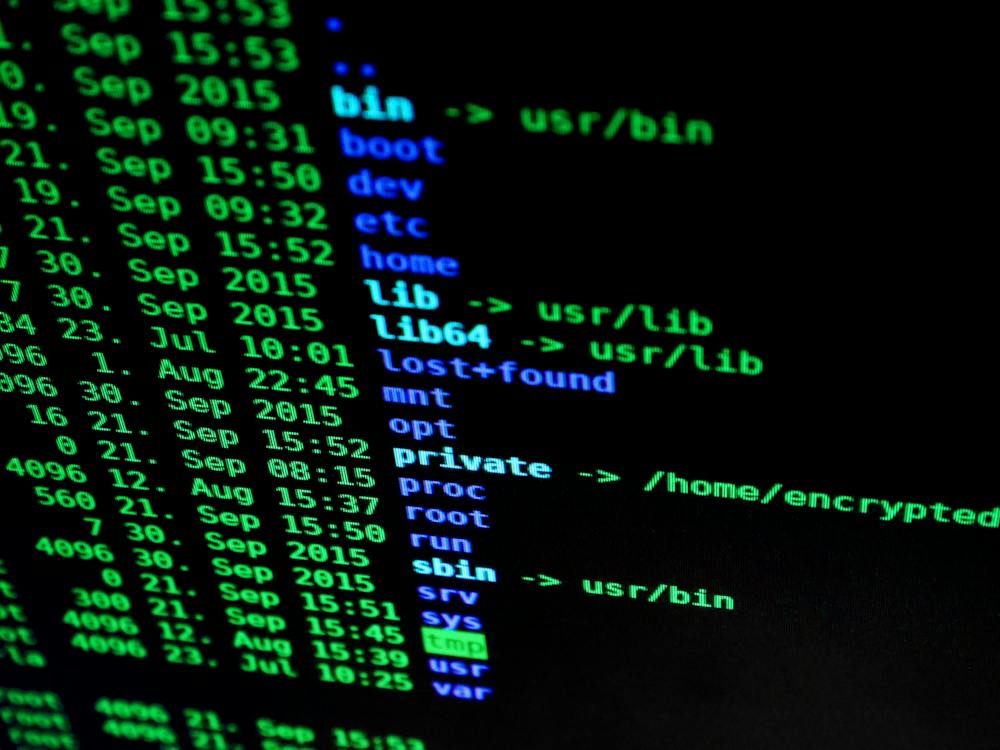
PHP is a popular server-side scripting language used for web development. IT provides a wide range of data types and variables to handle different types of data. Understanding these data types and variables is crucial for writing efficient and error-free PHP code.
PHP Data Types
PHP supports various data types, including:
- Integer: Represents whole numbers without any decimal points.
- Float: Represents numbers with decimal points.
- String: Represents a sequence of characters.
- Boolean: Represents true or false values.
- Array: Represents a collection of elements.
- Object: Represents an instance of a class.
- NULL: Represents a variable with no value.
Examples:
Here are some examples of using different data types in PHP:
$integerVar = 10;
$floatVar = 3.14;
$stringVar = "Hello, world!";
$boolVar = true;
$arrayVar = array(1, 2, 3, 4, 5);
$objectVar = new stdClass();
$nullVar = NULL;
PHP Variables
In PHP, variables are used to store data values. Variable names must start with a dollar sign ($) followed by the name of the variable. They are case-sensitive and must begin with a letter or an underscore.
Variable Scope:
PHP variables have different scopes:
- Local: Variables declared within a function have local scope and can only be accessed inside that function.
- Global: Variables declared outside of any function have global scope and can be accessed from anywhere in the PHP script.
- Static: Static variables retain their values between function calls and have function scope.
Examples:
Here are some examples of using variables in PHP:
$globalVar = "I am a global variable.";
function testFunction() {
$localVar = "I am a local variable.";
static $staticVar = 0;
$staticVar++;
echo "Global Variable: " . $GLOBALS['globalVar'] . "
";
echo "Local Variable: " . $localVar . "
";
echo "Static Variable: " . $staticVar . "
";
}
testFunction();
testFunction();
The above example demonstrates the usage of global, local, and static variables in PHP.
Conclusion
Understanding PHP data types and variables is essential for writing robust and efficient code. By leveraging the appropriate data types and variables, developers can ensure that their PHP applications are optimized for performance and reliability. Whether IT‘s handling numeric values, text data, or complex data structures, PHP offers a rich set of data types and variables to cater to diverse programming needs.
FAQs
Q: What is the difference between single quotes and double quotes for defining strings in PHP?
A: In PHP, single quotes and double quotes can be used to define strings. The key difference is that variables and escape sequences inside double-quoted strings are interpreted, whereas they are treated literally in single-quoted strings.
Q: Can PHP variables store multiple values?
A: Yes, PHP provides the array data type to store multiple values in a single variable. Arrays can hold a collection of elements, which can be accessed and manipulated using various built-in array functions in PHP.
Q: What is the purpose of the NULL data type in PHP?
A: The NULL data type in PHP represents a variable with no value. IT can be used to initialize a variable without assigning any specific value to IT, or to reset a variable’s value to NULL after IT has been used.
Q: How can I declare a constant variable in PHP?
A: In PHP, you can use the define()
function to declare a constant variable. Constants are similar to variables, but their values cannot be changed once they are defined.
Q: What is variable scope in PHP?
A: Variable scope in PHP refers to the accessibility of variables within different parts of a PHP script. Variables can have local, global, or static scope, which determines where they can be accessed and modified.