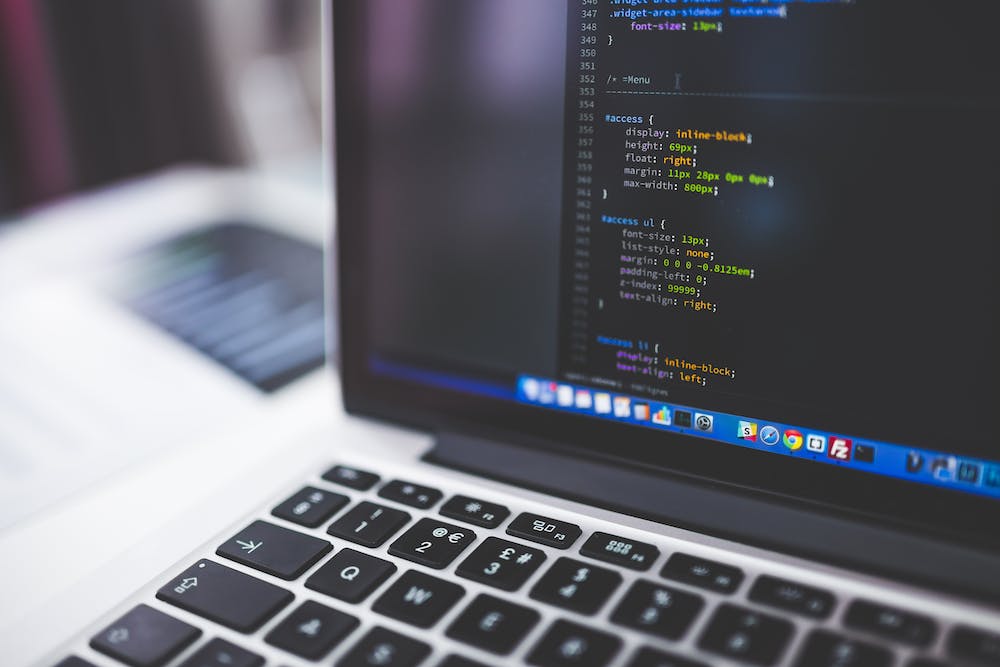
PHP is a powerful programming language that is commonly used for web development. IT provides several built-in functions to work with arrays, which are a fundamental data structure in PHP. At times, you may need to convert an array into a string for various purposes, such as data storage, display, or manipulation. In this article, we will explore different methods to convert PHP arrays into strings.
Method 1: Using implode() function
The implode()
function in PHP is used to join the elements of an array into a string. IT takes two parameters: a string that will be used as a separator between the elements, and the array to be converted into a string. Here’s an example:
$array = array('apple', 'banana', 'orange');
$string = implode(', ', $array);
echo $string; // Output: apple, banana, orange
In this example, the elements of the array are joined together with a comma and space as the separator.
Method 2: Using serialize() function
The serialize()
function in PHP is used to create a storable representation of a value. IT returns a string containing a byte-stream representation of the value that can be stored anywhere. When used with arrays, IT converts the array into a string that can be stored in a file, a database, or passed to another PHP script. Here’s an example:
$array = array('apple', 'banana', 'orange');
$string = serialize($array);
echo $string; // Output: a:3:{i:0;s:5:"apple";i:1;s:6:"banana";i:2;s:6:"orange";}
In this example, the array is serialized into a string representation that can be stored and later unserialized back into an array.
Method 3: Using JSON functions
PHP provides json_encode()
and json_decode()
functions to work with JSON data. The json_encode()
function converts a PHP array into a JSON string, while the json_decode()
function converts a JSON string into a PHP array. Here’s an example:
$array = array('apple', 'banana', 'orange');
$string = json_encode($array);
echo $string; // Output: ["apple","banana","orange"]
In this example, the array is converted into a JSON string representation, which can be stored, transferred, and later converted back into an array using json_decode()
function.
Method 4: Using foreach loop
Another method to convert a PHP array into a string is by using a foreach
loop to iterate through the array and concatenate its elements into a string. Here’s an example:
$array = array('apple', 'banana', 'orange');
$string = '';
foreach($array as $item) {
$string .= $item . ', ';
}
$string = rtrim($string, ', '); // remove the trailing comma and space
echo $string; // Output: apple, banana, orange
This method gives you more control over the string concatenation process, allowing you to customize the format and structure of the resulting string.
Conclusion
In this article, we have explored different methods to convert PHP arrays into strings. Each method has its own advantages and use cases, and the choice of method depends on the specific requirements of your application. The implode()
function is a straightforward and efficient way to join array elements into a string with a specified separator. The serialize()
function is useful for creating a storable representation of an array that can be easily stored and restored. The JSON functions provide a standardized format for data interchange and are widely used for web APIs and data transfer. Finally, using a foreach
loop gives you more control over the string concatenation process and allows for custom formatting.
FAQs
Q: Can I use these methods to convert multi-dimensional arrays into strings?
A: Yes, all of the methods discussed in this article can be used to convert multi-dimensional arrays into strings. For multi-dimensional arrays, you can use nested loops or recursive functions to traverse the array and convert IT into a string.
Q: Is there a limit to the size of the array that can be converted into a string using these methods?
A: The limitations on the size of the array that can be converted into a string using these methods are based on the memory and performance constraints of your server environment. For very large arrays, IT‘s important to consider memory usage and optimize the conversion process to avoid performance issues.
Q: Which method is the most efficient for converting arrays into strings?
A: The efficiency of a method for converting arrays into strings depends on various factors, such as the size of the array, the complexity of the data, and the specific requirements of your application. In general, the implode()
function is often the most efficient method for joining array elements into a string, while the JSON functions are preferable for data interchange and compatibility with other systems.