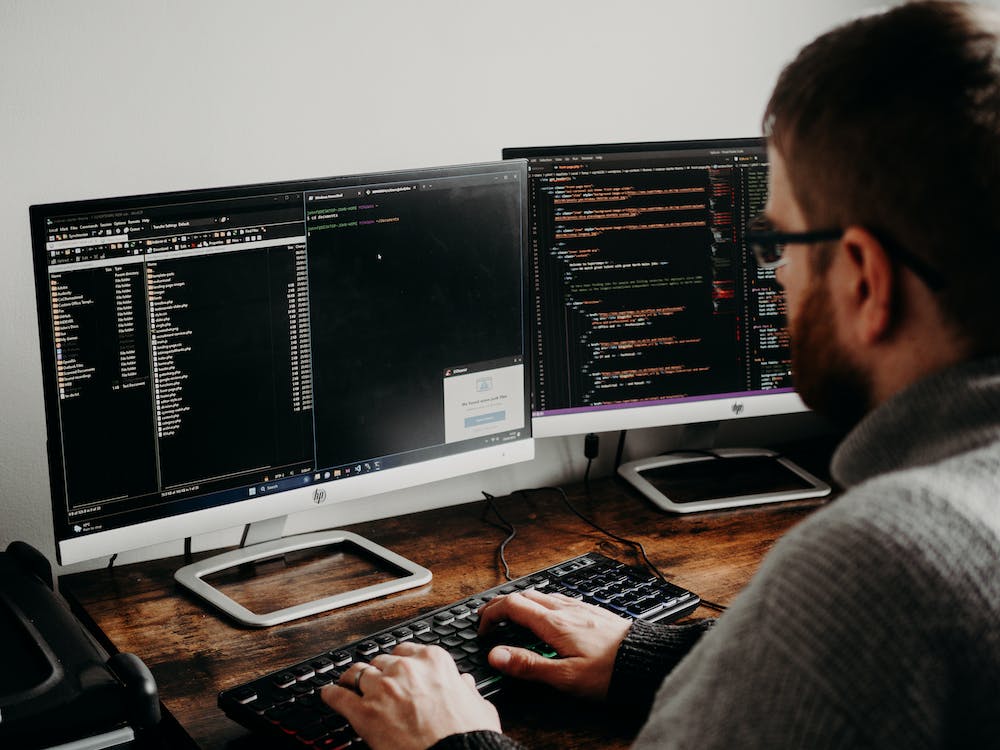
PHP is a widely-used open-source scripting language that is especially suited for web development and can be embedded into HTML. One of the fundamental data structures in PHP is the array, and fortunately, PHP provides a plethora of array functions to manipulate, iterate, and extract data from arrays. In this comprehensive guide, we will explore some of the most commonly used PHP array functions and provide examples to illustrate their usage.
1. array_push()
The array_push() function is used to add one or more elements to the end of an array. IT modifies the original array and returns the new number of elements in the array.
$fruits = array("apple", "banana", "orange");
array_push($fruits, "grape", "watermelon");
print_r($fruits);
?>
In the above example, the array_push() function adds “grape” and “watermelon” to the end of the $fruits array.
2. array_pop()
The array_pop() function removes the last element from the array and returns IT. The original array is modified as a result.
$fruits = array("apple", "banana", "orange");
$lastFruit = array_pop($fruits);
echo $lastFruit; // outputs "orange"
?>
In this example, the array_pop() function removes “orange” from the $fruits array and returns IT.
3. array_merge()
The array_merge() function is used to merge two or more arrays into a single array. The resulting array contains the elements of all the arrays that are passed as arguments.
$fruits1 = array("apple", "banana");
$fruits2 = array("orange", "grape");
$combinedFruits = array_merge($fruits1, $fruits2);
print_r($combinedFruits);
?>
The array_merge() function in this example combines the $fruits1 and $fruits2 arrays into a single $combinedFruits array.
4. array_search()
The array_search() function is used to search for a specific value in an array. If the value is found, the function returns the corresponding key; otherwise, IT returns false.
$fruits = array("apple", "banana", "orange");
$index = array_search("banana", $fruits);
echo $index; // outputs 1
?>
In this example, the array_search() function returns the index of “banana” in the $fruits array.
5. array_column()
The array_column() function is used to extract a single column from a multi-dimensional array. IT returns an array containing the values from a single column.
$products = array(
array("id" => 1, "name" => "Product 1", "price" => 100),
array("id" => 2, "name" => "Product 2", "price" => 200),
array("id" => 3, "name" => "Product 3", "price" => 300)
);
$productNames = array_column($products, "name");
print_r($productNames);
?>
The array_column() function in this example returns an array containing the names of the products from the $products array.
Conclusion
PHP array functions are essential for manipulating arrays and extracting data efficiently. In this comprehensive guide, we have explored some of the most commonly used array functions in PHP, along with examples illustrating their usage. By mastering these functions, you can work with arrays more effectively and write cleaner, more maintainable code.
FAQs
Q: Are PHP array functions case-sensitive?
A: Yes, most PHP array functions are case-sensitive. For example, in array_search(), the value being searched for must have the exact same case as the elements in the array.
Q: Can array functions be used with associative arrays?
A: Yes, array functions can be used with both indexed and associative arrays. However, some functions may behave differently with associative arrays, so IT‘s important to consult the PHP documentation for specific details.
Q: Are there any array functions to sort arrays?
A: Yes, PHP provides array sorting functions such as sort(), rsort(), asort(), ksort(), and more. These functions can be used to sort arrays in ascending or descending order based on their values or keys.