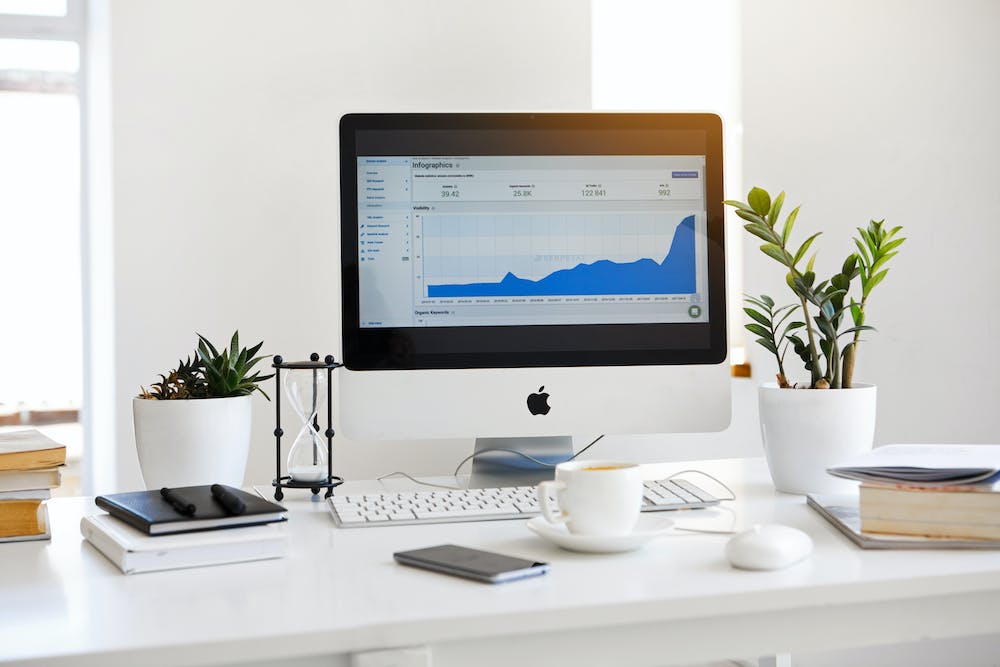
PHP is a powerful server-side scripting language that is widely used for web development. One of the many functions that PHP offers is the ability to convert strings into arrays. This can be incredibly useful when working with data from forms, databases, or APIs. In this article, we will explore some of the most commonly used PHP functions for converting strings into arrays, along with examples to help you understand how to use them effectively.
Exploring the explode() Function
The explode() function is probably the most commonly used function for converting strings into arrays in PHP. IT takes a delimiter and a string as input and returns an array of substrings. The delimiter is the character or characters that separate the substrings. Here’s a simple example:
“`php
$str = “apple,banana,orange”;
$arr = explode(“,”, $str);
print_r($arr);
“`
In this example, the explode() function takes the string “apple,banana,orange” and splits it into an array using the comma as the delimiter. The resulting array would look like this:
“`php
Array
(
[0] => apple
[1] => banana
[2] => orange
)
“`
Using the preg_split() Function for Advanced String Parsing
Another function that can be used to convert strings into arrays is preg_split(). This function takes a regular expression pattern and a string as input and returns an array of substrings. This makes it a powerful tool for more complex string parsing. Here’s an example:
“`php
$str = “apple,banana,orange”;
$arr = preg_split(“/,/”, $str);
print_r($arr);
“`
In this example, we are using preg_split() with the regular expression pattern “/,/” to split the string using the comma as the delimiter. The resulting array would be the same as the previous example.
Converting Strings with Fixed Widths into Arrays
Sometimes, you may need to convert a string with fixed widths into an array of substrings. This can be accomplished using the str_split() function, which takes a string and a length as input and returns an array of chunks. Here’s an example:
“`php
$str = “apple banana orange”;
$arr = str_split($str, 6);
print_r($arr);
“`
In this example, the str_split() function takes the string “apple banana orange” and splits it into an array of chunks with a length of 6 characters. The resulting array would look like this:
“`php
Array
(
[0] => apple
[1] => banana
[2] => orange
)
“`
Conclusion
PHP provides several powerful functions for converting strings into arrays, including explode(), preg_split(), and str_split(). These functions can be incredibly useful when working with data in your PHP applications, allowing you to easily separate and manipulate strings as needed. By understanding how to use these functions effectively, you can take full advantage of the capabilities of PHP and improve the efficiency of your web development projects.
FAQs
What is the main difference between explode() and preg_split()?
The main difference between explode() and preg_split() is that explode() uses a simple string as the delimiter, while preg_split() allows you to use a regular expression pattern as the delimiter. This makes preg_split() more powerful for more complex string parsing.
Can I use these functions with multi-byte characters?
Yes, these functions support multi-byte characters, but you need to be careful when working with multi-byte encodings to ensure proper string manipulation.
Are there any limitations to using str_split() for converting strings into arrays?
One limitation of str_split() is that it does not take into account multi-byte characters, so it may not work as expected with multi-byte encodings. In such cases, you may need to use a different approach for splitting strings with fixed widths.
Can I use these functions to convert strings from other sources, such as files or APIs?
Yes, you can use these functions to convert strings from a variety of sources, including files, databases, and APIs. As long as you have a string to work with, these functions can be used to convert it into an array for further manipulation.