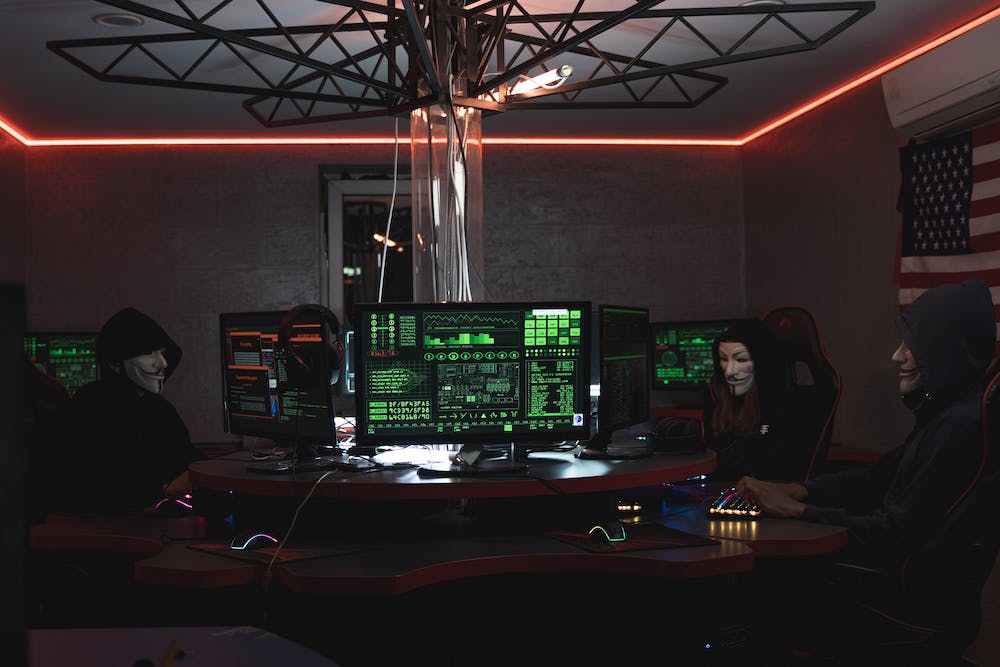
PHP, which stands for Hypertext Preprocessor, is a widely used server-side scripting language for web development. IT provides developers with immense power and flexibility to create dynamic and interactive web applications. Whether you are a beginner or an experienced programmer, exploring unconventional techniques in PHP can help you take your skills to the next level. In this article, we will delve into the mind-blowing secrets of PHP and unveil unconventional techniques that will supercharge your programming skills.
PHP Object-Oriented Programming (OOP)
One of the most powerful techniques in PHP is Object-Oriented Programming (OOP). OOP allows you to organize your code into reusable objects, making IT easier to maintain and extend your applications. By creating classes and objects, you can encapsulate data and behavior, leading to cleaner and more modular code. Here is an example:
“`php
class Car {
private $brand;
private $color;
public function __construct($brand, $color) {
$this->brand = $brand;
$this->color = $color;
}
public function startEngine() {
echo “The $this->brand car with $this->color color has started the engine.”;
}
}
$myCar = new Car(“Toyota”, “blue”);
$myCar->startEngine(); // Output: The Toyota car with blue color has started the engine.
“`
OOP also introduces the concept of inheritance, allowing you to create specialized classes that inherit properties and methods from a parent (base) class. This promotes code reusability and reduces redundancy. Here is an example:
“`php
class Animal {
protected $name;
protected $sound;
public function __construct($name, $sound) {
$this->name = $name;
$this->sound = $sound;
}
public function makeSound() {
echo “The $this->name makes a sound: $this->sound.”;
}
}
class Dog extends Animal {
public function __construct($name) {
parent::__construct($name, “Woof”);
}
}
$myDog = new Dog(“Buddy”);
$myDog->makeSound(); // Output: The Buddy makes a sound: Woof.
“`
PHP Generators
Another mind-blowing secret of PHP is generators. Generators provide an alternative to traditional loops by allowing you to iterate over a set of values without having to create an entire array in memory. This can be extremely useful when dealing with large datasets or when memory optimization is required. Here is an example:
“`php
function fibonacci($limit) {
$a = 0;
$b = 1;
while ($a <= $limit) {
yield $a;
$sum = $a + $b;
$a = $b;
$b = $sum;
}
}
foreach (fibonacci(100) as $number) {
echo $number . ” “;
}
// Output: 0 1 1 2 3 5 8 13 21 34 55 89
“`
PHP Closures
Closures, also known as anonymous functions, allow you to create functions without having to explicitly name them. This can be handy when you need to define small, one-time use functions within your code. Closures can also capture variables from the surrounding scope, making them highly flexible. Here is an example:
“`php
$multiplier = 2;
$double = function($number) use ($multiplier) {
return $number * $multiplier;
};
echo $double(5); // Output: 10
“`
Conclusion
By exploring the mind-blowing secrets of the PHP path, you can unleash the true potential of your programming skills. Object-Oriented Programming enables you to write cleaner and more maintainable code, while generators provide memory optimization and efficient iteration. Closures empower you with the ability to create flexible, anonymous functions on the fly. Incorporating these unconventional techniques into your PHP programming will undoubtedly supercharge your skills and enhance your ability to build amazing web applications.
FAQs
Q: What is PHP?
PHP is a server-side scripting language commonly used for web development. IT allows developers to create dynamic and interactive websites.
Q: What is Object-Oriented Programming (OOP) in PHP?
Object-Oriented Programming is a programming paradigm that organizes code into reusable objects. In PHP, OOP enables code modularity, inheritance, and encapsulation.
Q: How do PHP generators work?
PHP generators allow you to iterate over a set of values without creating an entire array in memory. This is useful for dealing with large datasets and optimizing memory usage.
Q: What are PHP closures?
PHP closures, also known as anonymous functions, allow you to create functions without explicitly naming them. They can also capture variables from the surrounding scope, providing flexibility in coding.
References:
- PHP Manual: https://www.php.net/manual/en/