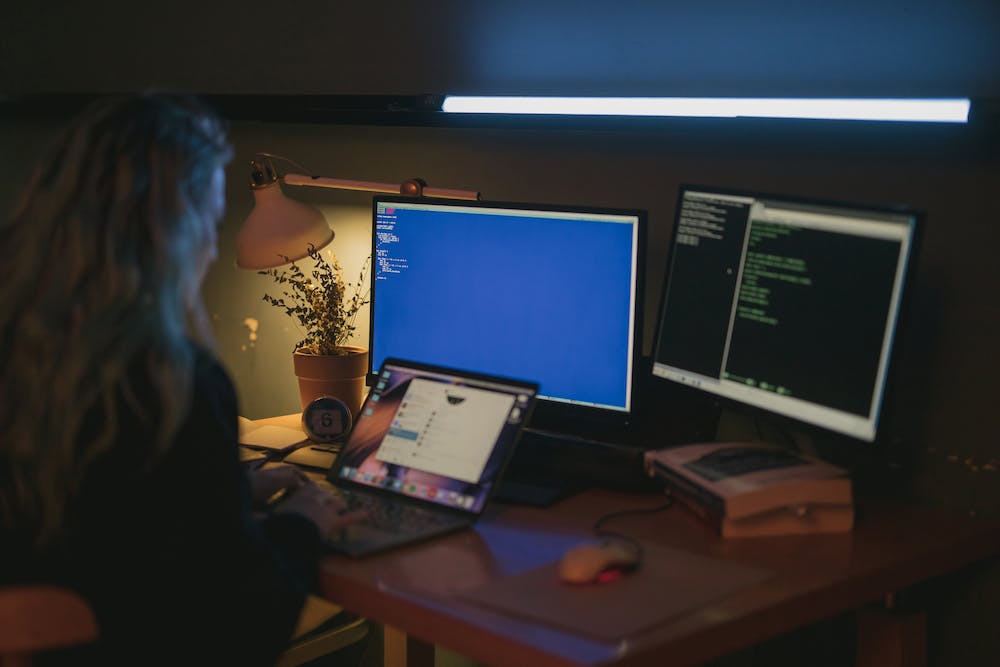
When working with PHP, there are various ways to exit a script. While the most common way is to use the exit()
function, there are other methods that can be employed for different scenarios. In this article, we will explore the different ways to exit a PHP script, along with their use cases, examples, and best practices.
The exit() Function
The exit()
function is the most straightforward way to terminate a PHP script. IT accepts an optional status code, which can be used to communicate the reason for exiting the script. Here’s a basic example of using exit()
:
// Perform some operations
if ($condition) {
exit("Error: Condition not met");
}
// Continue with the script
?>
In this example, if the condition is met, the script will exit with the provided error message. The status code can also be used for HTTP status responses, such as exit(404)
for a “Not Found” error.
die() Function
The die()
function is an alias of exit()
and can be used interchangeably. It is mainly used for error handling and can provide a more descriptive message when exiting the script. Here’s how to use die()
:
// Perform some operations
if ($error) {
die("Error: An error occurred");
}
// Continue with the script
?>
As with exit()
, the die()
function allows you to provide an error message and optionally a status code to communicate the reason for exiting the script.
Return Statement
In PHP, the return
statement is used to exit a function or a method. While it is not typically used to exit an entire script, it can be used to terminate the execution of a specific function or method. Here’s how to use return
:
function processData($data) {
if (!$data) {
return false;
}
// Process the data
}
?>
In this example, if the input data is empty, the processData
function will return false and exit, preventing the remaining code from executing.
Headers Sent
Another scenario that may require exiting a PHP script is when headers have already been sent to the client. This can occur if there is any output, including whitespace, before the header()
function is called. In such cases, the exit()
function can be used to terminate the script and prevent any further output. Here’s an example:
// Perform some operations
if ($headersSent) {
exit("Error: Headers already sent");
}
?>
The code checks if headers have already been sent and exits with an error message if they have, preventing any further output.
Best Practices
When it comes to exiting a PHP script, it’s essential to consider the context and the specific requirements of the application. Here are some best practices to keep in mind:
- Use
exit()
ordie()
for general script termination and error handling. - Use the
return
statement for exiting functions and methods. - Avoid exiting the script prematurely unless it’s necessary for error handling or flow control.
- Always provide meaningful error messages when using
exit()
ordie()
to assist with debugging and troubleshooting.
Conclusion
In conclusion, there are several ways to exit a PHP script, each with its own use cases and best practices. Whether it’s terminating the entire script, exiting a function, or handling errors, choosing the right method for exiting a script is crucial for the overall functionality and reliability of the application.
FAQs
What is the difference between exit() and die()?
exit()
and die()
are essentially the same and can be used interchangeably. They both terminate the script and accept an optional status code or error message.
Can I use return to exit a script?
The return
statement is primarily used to exit functions or methods, but it can be used to terminate the script if necessary, although it’s not a common practice.
When should I exit a PHP script?
You should exit a PHP script when you need to handle errors, terminate the execution under specific conditions, or prevent further output, such as when headers have already been sent.
Is it okay to use exit() for normal script termination?
While it’s okay to use exit()
for normal script termination in some cases, it’s generally best to allow the script to naturally reach the end of its execution. Using exit()
for normal termination can make the code less maintainable and harder to debug.