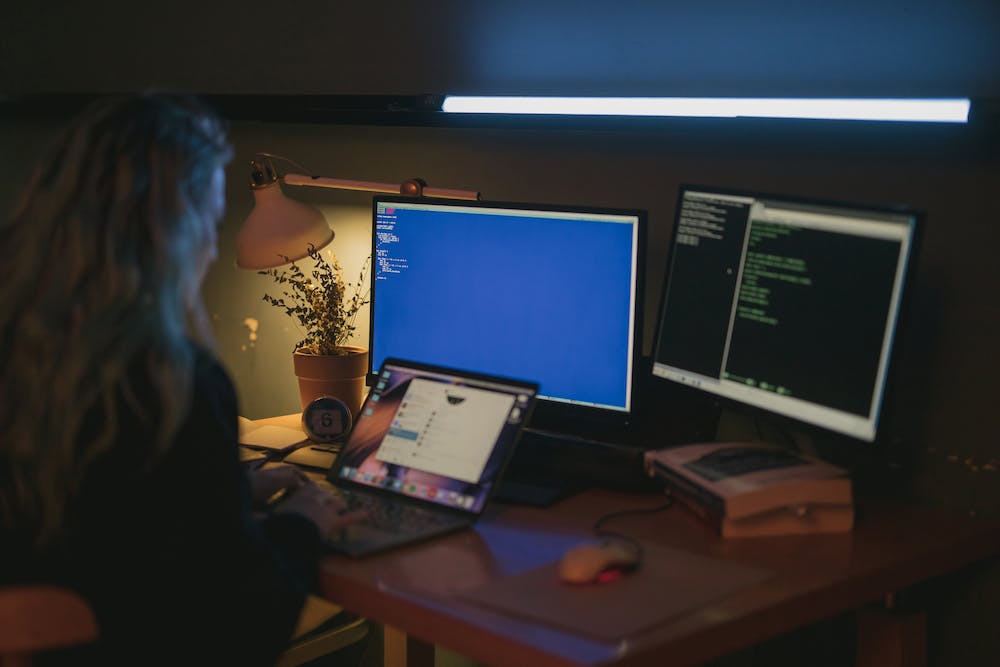
PHP is a popular scripting language used for web development. IT offers various built-in functions for manipulating data, including string manipulation. One common task in PHP development is converting a string into an array. There are several techniques and functions available in PHP to accomplish this task. In this article, we will explore different techniques for converting a string to an array in PHP.
Using explode() Function
The explode() function is one of the most commonly used functions for converting a string into an array in PHP. It takes a delimiter and a string as input and returns an array of strings based on the delimiter. Here’s an example of how to use the explode() function:
$str = "apple,banana,orange";
$arr = explode(",", $str);
print_r($arr);
In this example, the string “apple,banana,orange” is converted into an array with three elements: “apple”, “banana”, and “orange”. The comma (,) is used as the delimiter to split the string.
Using str_split() Function
The str_split() function is another option for converting a string into an array in PHP. It breaks a string into an array of characters. Here’s an example of how to use the str_split() function:
$str = "hello";
$arr = str_split($str);
print_r($arr);
In this example, the string “hello” is converted into an array with five elements, each representing a character in the string: “h”, “e”, “l”, “l”, and “o”.
Using preg_split() Function
The preg_split() function allows you to use a regular expression as a delimiter for splitting a string into an array. This can be useful for more complex string manipulation. Here’s an example of how to use the preg_split() function:
$str = "apple,banana;orange";
$arr = preg_split("/[,;]/", $str);
print_r($arr);
In this example, the string “apple,banana;orange” is split into an array using the regular expression “/[,;]/”, which matches both commas and semicolons as delimiters. The resulting array contains three elements: “apple”, “banana”, and “orange”.
Using StringTokenizer Class
The PHP StringTokenizer class provides a way to tokenize a string into an array of tokens based on a set of delimiters. Here’s an example of how to use the StringTokenizer class:
$str = "apple,banana;orange";
$tokenizer = new \StringTokenizer($str, ",;");
$arr = [];
while ($tokenizer->hasMoreTokens()) {
$arr[] = $tokenizer->nextToken();
}
print_r($arr);
In this example, the string “apple,banana;orange” is tokenized using the delimiters “,” and “;”, resulting in an array with three elements: “apple”, “banana”, and “orange”.
Using Regular Expression
Regular expressions can also be used to split a string into an array. The preg_match_all() function can be used to achieve this. Here’s an example:
$str = "apple,banana;orange";
preg_match_all('/\w+/', $str, $arr);
print_r($arr[0]);
In this example, the regular expression ‘\w+’ matches one or more word characters, effectively splitting the string into an array of words. The resulting array contains three elements: “apple”, “banana”, and “orange”.
Conclusion
In conclusion, there are multiple techniques and functions available in PHP for converting a string into an array. Each method has its own advantages and use cases, so it’s important to choose the right technique based on the specific requirements of your application. Whether you need to split a string based on a specific delimiter, tokenize a string using a set of delimiters, or use regular expressions for more complex manipulation, PHP provides the tools to accomplish these tasks efficiently.
FAQs
Q: Can I use a custom delimiter with the explode() function?
A: Yes, the explode() function allows you to specify a custom delimiter to split the string into an array.
Q: What is the difference between explode() and preg_split()?
A: explode() uses a simple string as a delimiter, while preg_split() allows you to use a regular expression as a delimiter.
Q: How do I choose the right technique for converting a string to an array?
A: The choice of technique depends on the specific requirements of your application. If you need simple string splitting based on a specific character, explode() or str_split() may be suitable. For more complex manipulation using regular expressions, preg_split() or preg_match_all() can be used. The StringTokenizer class provides a flexible way to tokenize a string using multiple delimiters.