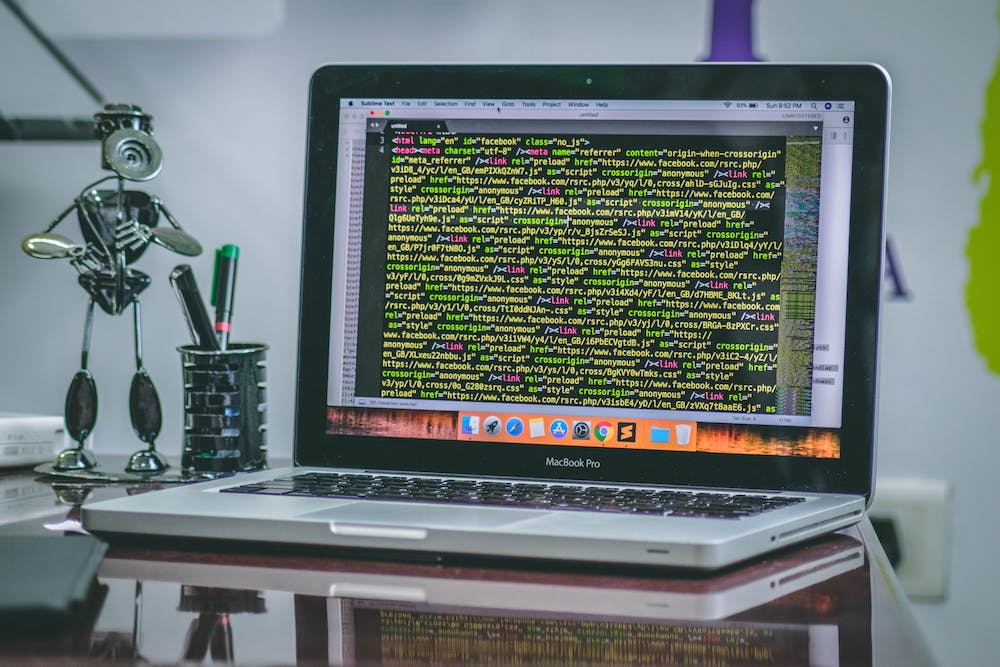
When IT comes to working with arrays in PHP, developers often find themselves in situations where they need to check if a specific value exists in an array. Traditionally, this would be done using a loop or a series of conditional statements. However, in this article, we will explore an array function that can greatly simplify this process and enhance your coding skills.
The Array Includes Function
The array includes function is a powerful tool introduced in PHP 5.1.0. IT allows you to check if an array contains a particular value in a much simpler and more efficient way than traditional methods.
The syntax of the array includes function is as follows:
bool in_array ( mixed $needle, array $haystack [, bool $strict = FALSE ] )
The function takes in three parameters:
- $needle: The value you want to search for in the array.
- $haystack: The array in which you want to search for the value.
- $strict: (Optional) A boolean parameter that specifies whether strict type checking should be used. By default, IT is set to FALSE, which means type conversion will occur during the search.
The array includes function returns TRUE if the value is found in the array and FALSE otherwise.
Examples
Let’s go through a few examples to understand how the array includes function works:
Example 1: Basic Usage
<?php
$fruits = array('apple', 'banana', 'orange');
if (in_array('banana', $fruits)) {
echo 'Found!';
} else {
echo 'Not found!';
}
?>
In this example, we have an array of fruits. We use the array includes function to check if ‘banana’ is present in the array. Since ‘banana’ exists, the output will be ‘Found!’
Example 2: Strict Type Checking
<?php
$numbers = array(1, 2, 3);
if (in_array('2', $numbers, true)) {
echo 'Found!';
} else {
echo 'Not found!';
}
?>
In this example, we have an array of numbers. By setting the third parameter of the array includes function to true, we enforce strict type checking. Since ‘2’ is a string and not an integer like the elements in the array, the output will be ‘Not found!’
Conclusion
The array includes function in PHP is a handy tool that simplifies the process of checking if a value exists in an array. By utilizing this function, you can write more concise and efficient code. So, next time you find yourself needing to check for array values, remember to leverage the power of the array includes function, and revolutionize your coding skills!
FAQs (Frequently Asked Questions)
Q: Can the array includes function be used with associative arrays?
A: No, the array includes function is designed to work with indexed arrays only. IT will not work as expected when used with associative arrays.
Q: Is the array includes function case-sensitive?
A: Yes, by default, the array includes function is case-sensitive. If you want to perform a case-insensitive search, you can convert your array and search value to lowercase (or uppercase) before using the function.
Q: Are there any other similar array functions in PHP?
A: Yes, PHP provides other array functions such as array_search and in_array that can be used for different array operations. IT‘s recommended to explore the PHP documentation to learn more about these functions and their specific use cases.