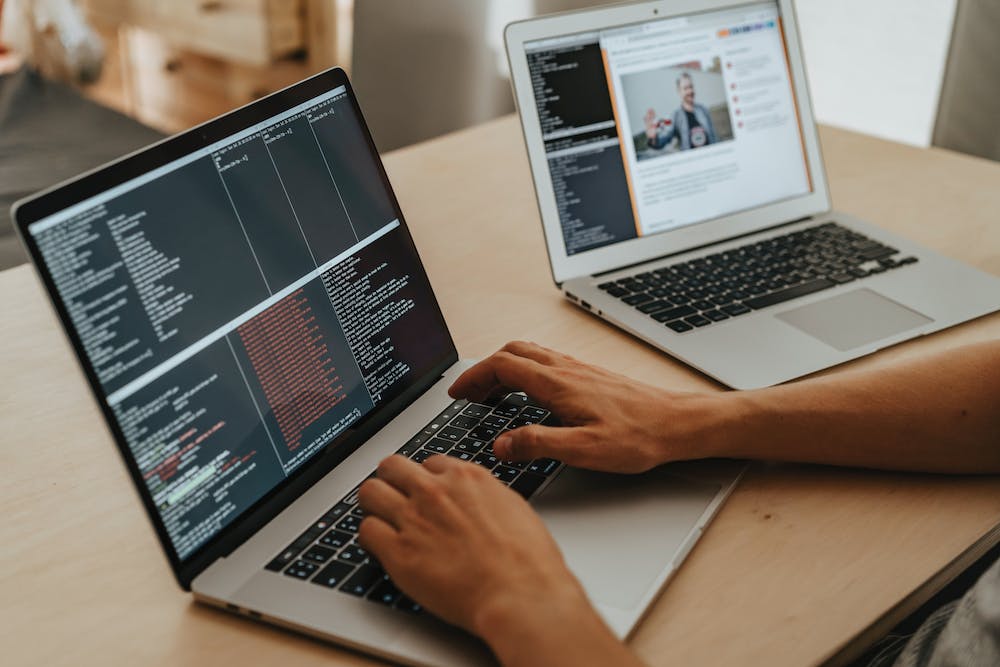
JavaScript is a versatile and powerful programming language that is widely used for creating interactive and dynamic websites. One of the key features of JavaScript is its ability to manipulate arrays and objects efficiently. In this comprehensive guide, we will explore the find
feature in JavaScript, which is a handy method for searching and retrieving specific elements from an array. We will cover the syntax, usage, and examples of the find
method to help you understand how to use IT effectively in your projects.
Understanding the Find Method in JavaScript
The find
method is available for arrays in JavaScript and is used to retrieve the first element in the array that satisfies a specified condition. IT provides a concise and readable way to search for an item based on a given criterion. The syntax of the find
method is as follows:
array.find(callback(element[, index, [array]])[, thisArg])
Here, the callback
function is the condition that each element of the array is tested against. IT should return true
for the first matching element, and false
otherwise. The callback
function can take up to three arguments: element
(the current element being processed), index
(the index of the current element), and array
(the array that find
was called upon). The optional thisArg
parameter can be provided to set the value of this
when executing the callback
function.
When the find
method is called on an array, IT iterates through each element and applies the callback
function to IT. As soon as the callback
function returns true
for an element, the find
method stops and returns that element. If no element satisfies the condition, undefined
is returned.
Using the Find Method in JavaScript
Let’s take a look at an example to understand how the find
method can be used in JavaScript. Suppose we have an array of objects representing products, and we want to find the first product with a specific name. We can use the find
method to achieve this as shown below:
const products = [
{ id: 1, name: 'Laptop', price: 1200 },
{ id: 2, name: 'Smartphone', price: 800 },
{ id: 3, name: 'Tablet', price: 500 }
];
const productName = 'Smartphone';
const foundProduct = products.find(product => product.name === productName);
console.log(foundProduct); // Output: { id: 2, name: 'Smartphone', price: 800 }
In this example, we define an array of products
and use the find
method to search for the product with the name ‘Smartphone’. The callback
function checks each product’s name and returns true
when a match is found. The foundProduct
variable then stores the first matching product. As a result, the foundProduct
will contain the object representing the ‘Smartphone’ product.
Benefits of Using the Find Method
The find
method offers several benefits when working with arrays in JavaScript:
- Concise and Readable Code: The
find
method provides an elegant way to express the search criteria, making the code more readable and maintainable. - Efficient Searching: The
find
method stops as soon as IT finds the first matching element, which can be more efficient than iterating through the entire array. - Flexibility: The
callback
function used with thefind
method can be customized to match various conditions, giving you the flexibility to search for specific elements based on different criteria.
Conclusion
The find
method in JavaScript provides a powerful and efficient way to search for elements in an array based on a specified condition. By understanding its syntax, usage, and benefits, you can leverage the find
method to streamline your code and enhance the functionality of your JavaScript applications. Whether you are working with arrays of objects or simple arrays, the find
method can simplify your search operations and improve the overall performance of your code.
FAQs
Q: Can the find method be used for nested arrays or multi-dimensional arrays?
A: Yes, the find
method can be used to search for elements in nested arrays or multi-dimensional arrays. When writing the callback
function, you can access nested properties or elements within the array to define your search criteria.
Q: Does the find method modify the original array?
A: No, the find
method does not modify the original array. IT only retrieves the first matching element based on the specified condition without changing the original array.
Q: What is the difference between find and filter in JavaScript?
A: The main difference is that the find
method returns the first matching element, while the filter
method returns an array of all elements that satisfy the specified condition.