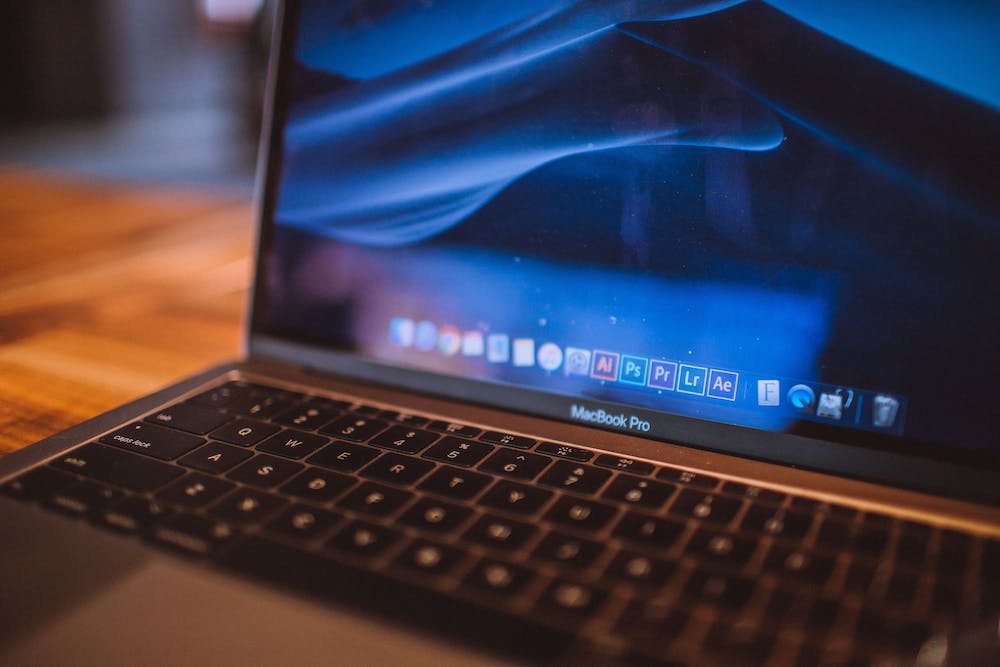
Python is a powerful and versatile programming language that is widely used for various applications, from web development to scientific computing. One of the key features of Python is its exception handling mechanism, which allows developers to handle errors and unexpected situations gracefully.
One of the common ways to handle exceptions in Python is using the ‘try…except’ block. This block enables developers to catch and handle specific exceptions that may occur during the execution of their code. However, there is a lesser-known variation of the ‘except’ clause that can be quite powerful when used correctly – ‘except Exception as e’.
What Does ‘except Exception as e’ Do?
When using the ‘except Exception as e’ clause, Python catches any exception that is derived from the base ‘Exception’ class and assigns IT to the variable ‘e’. This means that you can access the details of the caught exception through the ‘e’ variable, allowing you to inspect the type and message of the exception and take appropriate action based on the specific error that occurred.
Here’s a simple example to illustrate the usage of ‘except Exception as e’:
“`python
try:
# Some code that may raise an exception
result = 10 / 0
except Exception as e:
print(f”An error occurred: {e}”)
“`
In this example, if the division by zero operation raises an exception, it will be caught by the ‘except Exception as e’ clause, and the error message will be printed to the console. This allows the program to continue its execution gracefully without crashing.
Why Use ‘except Exception as e’?
So, why should you use ‘except Exception as e’ instead of the standard ‘except’ clause? The key advantage of using ‘except Exception as e’ is that it provides more granular control over the types of exceptions that you want to catch. By catching exceptions derived from the base ‘Exception’ class, you can handle specific types of errors or even create custom exception classes that inherit from ‘Exception’ to capture and handle unique error scenarios in your code.
Additionally, the ‘e’ variable gives you access to the details of the caught exception, allowing you to log or report the error, retry the operation, or take any other appropriate action based on the specific type of exception that occurred. This level of control can be especially valuable in larger codebases where different modules or libraries may raise different types of exceptions that need to be handled in a uniform manner.
Best Practices for Using ‘except Exception as e’
While ‘except Exception as e’ can be a powerful tool for handling exceptions in Python, it’s important to use it judiciously and follow best practices to ensure that your code remains maintainable and easy to understand.
- Be specific: When catching exceptions using ‘except Exception as e’, try to be as specific as possible about the types of exceptions you want to handle. For example, if you only want to catch division by zero errors, you can create a custom exception class that inherits from ‘Exception’ and use ‘except CustomException as e’ to catch and handle only those specific errors.
- Handle different types of exceptions separately: If your code needs to handle multiple types of exceptions, consider using separate ‘except’ clauses for each type of error rather than lumping them all together under ‘except Exception as e’. This can make your code more readable and maintainable, as it clearly delineates how different types of errors are handled.
- Use logging for error reporting: When catching exceptions using ‘except Exception as e’, consider using Python’s built-in logging module to report the error details, rather than simply printing them to the console. This can make it easier to track and debug errors, especially in production environments.
Conclusion
In conclusion, the ‘except Exception as e’ clause in Python’s exception handling mechanism provides a powerful and flexible way to catch and handle specific types of exceptions in your code. By using this feature judiciously and following best practices for exception handling, you can write more robust and maintainable code that gracefully handles unexpected errors and keeps your applications running smoothly.
FAQs
What is the difference between ‘except Exception’ and ‘except Exception as e’ in Python?
The ‘except Exception’ clause catches any exception derived from the base ‘Exception’ class, but does not provide access to the details of the caught exception. On the other hand, ‘except Exception as e’ assigns the caught exception to the variable ‘e’, allowing you to access and inspect the type and message of the exception.
When should I use ‘except Exception as e’ in my Python code?
You should use ‘except Exception as e’ when you want to handle specific types of exceptions and need access to the details of the caught exception. This can be useful for logging, error reporting, and taking appropriate action based on the specific type of error that occurred.
Can I create custom exception classes to use with ‘except Exception as e’?
Yes, you can create custom exception classes that inherit from the base ‘Exception’ class and use them with ‘except CustomException as e’ to catch and handle specific types of errors in your code.
Is it a good practice to catch all exceptions using ‘except Exception as e’?
While ‘except Exception as e’ can be useful for handling specific types of errors, it’s generally not a good practice to catch all exceptions in this manner. It’s better to be specific about the types of exceptions you want to catch and handle them separately to make your code more maintainable and understandable.