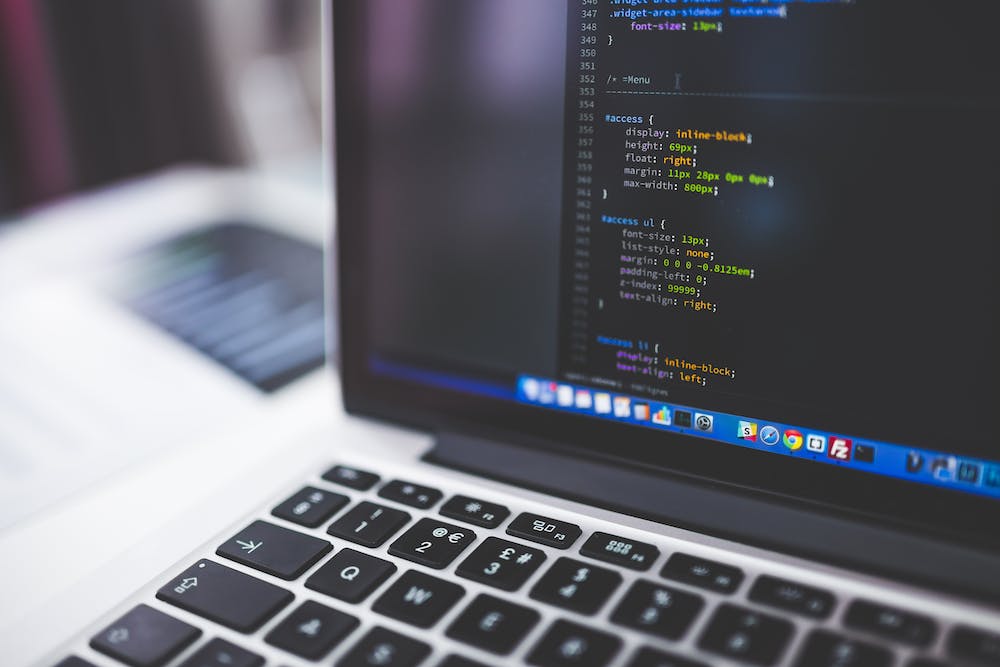
PHP is a powerful programming language for web development, and IT provides a wide range of array manipulation and filtering techniques. In this article, we will explore some advanced array filtering techniques in PHP that will help you manipulate and extract data from arrays efficiently.
1. Filtering Arrays with array_filter()
The array_filter()
function in PHP is used to filter the elements of an array using a callback function. The callback function is applied to each element of the array, and only the elements for which the callback function returns true are included in the resulting array.
Example:
$array = [1, 2, 3, 4, 5, 6];
$filteredArray = array_filter($array, function($value) {
return $value % 2 == 0; // Filter even numbers
});
print_r($filteredArray);
2. Filtering Arrays with array_map()
The array_map()
function in PHP is used to apply a callback function to the elements of an array and return a new array with the modified elements. It can be used to filter an array by applying a callback function that returns only the elements that meet certain criteria.
Example:
$array = [1, 2, 3, 4, 5, 6];
$filteredArray = array_map(function($value) {
return $value * 2; // Double each element
}, $array);
print_r($filteredArray);
3. Filtering Arrays with array_reduce()
The array_reduce()
function in PHP is used to reduce an array to a single value by applying a callback function to each element. It can be used to filter an array by applying a callback function that accumulates the elements that meet certain criteria into a new array.
Example:
$array = [1, 2, 3, 4, 5, 6];
$filteredArray = array_reduce($array, function($carry, $item) {
if ($item % 2 == 0) {
$carry[] = $item; // Accumulate even numbers
}
return $carry;
}, []);
print_r($filteredArray);
4. Filtering Arrays with custom callback functions
In addition to the built-in array filtering functions, you can also create custom callback functions to filter arrays based on specific criteria. This allows you to create more complex filtering logic tailored to your specific requirements.
Example:
function filterByLength($value) {
return strlen($value) > 5; // Filter strings with length greater than 5
}
$array = ["apple", "banana", "orange", "strawberry", "kiwi"];
$filteredArray = array_filter($array, "filterByLength");
print_r($filteredArray);
5. Conclusion
PHP offers a variety of advanced array filtering techniques that allow you to manipulate and extract data from arrays efficiently. By using functions such as array_filter()
, array_map()
, and array_reduce()
, along with custom callback functions, you can easily filter arrays to meet your specific requirements. These techniques are essential for handling large amounts of data in web development and can greatly improve the performance and scalability of your PHP applications.
6. FAQs
Q: Can I use multiple filtering criteria on the same array?
A: Yes, you can chain array filtering techniques together to apply multiple filtering criteria to the same array. For example, you can use array_filter()
to remove certain elements, and then use array_map()
to modify the remaining elements.
Q: Are there any performance considerations when using array filtering techniques?
A: While array filtering techniques are powerful, it’s important to consider the performance implications, especially when working with large arrays. Using custom callback functions or complex logic may impact performance, so it’s essential to test and optimize your code for efficiency.
Q: Can I use array filtering techniques with multidimensional arrays?
A: Yes, array filtering techniques can be applied to multidimensional arrays as well. You can use custom callback functions to filter nested arrays based on specific criteria, or use built-in functions with multidimensional arrays to achieve the desired results.
Now that you’ve learned about advanced array filtering techniques in PHP, you can apply these techniques to manipulate and extract data from arrays in your web development projects. Experiment with different filtering functions and custom callback functions to harness the full power of array manipulation in PHP.