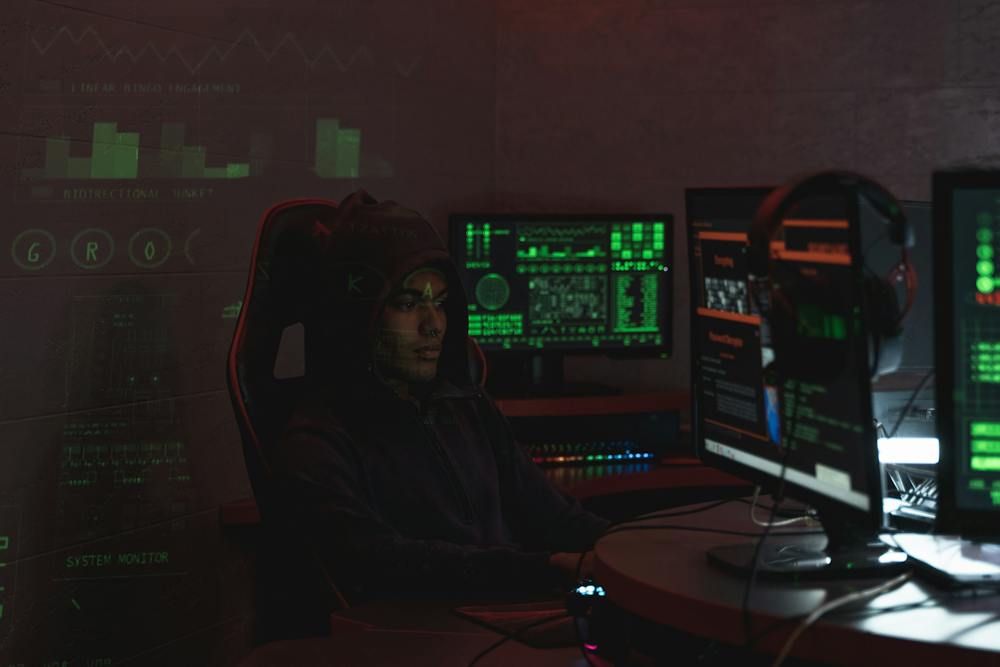
Arrays are a fundamental data structure in JavaScript, and often, we need to compare arrays to find the differences between them. This can be useful for a variety of applications, such as data synchronization, change detection, and more. In this article, we will explore different methods to find array differences in JavaScript.
Method 1: Using a For Loop
One of the simplest ways to find the differences between two arrays is by using a for loop. We can iterate through each element of one array and check if IT exists in the other array. If it doesn’t, we add it to a new array representing the differences.
“`javascript
function findArrayDifferences(arr1, arr2) {
let differences = [];
for (let i = 0; i < arr1.length; i++) {
if (!arr2.includes(arr1[i])) {
differences.push(arr1[i]);
}
}
for (let i = 0; i < arr2.length; i++) {
if (!arr1.includes(arr2[i])) {
differences.push(arr2[i]);
}
}
return differences;
}
const array1 = [1, 2, 3, 4];
const array2 = [3, 4, 5, 6];
console.log(findArrayDifferences(array1, array2)); // Output: [1, 2, 5, 6]
“`
This method is straightforward and easy to understand, but it may not be the most efficient for large arrays, as it has a time complexity of O(n^2).
Method 2: Using Filter and Includes
We can also use the filter method along with the includes method to find the differences between two arrays. This approach allows us to achieve the same result with more concise and readable code.
“`javascript
function findArrayDifferences(arr1, arr2) {
const differences = arr1.filter(item => !arr2.includes(item))
.concat(arr2.filter(item => !arr1.includes(item)));
return differences;
}
const array1 = [1, 2, 3, 4];
const array2 = [3, 4, 5, 6];
console.log(findArrayDifferences(array1, array2)); // Output: [1, 2, 5, 6]
“`
Using the filter and includes methods provides a more elegant solution and has a time complexity of O(n), making it more efficient for larger arrays.
Method 3: Using Set Object
Another approach to find array differences is by using the Set object, which allows us to easily perform operations like union, intersection, and difference on arrays.
“`javascript
function findArrayDifferences(arr1, arr2) {
const set1 = new Set(arr1);
const set2 = new Set(arr2);
const differences = […new Set([…arr1, …arr2].filter(item => !(set1.has(item) && set2.has(item))) )];
return differences;
}
const array1 = [1, 2, 3, 4];
const array2 = [3, 4, 5, 6];
console.log(findArrayDifferences(array1, array2)); // Output: [1, 2, 5, 6]
“`
Using the Set object provides a more efficient solution with a time complexity of O(n), similar to the method using filter and includes.
Conclusion
In conclusion, there are multiple ways to find array differences in JavaScript, each with its own advantages and disadvantages. While using a for loop is simple and straightforward, methods utilizing higher-order functions like filter and includes, as well as the Set object, offer more efficient and readable solutions. When working with large arrays, it’s important to consider the time complexity of each method to ensure optimal performance.
FAQs
Q: Are these methods applicable to multi-dimensional arrays?
A: Yes, the methods discussed in this article can be applied to multi-dimensional arrays by modifying the comparison logic for each dimension.
Q: Can these methods be used to find the intersection of arrays as well?
A: Yes, these methods can be adapted to find the intersection of arrays by using the opposite logic of finding differences.
Q: Is there a built-in method in JavaScript to find array differences?
A: No, JavaScript does not have a built-in method specifically for finding array differences, but the methods discussed in this article provide effective solutions.
As demonstrated, there are various methods to find array differences in JavaScript, and each has its own use case. Whether you prioritize simplicity, efficiency, or readability, there is a method that suits your needs. By understanding the different techniques available, you can choose the best approach for your specific requirements.