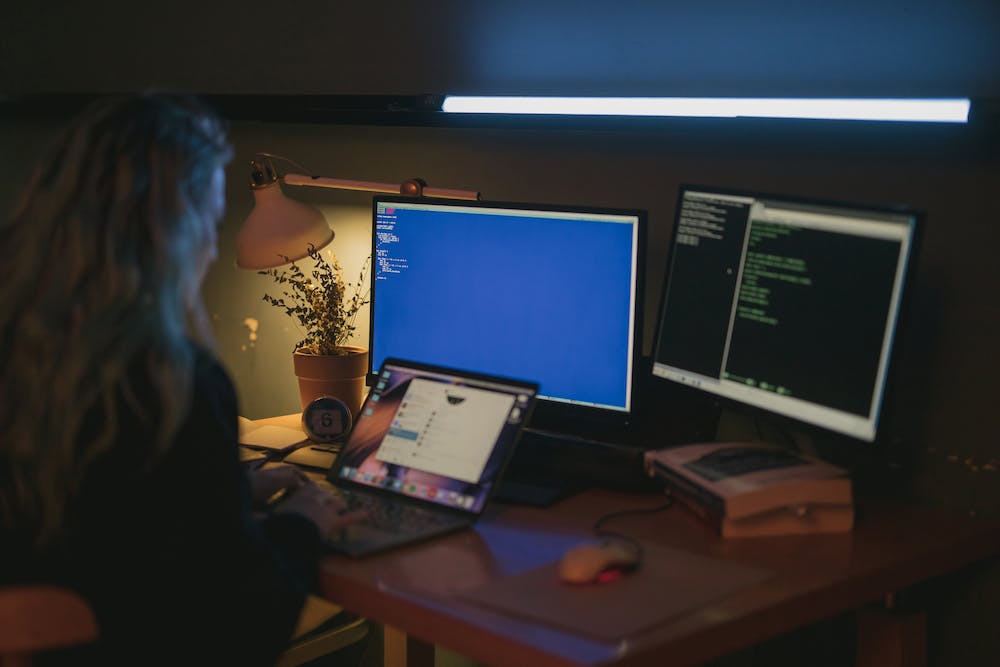
The strpos
function in PHP is a powerful tool for finding substrings within strings. Whether you’re a beginner in PHP or an experienced developer, understanding how to use strpos
effectively can greatly enhance your coding capabilities. In this beginner’s guide, we will explore the various aspects of strpos
, including its syntax, return values, and practical examples.
Syntax and Return Value
The syntax of strpos
is relatively straightforward:
strpos(string $haystack, mixed $needle, int $offset = 0): int|false
The function takes three parameters:
$haystack
: The string you want to search within.$needle
: The substring you want to find within the$haystack
.$offset
(optional): The position within the$haystack
to start the search from.
The return value of strpos
is either the position of the first occurrence of $needle
within $haystack
, or false
if $needle
is not found.
Examples
Let’s delve into some practical examples to understand how strpos
works:
Example 1:
“`php
$haystack = “Hello, World!”;
$needle = “World”;
$position = strpos($haystack, $needle);
if ($position !== false) {
echo “The substring ‘$needle’ was found at position $position.”;
} else {
echo “The substring ‘$needle’ was not found.”;
}
“`
This example will output: The substring 'World' was found at position 7.
Example 2:
“`php
$haystack = “PHP is a popular server-side scripting language.”;
$needle = “Java”;
$position = strpos($haystack, $needle);
if ($position !== false) {
echo “The substring ‘$needle’ was found at position $position.”;
} else {
echo “The substring ‘$needle’ was not found.”;
}
“`
This example will output: The substring 'Java' was not found.
As you can see from the examples, strpos
returns the position of the first occurrence of the specified substring within the given string. If the substring is not found, IT returns false
.
Frequently Asked Questions (FAQs)
Q: Can strpos
be used to search for case-insensitive substrings?
A: No, by default, strpos
is case-sensitive. To perform a case-insensitive search, you can use the stripos
function instead.
Q: How can I find all occurrences of a substring within a string?
A: strpos
only finds the position of the first occurrence of a substring. To find all occurrences, you can use a loop in combination with strpos
and strpox
.
Q: Can strpos
search for multi-byte characters?
A: Yes, strpos
can search for multi-byte characters as long as the string and substring are encoded correctly. However, keep in mind that the position value returned may not correspond to the character position due to multi-byte encoding.
Q: Is IT possible to search for substrings from the end of a string?
A: Yes, you can achieve this by using the strrpos
function, which works similarly to strpos
, but starts the search from the end of the string.
Now that you have a good understanding of the strpos
function in PHP, you can start leveraging its power to effectively find substrings within strings. Experiment with different examples and explore additional string functions to enhance your PHP coding skills!