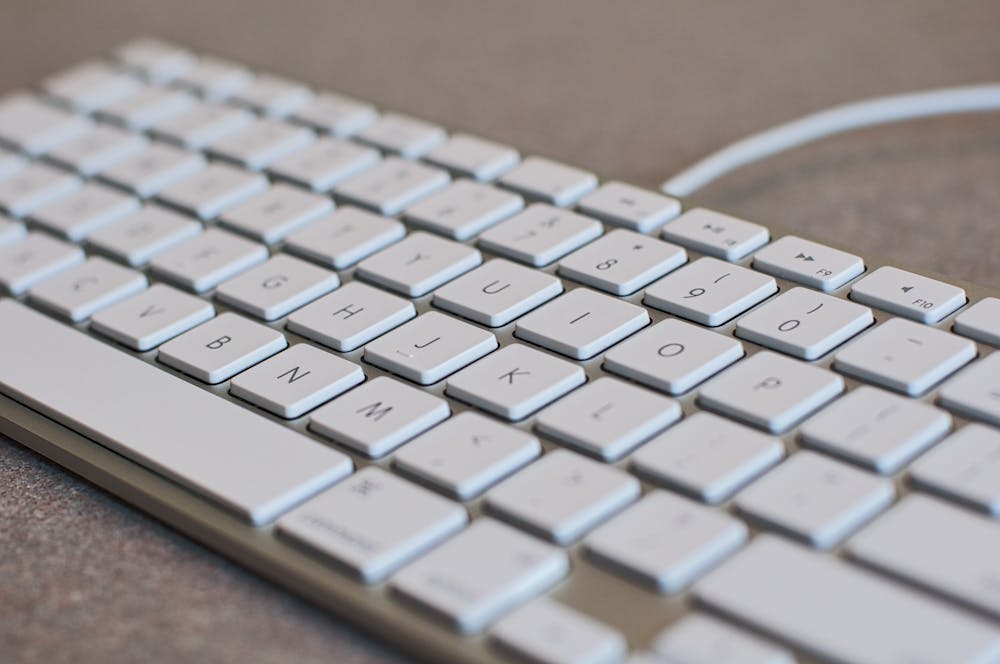
Python is a powerful programming language that is widely used for various applications, including web development, data analysis, artificial intelligence, and more. One of the most fascinating aspects of Python is its simplicity and readability, which makes IT an ideal choice for beginners and seasoned developers alike.
One of the classic programming exercises that many developers encounter is generating the Fibonacci series. The Fibonacci series is a sequence of numbers in which each number is the sum of the two preceding ones, usually starting with 0 and 1. This sequence has fascinated mathematicians and programmers alike for centuries, and with Python, generating the Fibonacci series is a breeze.
Getting Started with Python and Fibonacci
If you’re new to Python, the first step is to install the Python interpreter on your computer. You can download the latest version of Python from the official Website and follow the installation instructions provided. Once Python is installed, you can start writing your first program to generate the Fibonacci series.
Open your favorite text editor or integrated development environment (IDE) and create a new file with a .py extension. You can name the file fibonacci.py or any other name of your choice. In this file, you can start writing your Python program to generate the Fibonacci series.
Writing the Python Program for Fibonacci
Now that you have your development environment set up, you can start writing the Python program to generate the Fibonacci series. The beauty of Python is its simplicity and readability, which makes it easy to understand even for beginners.
Here’s a simple Python program to generate the Fibonacci series:
“`python
def fibonacci(n):
fib_list = [0, 1]
while len(fib_list) < n:
fib_list.append(fib_list[-1] + fib_list[-2])
return fib_list
“`
In this program, we define a function called fibonacci that takes an argument n, which represents the number of elements in the Fibonacci series that we want to generate. We initialize a list called fib_list with the first two numbers of the Fibonacci series, 0 and 1. We then use a while loop to append the sum of the last two numbers in the list to the list until its length reaches n. Finally, we return the list of Fibonacci numbers.
With just a few lines of code, we have a fully functional Python program to generate the Fibonacci series. You can now save the file and run it using the Python interpreter to see the Fibonacci series in action.
Optimizing the Fibonacci Program in Python
While the above program works perfectly fine for generating the Fibonacci series, we can optimize it further to make it more efficient. One way to optimize the Fibonacci program is to use memoization, a technique that stores the results of expensive function calls and returns the cached result when the same inputs occur again.
Here’s an optimized version of the Fibonacci program using memoization:
“`python
def fibonacci_memoization(n, memo={0: 0, 1: 1}):
if n not in memo:
memo[n] = fibonacci_memoization(n-1, memo) + fibonacci_memoization(n-2, memo)
return memo[n]
“`
In this optimized version, we define a new function called fibonacci_memoization that takes an additional argument memo, which is a dictionary that stores the results of previous function calls. If the value of n is not in the memo dictionary, we recursively call the fibonacci_memoization function to calculate the Fibonacci number and store it in the memo dictionary. This way, we avoid redundant calculations and make the program more efficient.
By using memoization, we can significantly improve the performance of the Fibonacci program, especially for large values of n. You can save the optimized program in a new file and run it to see the difference in execution time compared to the non-optimized version.
Conclusion
Python is a versatile programming language that makes it incredibly easy to write programs to solve a wide range of problems, including generating the Fibonacci series. With its simple syntax and powerful features, Python is the perfect choice for beginners and experienced developers alike. Whether you’re a student learning programming for the first time or a professional looking to build complex algorithms, Python has something to offer for everyone.
By following the steps outlined in this article, you can discover the amazing Python program for generating the Fibonacci series and master it with ease. From writing a basic program to optimizing it for better performance, Python gives you the tools to unleash your creativity and solve problems in elegant ways. The Fibonacci series is just one example of the countless possibilities that Python opens up for you, and the journey of exploration and discovery has only just begun.
FAQs
Q: What is the Fibonacci series?
A: The Fibonacci series is a sequence of numbers in which each number is the sum of the two preceding ones, usually starting with 0 and 1.
Q: Why is Python a good choice for generating the Fibonacci series?
A: Python’s simplicity and readability make it easy to understand and write programs for generating the Fibonacci series, making it an ideal choice for both beginners and experienced developers.
Q: How can I optimize the Fibonacci program in Python?
A: You can optimize the Fibonacci program in Python using techniques like memoization, which stores the results of expensive function calls and returns the cached result when the same inputs occur again.