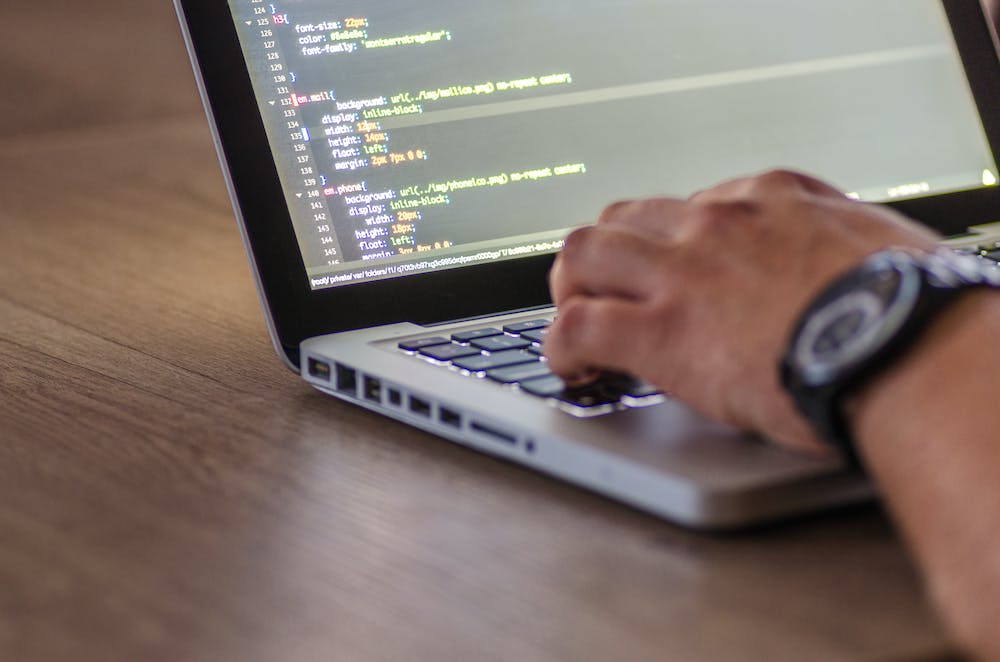
In the world of computer science, understanding the fundamental principles of structure and interpretation in computer programs is crucial. These building blocks form the foundation upon which all software applications and systems are constructed. By delving into the underlying concepts of structure and interpretation, we can gain a deeper understanding of how programs are designed, executed, and interpreted by computers.
The Importance of Structure and Interpretation in Computer Programs
Structure and interpretation are two key components that define the behavior and functionality of computer programs. Structure refers to the organization and arrangement of the various elements within a program, such as statements, functions, and data. IT determines how the program is organized and how its components interact with each other.
Interpretation, on the other hand, involves the process of understanding and executing the instructions defined within a program. It encompasses the translation of high-level programming languages into machine code that can be executed by a computer. Interpretation also involves the evaluation of program behavior and output based on the input provided.
Understanding the Building Blocks: Structure
When it comes to the structure of a computer program, there are several fundamental concepts that play a crucial role in its design and implementation. These include:
Data Types and Structures
Data types and structures form the building blocks of any program. They define the various types of data that can be used within a program, such as integers, floating-point numbers, characters, and strings. Data structures, on the other hand, define how data is organized and stored within a program, such as arrays, lists, and dictionaries.
Control Structures
Control structures determine the flow of execution within a program. They include constructs such as loops, conditionals, and branching statements, which allow the program to make decisions and execute specific blocks of code based on certain conditions.
Functions and Procedures
Functions and procedures encapsulate a set of instructions that can be reused and invoked multiple times within a program. They help to modularize the program and make it more maintainable, as well as improve code reusability and readability.
Understanding the Building Blocks: Interpretation
Interpretation in computer programs involves the process of translating and executing the instructions defined within a program. This encompasses several key concepts, including:
Compilation vs. Interpretation
There are two primary methods for translating high-level programming languages into machine code: compilation and interpretation. Compilation involves translating the entire program into machine code before execution, while interpretation involves translating and executing the program line by line.
Execution Models
There are several execution models that dictate how programs are executed and interpreted by a computer. These include stack-based, register-based, and bytecode interpreters, each of which has its own advantages and limitations.
Runtime Environments
Runtime environments provide the necessary infrastructure and resources for executing and interpreting programs. These environments include memory management, input/output operations, and system libraries that are essential for program execution.
Examples of Structure and Interpretation in Computer Programs
Let’s consider a simple example to illustrate the concepts of structure and interpretation in computer programs. Suppose we have a basic program that calculates the factorial of a given number:
“`python
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
“`
In this example, the structure of the program consists of a function called `factorial` that uses a conditional statement to calculate the factorial of a given number. The interpretation of the program involves executing the instructions defined within the function, recursively calling the `factorial` function until a base case is reached.
Conclusion
Understanding the building blocks of structure and interpretation in computer programs is essential for anyone involved in software development and computer science. These fundamental concepts form the backbone of all programming languages and systems, and they play a crucial role in determining the behavior and functionality of computer programs. By exploring the concepts of structure and interpretation, we can gain a deeper understanding of how programs are designed, executed, and interpreted by computers, ultimately leading to more efficient and effective software development practices.
FAQs
What are the building blocks of structure in computer programs?
The building blocks of structure in computer programs include data types and structures, control structures, and functions/procedures.
What is the role of interpretation in computer programs?
Interpretation in computer programs involves the process of translating and executing the instructions defined within a program, allowing the program to be executed by a computer.
How do structure and interpretation impact software development practices?
Structure and interpretation play a crucial role in determining the behavior and functionality of computer programs, ultimately leading to more efficient and effective software development practices.
Are there different execution models for interpreting computer programs?
Yes, there are several execution models, including stack-based, register-based, and bytecode interpreters, each of which has its own advantages and limitations.
How can I learn more about structure and interpretation in computer programs?
There are several resources available, including textbooks, online courses, and tutorials, that can provide a deeper understanding of structure and interpretation in computer programs.