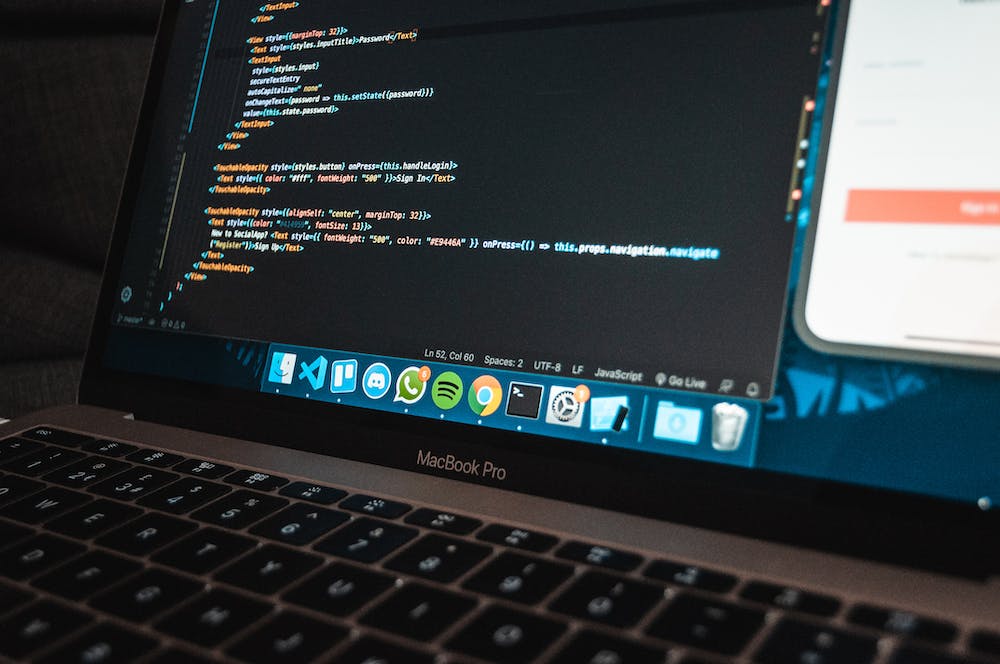
JavaScript is a versatile and powerful programming language that is widely used for web development. When IT comes to declaring variables in JavaScript, developers have three options: var, let, and const. Each of these options has its own unique characteristics and use cases, and it’s important for developers to understand the differences between them in order to write efficient and maintainable code.
The var keyword
The var keyword was the only way to declare variables in JavaScript before the introduction of let and const. Variables declared with var are function-scoped, meaning they are only accessible within the function in which they are declared.
One of the key features of var is that it allows for variable hoisting, which means that the variable declaration is moved to the top of the function or global scope. This can sometimes lead to unexpected behavior in the code, as the variable might be accessed before it is declared.
The let keyword
The let keyword was introduced in ECMAScript 6 (ES6) as a way to address some of the shortcomings of var. Variables declared with let are block-scoped, meaning they are only accessible within the block in which they are declared, whether it’s a loop, a conditional statement, or a function.
Unlike var, variables declared with let are not hoisted to the top of the block, and attempting to access them before they are declared will result in a ReferenceError. This makes the code more predictable and reduces the chances of encountering bugs related to variable hoisting.
The const keyword
The const keyword also made its debut in ES6, and it is used to declare constants, which are variables that cannot be re-assigned after they have been initialized. Like let, variables declared with const are block-scoped and not hoisted to the top of the block.
It’s important to note that while the value of a constant cannot be changed, if it is an object or array, the properties or elements of the object or array can still be modified. This can sometimes lead to confusion, so developers need to be mindful of this when using const.
Differences and use cases
Now that we have an understanding of the characteristics of var, let, and const, let’s explore their differences and use cases.
var vs let
One of the main differences between var and let is their scoping behavior. Variables declared with var are function-scoped, which means they are accessible throughout the entire function in which they are initialized. On the other hand, variables declared with let are block-scoped, meaning they are only accessible within the block in which they are defined.
In general, it is recommended to use let instead of var when declaring variables, as it helps to eliminate potential issues related to variable hoisting and makes the code more predictable. Additionally, the block-scoping behavior of let can help to reduce the chances of variable conflicts and unintended side effects in the code.
let vs const
While let and const are both block-scoped and do not hoist their declarations, the key difference between them is the ability to re-assign values. Variables declared with let can be re-assigned, while those declared with const cannot.
When it comes to choosing between let and const, it’s important to consider whether the value of the variable will need to be re-assigned. If the value will remain constant throughout the program, then it makes sense to use const to prevent accidental re-assignment. On the other hand, if the value needs to be updated or re-assigned, then let is the more appropriate choice.
Benefits of using let and const
By using let and const instead of var, developers can enjoy several benefits that contribute to the overall readability, predictability, and maintainability of the code.
Improved scoping behavior
The block-scoping behavior of let and const helps to minimize the risk of variable conflicts and unintended side effects in the code. This makes it easier to reason about the behavior of variables and reduces the potential for bugs related to variable hoisting.
Prevention of accidental re-assignment
By using const for variables that should not be re-assigned, developers can prevent accidental re-assignment and make the code more resistant to errors and unintended modifications. This can be particularly helpful when working with constants such as mathematical or configuration values.
Conclusion
In conclusion, the differences between var, let, and const in JavaScript are significant and have important implications for the readability, predictability, and maintainability of the code. By understanding the unique characteristics and use cases of each keyword, developers can make informed decisions about which one to use in different scenarios, ultimately leading to more robust and bug-free code.
FAQs
Q: Can I re-declare a variable declared with let or const?
A: No, attempting to re-declare a variable declared with let or const will result in a SyntaxError. This helps to prevent accidental re-declaration and contributes to the predictability of the code.
Q: When should I use var instead of let or const?
A: In general, it is recommended to use let or const instead of var in modern JavaScript code. However, if you need to support older browsers or if you specifically want the variable to be function-scoped and hoisted, then var might still be a viable option.
Q: Can I use let and const in Node.js?
A: Yes, both let and const are fully supported in Node.js, as long as you are using a version that supports ES6 (or later) features. It’s generally a good idea to use let and const in Node.js to take advantage of their benefits and improve the quality of your code.
Q: Are there any performance differences between var, let, and const?
A: In terms of performance, there are no significant differences between var, let, and const. The choice between them should be based on their scoping behavior and the intended use of the variables, rather than performance considerations.