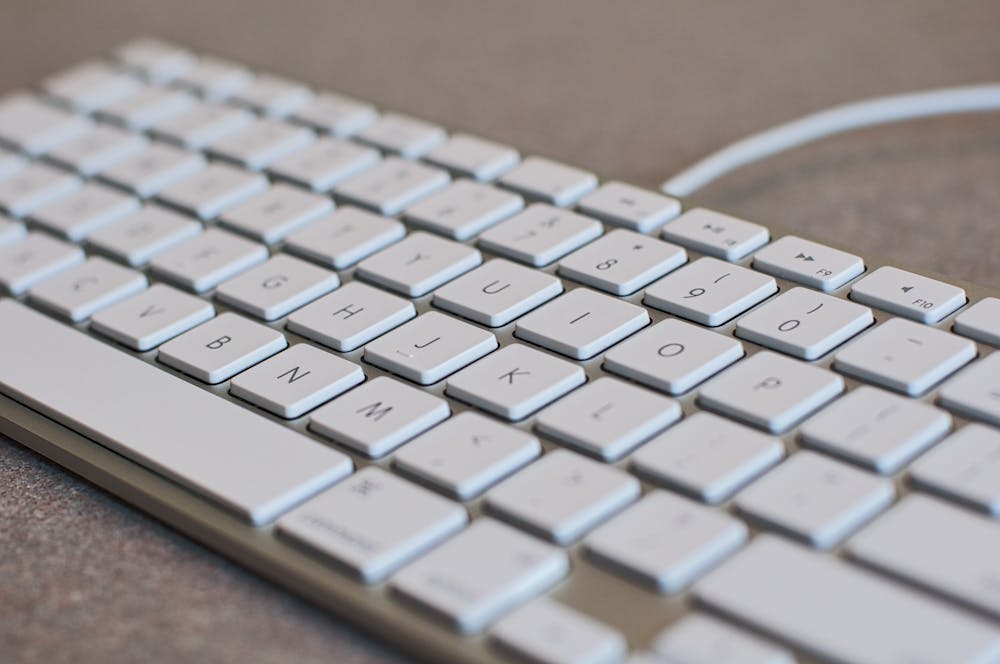
Laravel is a popular PHP framework that provides a wide range of features to simplify web development. One of its key features is session management, which allows developers to maintain stateful information across multiple requests. In this comprehensive guide, we will explore how Laravel handles session management and the various techniques and functionalities IT offers. Whether you are new to Laravel or an experienced developer, this article will provide a detailed understanding of Laravel session management.
Introduction to Laravel Session Management
Laravel session management is the process of handling and maintaining user state information across multiple HTTP requests. IT allows developers to store and retrieve data for individual users between requests, ensuring a seamless and personalized browsing experience. Laravel provides a reliable, secure, and straightforward approach to session management, making IT an ideal choice for web application development.
Usage of Laravel Session
Laravel sessions can be used for various purposes, including:
- User authentication and authorization
- Remembering user preferences
- Shopping cart functionality
- Flash messages for displaying temporary notifications
Understanding how to utilize Laravel session management effectively is crucial for building dynamic and interactive web applications.
Configuring Session Driver
Laravel offers multiple session drivers to choose from, including file, cookie, database, and more. You can configure the desired driver in the config/session.php
file present in your Laravel project. By default, Laravel uses the file
driver, which stores session data in the server’s file system. However, you can easily switch to other drivers based on your requirements and preferences.
Storing Data in Sessions
Laravel provides a simple and intuitive approach to store and retrieve data in sessions. You can use the global session()
helper function to interact with the session. For example, to store a value in the session, you can use the put()
method:
session()->put('key', 'value');
Similarly, you can retrieve a value from the session using the get()
method:
$value = session()->get('key');
Retrieving and Removing Data
You can retrieve and remove session data using various methods provided by Laravel. The all()
method retrieves all data from the session:
$data = session()->all();
You can remove an item from the session using the forget()
method:
session()->forget('key');
Flashing Data in Laravel Sessions
Laravel allows you to flash data to the session, which is available only for the next request. This is useful to display temporary messages or notifications. You can flash data using the flash()
method:
session()->flash('message', 'Your message here');
The flashed data will be available for the next request, and automatically cleared afterwards.
FAQs
1. How long does Laravel session data last?
The duration of Laravel session data can vary depending on the configuration. By default, Laravel session data lasts until the user closes the browser. However, you can configure the session expiration time in the config/session.php
file.
2. Can Laravel sessions work across multiple domains?
No, Laravel sessions are bound to a single domain. They cannot be shared across multiple domains due to security restrictions imposed by web browsers.
3. How can I secure Laravel sessions?
Laravel supports various options for securing sessions, such as using the https
protocol, encrypting session data, and configuring the same_site
option. You can refer to the Laravel documentation for more information on securing your sessions.
4. How can I test Laravel sessions in my applications?
Laravel provides a convenient way to test session-related functionality using the built-in testing suite. You can create tests to simulate session interactions and ensure the expected behavior of your application.
5. Can I use custom session drivers in Laravel?
Yes, Laravel allows you to create custom session drivers if the built-in drivers do not meet your requirements. You can find detailed instructions on creating custom session drivers in the Laravel documentation.
By now, you should have a comprehensive understanding of Laravel session management. IT is a powerful feature that enables developers to build interactive and dynamic web applications. Laravel’s session management capabilities provide a seamless experience for users while ensuring the security and reliability of their data.