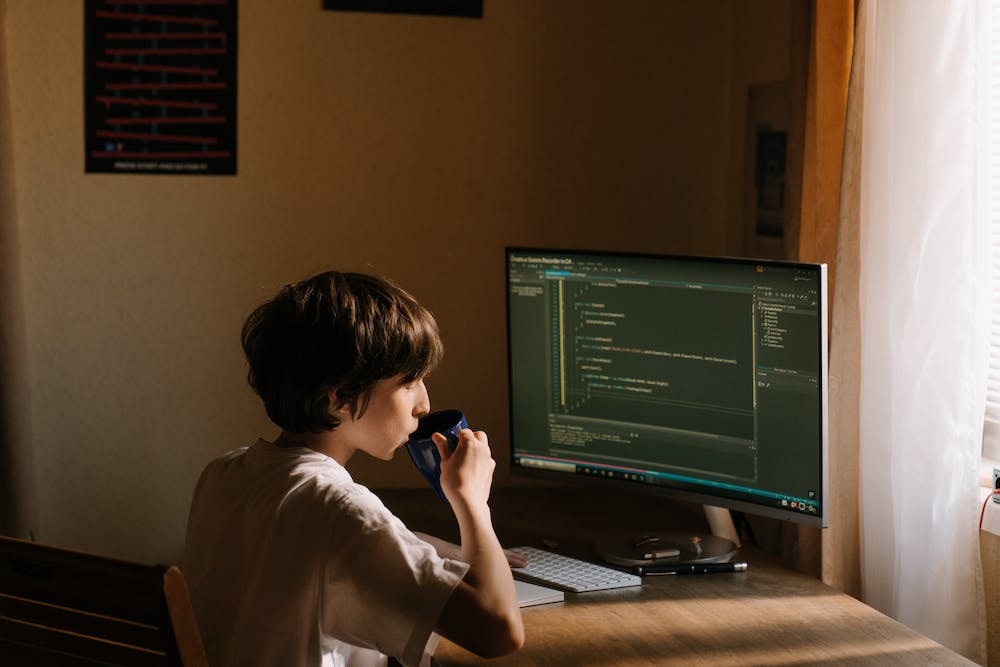
JavaScript is a powerful and versatile programming language that is widely used in web development. One common challenge that developers face is implementing waiting mechanisms to handle asynchronous operations, such as fetching data from an API, or waiting for user input. In this article, we will explore different approaches to waiting in JavaScript, and discuss their strengths and weaknesses.
Using Callbacks
Callbacks are a traditional approach to handling asynchronous operations in JavaScript. With callbacks, you can specify a function to be executed once the asynchronous operation is complete.
Example:
function fetchData(callback) {
// Simulate fetching data from an API
setTimeout(function() {
const data = { name: 'John', age: 30 };
callback(data);
}, 1000);
}
// Usage
fetchData(function(data) {
console.log(data);
});
While callbacks are simple to use, they can lead to callback hell, where you end up with deeply nested functions that are hard to manage and debug. To address this issue, developers have come up with alternative approaches to waiting in JavaScript.
Using Promises
Promises are an improvement over callbacks, providing a more elegant way to handle asynchronous operations. With promises, you can chain then() methods to handle the result of a promise, as well as catch() to handle errors.
Example:
function fetchData() {
return new Promise((resolve, reject) => {
// Simulate fetching data from an API
setTimeout(function() {
const data = { name: 'John', age: 30 };
resolve(data);
}, 1000);
});
}
// Usage
fetchData()
.then(data => {
console.log(data);
})
.catch(error => {
console.error(error);
});
Promises provide cleaner and more readable code compared to callbacks. However, they still have some limitations, such as the inability to cancel a promise once IT‘s been initiated. To address this limitation, the async/await syntax was introduced in ES2017.
Using async/await
Async/await is a modern approach to handling asynchronous operations in JavaScript. It allows you to write asynchronous code that looks and behaves like synchronous code, making it easier to read and maintain.
Example:
async function fetchData() {
// Simulate fetching data from an API
return new Promise(resolve => {
setTimeout(function() {
const data = { name: 'John', age: 30 };
resolve(data);
}, 1000);
});
}
// Usage
async function getData() {
try {
const data = await fetchData();
console.log(data);
} catch (error) {
console.error(error);
}
}
getData();
Async/await is a powerful feature that simplifies the handling of asynchronous operations. It allows you to write asynchronous code in a more synchronous style, making it easier to reason about and debug. However, it’s important to note that under the hood, async/await still utilizes promises.
Using setTimeout and setInterval
Another approach to waiting in JavaScript is using the setTimeout and setInterval functions. These functions allow you to execute a piece of code after a specified delay, or at regular intervals.
Example:
// Using setTimeout
setTimeout(function() {
console.log('Delayed log');
}, 1000);
// Using setInterval
let counter = 0;
const intervalId = setInterval(function() {
console.log('Interval log', counter);
counter++;
if (counter === 5) {
clearInterval(intervalId);
}
}, 1000);
setTimeout and setInterval are useful for scenarios where you need to introduce a delay or periodic execution. However, these functions are not suitable for handling asynchronous operations such as network requests, where you need to wait for a response before continuing.
Choosing the Right Approach
When it comes to waiting in JavaScript, there is no one-size-fits-all solution. The choice of approach depends on the specific requirements of your application and the nature of the asynchronous operation you need to handle.
If you’re working with legacy code or older libraries that use callbacks, you may need to stick with that approach. However, if you’re starting a new project or working with modern frameworks, using promises or async/await is recommended due to their superior readability and error handling capabilities.
For scenarios that involve delaying code execution or periodic tasks, setTimeout and setInterval are suitable options. Just be mindful of their limitations in handling asynchronous operations.
Conclusion
Exploring different approaches to waiting in JavaScript allows developers to choose the best tool for the job based on specific requirements. Whether it’s callbacks, promises, async/await, or setTimeout and setInterval, each approach has its own strengths and weaknesses. By understanding these approaches and their use cases, you can write more robust and maintainable JavaScript code.
FAQs
Q: Can I mix different approaches to waiting in JavaScript?
A: Yes, you can mix different approaches to waiting in JavaScript based on the needs of your application. For example, you can use promises with async/await to handle asynchronous operations and combine that with setTimeout for delaying code execution.
Q: Are there any libraries or tools that can help with waiting in JavaScript?
A: Yes, there are several libraries and tools that can assist with waiting in JavaScript, such as Async.js, Bluebird, and RxJS. Each of these tools provides additional features and utilities to manage asynchronous operations effectively.
Q: How do I handle errors when using different approaches to waiting in JavaScript?
A: When using promises, you can handle errors with the catch() method. With async/await, you can use try/catch blocks to catch and handle errors. For setTimeout and setInterval, you can use traditional error handling techniques such as try/catch or conditional statements.