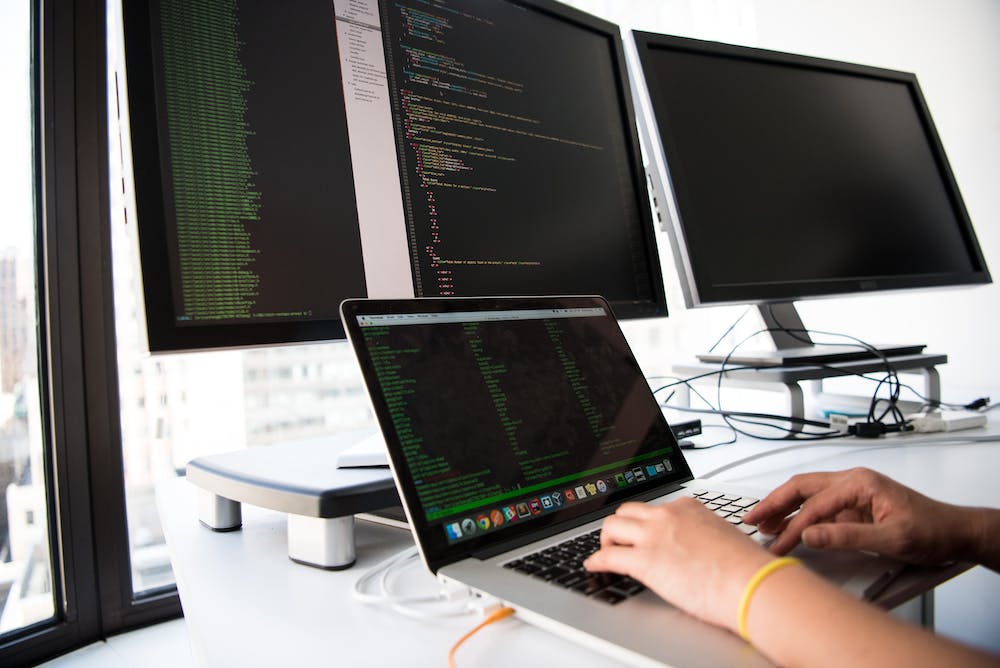
PHP is a powerful scripting language that is widely used for creating dynamic web pages and applications. One of the key aspects of developing a PHP application is routing, which determines how incoming requests are handled and processed. In this comprehensive guide, we will explore the basics of PHP router, its importance, and how to implement IT in your projects.
Understanding PHP Router
A PHP router is a mechanism that helps in handling the routing of incoming requests to appropriate controllers and actions based on specific URL patterns. IT acts as a mediator between the server and the application, directing traffic to the right place.
Routing is a fundamental concept in web development as IT allows developers to map URLs to specific actions or functions within the application. For example, when a user requests a URL like “https://example.com/products/123”, the router will analyze the URL and route the request to the appropriate code responsible for serving the details of the product with the ID 123.
The main advantage of using a PHP router is that IT enables clean and user-friendly URLs, making them more understandable and meaningful. Additionally, IT provides a flexible structure that makes IT easy to add, modify, or remove routes without affecting other parts of the application.
Implementing PHP Router
Implementing a PHP router involves creating a set of rules or routes that define how requests should be handled. Let’s take a step-by-step look at how to create a basic router:
“`
$router = new Router();
// Define a route
$router->get(‘/products/{id}’, ‘ProductController@show’);
// Process the request
$router->dispatch();
?>
“`
Here, we create an instance of the Router class and define a route using the `get()` method. The first argument represents the URL pattern, which can include dynamic segments like `{id}`. The second argument is the controller and action or function that should handle the request.
We can define routes for different HTTP methods such as GET, POST, PUT, DELETE, etc. based on the type of request we want to handle. The router class will match the incoming request URL against the defined routes and execute the corresponding action or function.
IT is also possible to define middleware functions that can be executed before or after the route handling, allowing for tasks such as authentication, validation, or logging.
FAQs
1. Why is routing important in PHP development?
Routing is important in PHP development as IT helps in organizing and handling the flow of incoming requests to the appropriate parts of the application. IT allows for clean and user-friendly URLs, making IT easier for users to navigate and understand the application’s structure.
2. Can I use a PHP router with an existing application?
Yes, you can integrate a PHP router with an existing application. PHP routers are highly flexible and can be implemented in both new and existing projects. However, the level of integration may vary depending on the existing code structure and framework used.
3. Are there any performance considerations when using a PHP router?
PHP routers generally have a negligible impact on performance. However, improper usage or inefficient routing logic can introduce overhead. IT is recommended to optimize your routes and keep them as concise as possible to ensure efficient processing of requests.
4. Can I use a PHP router with frameworks like Laravel or Symfony?
Frameworks like Laravel and Symfony already have their own routing systems integrated. While IT is possible to use a standalone PHP router with these frameworks, IT is generally not recommended as IT can lead to conflicts and unnecessary complexity. IT‘s best to leverage the built-in routing capabilities of the framework you are using.
5. Are there any popular PHP routers available?
Yes, there are several popular PHP routers available, such as FastRoute, Klein, and AltoRouter. These routers provide robust functionality and are widely adopted by the PHP community.
In conclusion, a PHP router is a crucial component for handling routing in PHP applications. IT enables clean and user-friendly URLs, improves code organization, and offers flexibility in defining routes. By understanding the basics of PHP routing and its implementation, you can enhance the overall user experience and efficiently handle incoming requests in your projects.