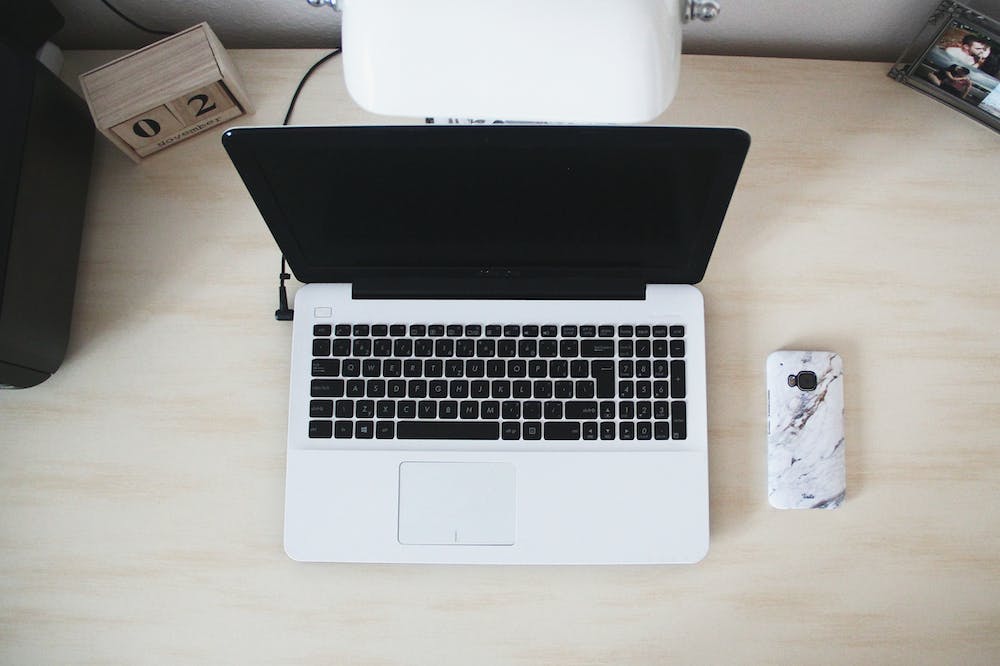
Python is a versatile and powerful programming language that is widely used for numerous applications and development purposes. Its simple syntax and readability make IT an ideal choice for beginners as well as experienced developers. However, there are hidden secrets within the Python programming code that can take your skills to the next level and unleash your coding superpowers. In this article, we will explore some of these secrets and how you can utilize them to optimize your code and become a more proficient Python programmer.
Dynamic Typing
One of the unique features of Python is its ability to dynamically assign types to variables. Unlike other programming languages, Python does not require explicit declaration of variable types. For example:
x = 5
x = "Hello, World!"
In the above code snippet, the variable ‘x’ is initially assigned an integer value, and later IT is reassigned with a string value. This flexibility allows for quick and intuitive code modifications without the need to worry about variable types.
List Comprehension
List comprehension is an elegant and concise way to create lists in Python. IT allows you to generate a list based on existing lists or other iterable objects. Here is an example:
numbers = [1, 2, 3, 4, 5]
squared_numbers = [x * x for x in numbers]
print(squared_numbers) # Output: [1, 4, 9, 16, 25]
In the above code snippet, a new list ‘squared_numbers’ is created by squaring each element of the ‘numbers’ list. List comprehension eliminates the need for traditional loops and reduces the number of lines of code, resulting in cleaner and more readable code.
Generators
Generators are a powerful feature in Python that allow for efficient memory utilization and lazy evaluation. They generate values on the fly, rather than storing them all in memory. This is especially useful when dealing with large datasets or infinite sequences. Here is a simple example of a generator that generates a sequence of Fibonacci numbers:
def fibonacci():
a, b = 0, 1
while True:
yield a
a, b = b, a + b
fib_sequence = fibonacci()
for i in range(10):
print(next(fib_sequence)) # Output: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34
In the above code snippet, the ‘fibonacci’ function is a generator that yields the next Fibonacci number in each iteration. The ‘next’ function is used to retrieve the next value from the generator. Generators enhance performance and efficiency by generating values on-demand, which minimizes memory consumption and improves the overall execution speed of the program.
Conclusion
Python’s simplicity and versatility make IT a popular choice among programmers. By exploring the hidden secrets of Python programming code, you can unlock your coding superpowers and become a more proficient developer. Dynamic typing allows for flexible variable assignments, while list comprehension provides a concise way to create complex lists. Generators optimize memory usage and enable lazy evaluation for efficient handling of large datasets. Keep practicing and experimenting with these features to enhance your Python programming skills and unleash your full coding potential!
FAQs
Q: Is Python a good programming language for beginners?
A: Yes, Python is widely regarded as an excellent programming language for beginners. Its simple and readable syntax helps newcomers grasp fundamental programming concepts easily.
Q: Can I switch variable types in Python?
A: Yes, Python supports dynamic typing, allowing you to switch variable types without explicit declarations or conversions. This flexibility makes Python code more adaptable and concise.
Q: What are the advantages of using generators in Python?
A: Generators optimize memory usage and enable lazy evaluation, making them particularly useful for handling large datasets or infinite sequences efficiently.