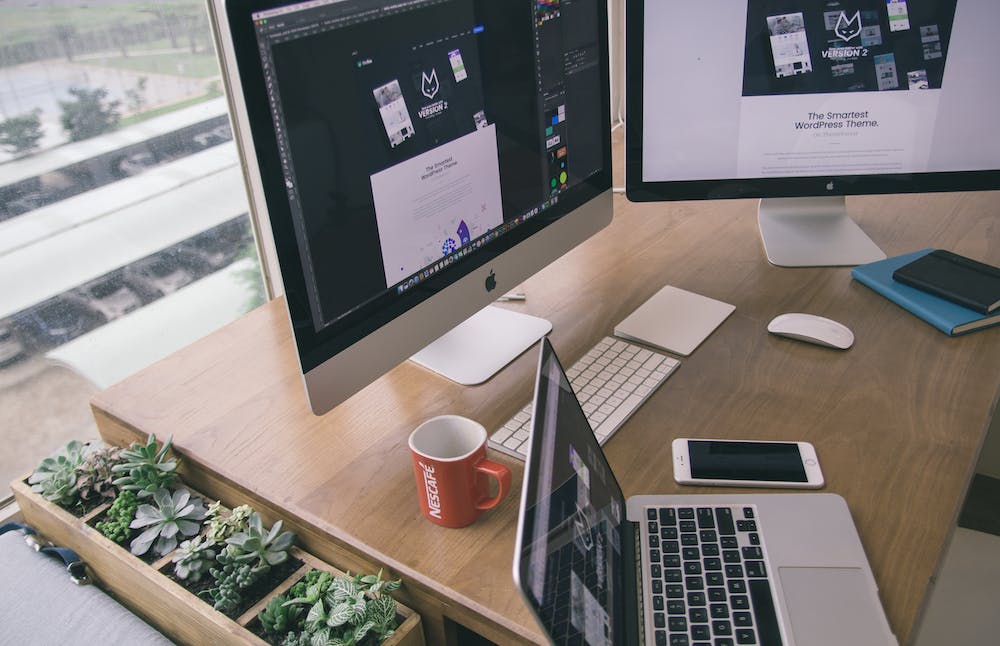
In the world of web development, efficient database manipulation is crucial for creating dynamic and interactive websites. One popular database management system that web developers frequently use is MySQL. To connect PHP with MySQL, the mysqli extension is widely used due to its improved security, performance, and feature set compared to the older mysql extension.
One important aspect of the mysqli extension is the mysqli_result class, which represents the result set obtained from a database query. Understanding the hidden secrets of the mysqli_result class can greatly enhance your ability to manipulate and retrieve data from your MySQL database.
Retrieving Data from the Result Set
After executing a SELECT query, the mysqli_query() function returns a mysqli_result object which contains the result set. To retrieve the rows from the result set, you can use the fetch methods available in mysqli_result:
- fetch_assoc(): Returns an associative array where the keys represent the column names.
- fetch_row(): Returns a numerically indexed array where the indices correspond to the column positions.
- fetch_array(): Returns an array that can be accessed both with column names and position indices.
Let’s consider an example where we have a “users” table with columns “id”, “name”, and “email”. Using fetch_assoc(), we can retrieve the rows as associative arrays:
$mysqli = new mysqli("localhost", "username", "password", "database");
$query = "SELECT * FROM users";
$result = $mysqli->query($query);
while ($row = $result->fetch_assoc()) {
// Accessing values using column names
echo $row['id'] . " " . $row['name'] . " " . $row['email'];
}
?>
Manipulating the Result Set
The mysqli_result class provides various methods to manipulate the result set:
- num_rows: Returns the number of rows in the result set.
- data_seek(): Moves the internal pointer of the result set to a specific row.
- field_seek(): Moves the internal pointer of the result set to a specific field.
- free(): Frees the memory occupied by the result set.
For example, if we want to count the number of rows in the result set:
$query = "SELECT * FROM users";
$result = $mysqli->query($query);
$numRows = $result->num_rows;
echo "Total rows: " . $numRows;
?>
Conclusion
The mysqli_result class is a powerful tool for manipulating and retrieving data from the database. Understanding its functions and methods allows you to have fine-grained control over the result set obtained from a query, enabling efficient data manipulation in your web applications.
FAQs
Q: Can we execute multiple queries using mysqli_result?
A: No, the mysqli_result class can handle the result of only one query at a time. If you need to execute multiple queries, you can use the multi_query() function of the mysqli class.
Q: Is mysqli_result applicable for stored procedure results?
A: Yes, mysqli_result can handle stored procedure results. You can use the next_result() method to move to the next result set if your stored procedure returns multiple result sets.
Q: How can we handle errors related to mysqli_result?
A: You can check for errors using the error property of the mysqli class:
if ($mysqli->error) {
echo "Error: " . $mysqli->error;
}
?>
Understanding the secrets of mysqli_result empowers you to maximize the potential of your MySQL databases. By leveraging the functionalities and methods of the mysqli_result class, you can manipulate and retrieve data efficiently, creating dynamic and interactive web applications.