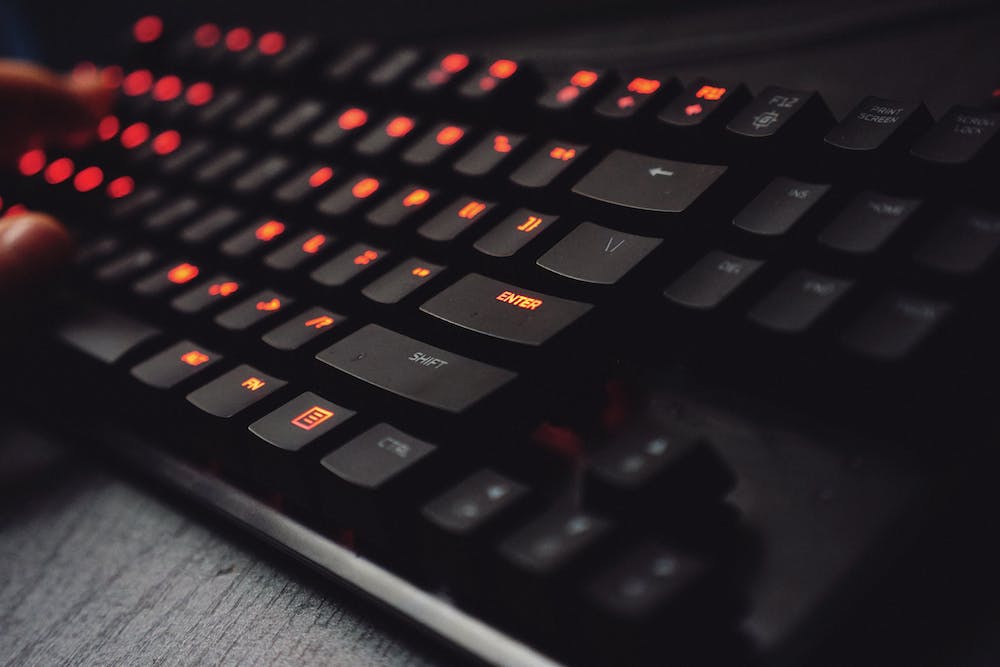
When IT comes to retrieving data from external sources in PHP, cURL (Client URL Library) functions play a crucial role in making the process efficient and reliable. Whether you need to fetch data from an API, interact with a third-party service, or simply scrape a Website, cURL functions provide the necessary tools to accomplish these tasks with ease.
Understanding cURL in PHP
cURL is a library and command-line tool for transferring data with URLs, supporting a wide range of protocols such as HTTP, HTTPS, FTP, FTPS, and more. In PHP, cURL functions enable developers to make requests to remote servers, handle responses, and manage various aspects of the data retrieval process.
Basic cURL Functionality
One of the core functions in cURL is curl_init
, which initializes a new cURL session. This is followed by setting various options such as the URL to be accessed, request method, headers, and data payload using functions like curl_setopt
. Once the options are configured, the actual request is executed using curl_exec
, and the response can be processed as needed.
Handling Response Data
After making a request with cURL, the response from the server can be captured and manipulated using curl_exec
. This allows for parsing the response body, extracting headers, and analyzing the HTTP status code to determine the success or failure of the request.
Efficient Data Retrieval with cURL in PHP
When it comes to efficient data retrieval, cURL functions offer several advantages over traditional methods such as file_get_contents or stream-based approaches. Some of the key benefits include:
- Customized Request Configuration: With cURL, developers have granular control over the request parameters, headers, and other options, allowing for fine-tuning the request according to specific requirements.
- Asynchronous Requests: cURL supports asynchronous requests, making it possible to perform multiple concurrent requests without blocking the execution of the PHP script.
- Cookie Handling: cURL provides built-in support for handling cookies, enabling seamless interaction with services that require cookie-based authentication or session management.
- SSL/TLS Support: Secure communication over HTTPS is simplified with cURL, as it inherently supports SSL/TLS protocols and certificate verification.
Example: Fetching Data from an API
Let’s explore a practical example of using cURL in PHP to retrieve data from a sample API. In this scenario, we’ll make a GET request to the backlink works API to fetch a list of available backlinks. Below is a simplified code snippet demonstrating the process:
<?php
$ch = curl_init('https://api.backlink-works.com/backlinks');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
$backlinks = json_decode($response, true);
// Process the retrieved backlinks as needed
foreach ($backlinks as $backlink) {
// Handle each backlink entry
}
?>
In this example, we initialize a new cURL session with the API endpoint, set the option to return the response as a string with CURLOPT_RETURNTRANSFER
, execute the request, and then process the retrieved JSON data accordingly.
Best Practices for Using cURL in PHP
While cURL functions provide powerful capabilities for data retrieval, there are certain best practices that developers should keep in mind to ensure efficient and reliable usage:
- Error Handling: Always check for errors and handle them gracefully when making cURL requests. This includes verifying the success of the request, handling network or protocol errors, and managing timeouts.
- Resource Cleanup: Properly close cURL sessions and release associated resources using
curl_close
to prevent memory leaks and optimize performance. - Security Considerations: When dealing with sensitive data or API credentials, take precautions to securely store and transmit this information, utilizing features such as secure HTTP headers and SSL/TLS encryption.
Conclusion
Efficient data retrieval is a fundamental aspect of modern web development, and cURL functions in PHP offer a robust solution for handling remote data sources with flexibility and performance. By understanding the core functionality of cURL, leveraging its advanced features, and following best practices, developers can streamline the process of retrieving and interacting with external data, contributing to the overall efficiency and reliability of their PHP applications.
FAQs
Q: Are cURL functions built into PHP by default?
A: Yes, cURL functions are included as part of the standard PHP distribution, enabling developers to utilize them without any additional installation or configuration.
Q: Can cURL be used for sending data as well, not just retrieving it?
A: Absolutely, cURL supports sending various types of data payloads such as form submissions, JSON, XML, and more, making it versatile for both data retrieval and data submission tasks.
Q: Are there any performance considerations when using cURL for large-scale data retrieval?
A: While cURL is generally performant, it’s important to consider factors such as network latency, server load, and resource utilization when dealing with large-scale data retrieval. Asynchronous requests and connection pooling can be valuable techniques to optimize performance in such scenarios.