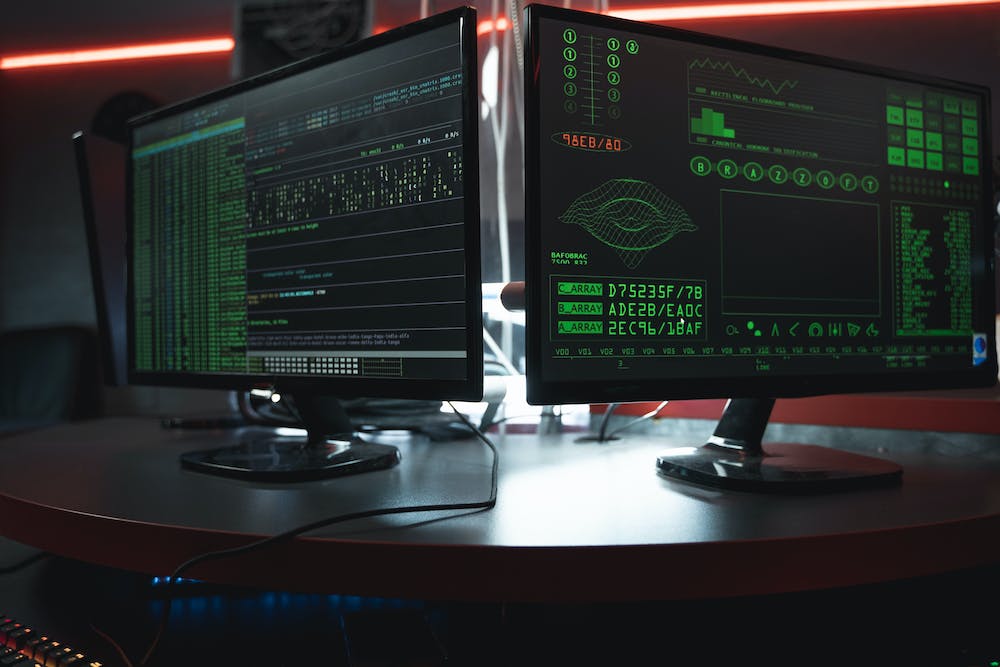
Prototype.js is a powerful JavaScript framework that provides a rich set of features and functionality for web development. IT has gained popularity among developers for its ease of use and robust capabilities. In this article, we will explore the key features and functionality of Prototype.js, and also discuss how it can be used to enhance the development of modern web applications.
Overview of Prototype.js
Prototype.js is an open-source JavaScript framework that was developed by Sam Stephenson. It is designed to simplify the process of writing complex JavaScript code and provides a wide range of utilities and APIs for various tasks such as DOM manipulation, event handling, Ajax requests, and much more.
One of the standout features of Prototype.js is its object-oriented nature, which allows developers to create and manipulate objects in a more organized and efficient manner. It also provides a set of useful classes and methods that can be used to streamline the development process and improve code readability and maintainability.
Key Features of Prototype.js
Prototype.js offers a plethora of features that make it a valuable tool for web developers. Some of the key features include:
DOM Manipulation
Prototype.js provides a simple and intuitive API for manipulating the Document Object Model (DOM). Developers can easily select elements, modify their attributes and content, and perform various operations on the DOM using Prototype.js. This makes it easier to create dynamic and interactive user interfaces for web applications.
Event Handling
Event handling is an essential aspect of web development, and Prototype.js simplifies this process by providing a comprehensive event handling system. Developers can easily attach event listeners to elements, handle user interactions, and create rich and responsive user experiences with the help of Prototype.js.
Ajax Requests
Prototype.js includes a powerful set of APIs for making asynchronous HTTP requests using Ajax. This allows developers to fetch data from the server and update the content of web pages without having to reload the entire page. Prototype.js also provides support for handling response data and managing the state of Ajax requests, making it a valuable tool for building dynamic and interactive web applications.
Utilities and Helpers
Prototype.js comes with a wide range of utility functions and helper methods that can be used to perform common tasks such as string manipulation, array operations, and more. These utilities streamline the development process and help developers write cleaner and more efficient code.
Object-Oriented Programming
Prototype.js embraces the principles of object-oriented programming, allowing developers to create and manipulate objects in a more organized and efficient manner. This makes it easier to structure code, reuse components, and build scalable and maintainable web applications.
Exploring the Functionality of Prototype.js
Let’s dive deeper into the functionality of Prototype.js and explore some of its capabilities in more detail.
Example: DOM Manipulation
Here’s an example of how Prototype.js can be used to manipulate the DOM:
“`javascript
// Selecting an element
var element = $$(‘div#myDiv’)[0];
// Modifying its content
element.update(‘Hello, World!’);
// Adding a CSS class
element.addClassName(‘highlight’);
// Handling a click event
element.observe(‘click’, function() {
alert(‘Element clicked!’);
});
“`
In this example, we use Prototype.js to select an element with the ID “myDiv”, modify its content, add a CSS class to it, and attach a click event listener to it. This demonstrates how Prototype.js simplifies the process of working with the DOM and creating interactive user interfaces.
Example: Ajax Requests
Prototype.js also provides a convenient API for making Ajax requests. Here’s an example of how Ajax requests can be made using Prototype.js:
“`javascript
// Making an Ajax request
new Ajax.Request(‘/data.json’, {
method: ‘get’,
onSuccess: function(response) {
var data = response.responseText;
// Process the response data
},
onFailure: function() {
// Handle errors
}
});
“`
In this example, we use Prototype.js to make an Ajax request to fetch data from the server. We specify the URL of the resource, the HTTP method to be used, and callbacks for handling the success and failure of the request. This demonstrates how Prototype.js simplifies the process of making asynchronous HTTP requests and handling response data.
Conclusion
Prototype.js is a powerful and versatile JavaScript framework that offers a wide range of features and functionality for web development. Its intuitive APIs, object-oriented nature, and comprehensive utilities make it a valuable tool for building modern web applications. By exploring the features and functionality of Prototype.js, developers can gain a deeper understanding of its capabilities and leverage its power to create rich and interactive user experiences.
FAQs
Q: What makes Prototype.js stand out among other JavaScript frameworks?
A: Prototype.js stands out for its object-oriented nature, comprehensive APIs for DOM manipulation, event handling, and Ajax requests, as well as its extensive utilities and helper methods. These features make it a valuable tool for streamlining the development process and creating dynamic and interactive web applications.
Q: Can Prototype.js be used in conjunction with other JavaScript libraries and frameworks?
A: Yes, Prototype.js can be used alongside other JavaScript libraries and frameworks. However, developers should be mindful of potential conflicts and ensure proper integration to avoid compatibility issues.
Q: Is Prototype.js suitable for building complex web applications?
A: Yes, Prototype.js is suitable for building complex web applications due to its object-oriented nature, rich set of APIs, and extensive utilities. It provides the flexibility and power needed to tackle the challenges of modern web development.