of the users.
Conclusion
Overall, mysqli_fetch_row
is a valuable function for efficient database querying in PHP applications. Its ability to fetch a single row at a time allows developers to conserve memory and optimize performance when dealing with large result sets. By leveraging the functionality of mysqli_fetch_row
, developers can ensure that their database queries are executed in a way that maximizes efficiency and responsiveness.
FAQs
Q: Are there any limitations to using mysqli_fetch_row?
A: While mysqli_fetch_row is efficient for fetching large result sets, it returns data as a numerical array, which may not be suitable for all use cases. Developers should consider the structure of the result set and the type of data being fetched when deciding whether to use mysqli_fetch_row.
Q: How does mysqli_fetch_row compare to other fetching functions in MySQLi?
A: mysqli_fetch_row is just one of several fetching functions available in MySQLi, each with its own specific use cases. Developers should choose the appropriate fetching function based on the structure and requirements of the result set they are working with.
Q: Can mysqli_fetch_row be used for non-SELECT queries?
A: No, mysqli_fetch_row is specifically designed for fetching rows from the result set of a SELECT query. For non-SELECT queries, developers should use other methods provided by the MySQLi extension.
By leveraging the functionality of mysqli_fetch_row
, developers can ensure that their database queries are executed in a way that maximizes efficiency and responsiveness.