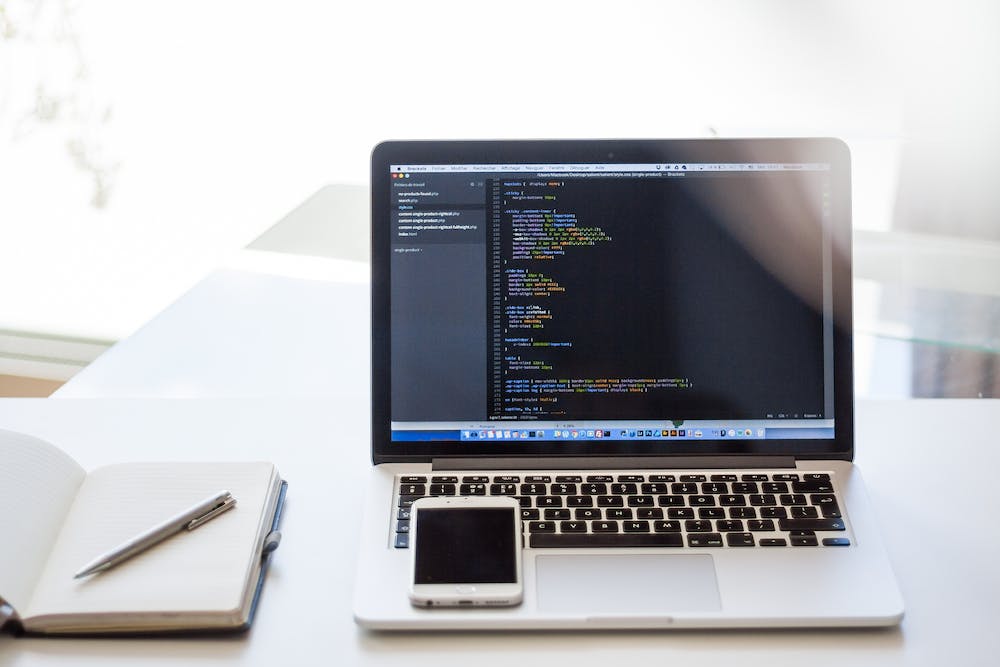
In JavaScript, the forEach loop is a powerful tool that allows developers to iterate over elements in an array or collection. IT provides an easy and efficient way to perform a specific action on each item within the array. This article will explore the syntax and functionality of the forEach loop, as well as provide real-world examples and use cases to demonstrate its effectiveness.
Syntax of the forEach Loop
The forEach loop is a method that is available to arrays in JavaScript. Its syntax is as follows:
array.forEach(function(element, index, array) {
// code to be executed on each element
});
Here, array
is the array that will be iterated over, and function
is the action to be performed on each element within the array. The element
parameter represents the current element being processed, index
represents the index of the current element, and array
is a reference to the array itself. The function is executed for each element within the array.
Using the forEach Loop
Let’s take a look at a simple example of how to use the forEach loop to iterate over an array of numbers and print each element to the console:
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(function(number) {
console.log(number);
});
In this example, the forEach loop iterates over the numbers
array and logs each element to the console. The function
takes the number
parameter, which represents the current element being processed. The result will be:
1
2
3
4
5
Real-World Use Cases
The forEach loop has many practical applications in web development. Let’s explore some real-world use cases to demonstrate its versatility.
Updating HTML Elements
One common use case for the forEach loop is updating HTML elements based on data from an array. For example, suppose we have an array of product names that we want to display on a webpage:
const products = ['Shirt', 'Pants', 'Shoes'];
const productList = document.getElementById('product-list');
products.forEach(function(product) {
const li = document.createElement('li');
li.textContent = product;
productList.appendChild(li);
});
In this example, we create a new li
element for each product in the array and append it to the productList
element in the HTML. This allows us to dynamically update the webpage with the product names from the array.
Calculating Total
Another use case for the forEach loop is performing calculations on array elements. Let’s say we have an array of prices and we want to calculate the total cost:
const prices = [10, 20, 30, 40, 50];
let total = 0;
prices.forEach(function(price) {
total += price;
});
console.log(`Total cost: $${total}`);
In this example, we use the forEach loop to iterate over the prices
array and calculate the total cost by adding each price to the total
variable. The result will be:
Total cost: $150
Conclusion
The forEach loop in JavaScript is a powerful tool for iterating over elements in an array and performing a specific action on each item. Its simple syntax and versatility make it a valuable asset for web developers. By utilizing the forEach loop, developers can easily update HTML elements, perform calculations, and handle other tasks with ease. Understanding how to use the forEach loop effectively can greatly enhance the functionality of JavaScript applications.
FAQs
What is the difference between forEach and for loop in JavaScript?
The main difference between forEach and for loop in JavaScript is that forEach is a method that is available to arrays, while the for loop is a general-purpose loop that can be used with various data structures. The forEach loop is often preferred for its simplicity and readability when working with arrays.
Can I break out of a forEach loop?
No, the forEach method does not support breaking out of the loop like the traditional for loop. If you need to break out of a loop, you may consider using a for loop or a different method such as some() or every() in JavaScript.
Is the forEach loop compatible with all browsers?
Yes, the forEach loop is compatible with all modern browsers, including Chrome, Firefox, Safari, and Edge. It is a part of the ECMAScript 5 specification, which is widely supported in modern web browsers.