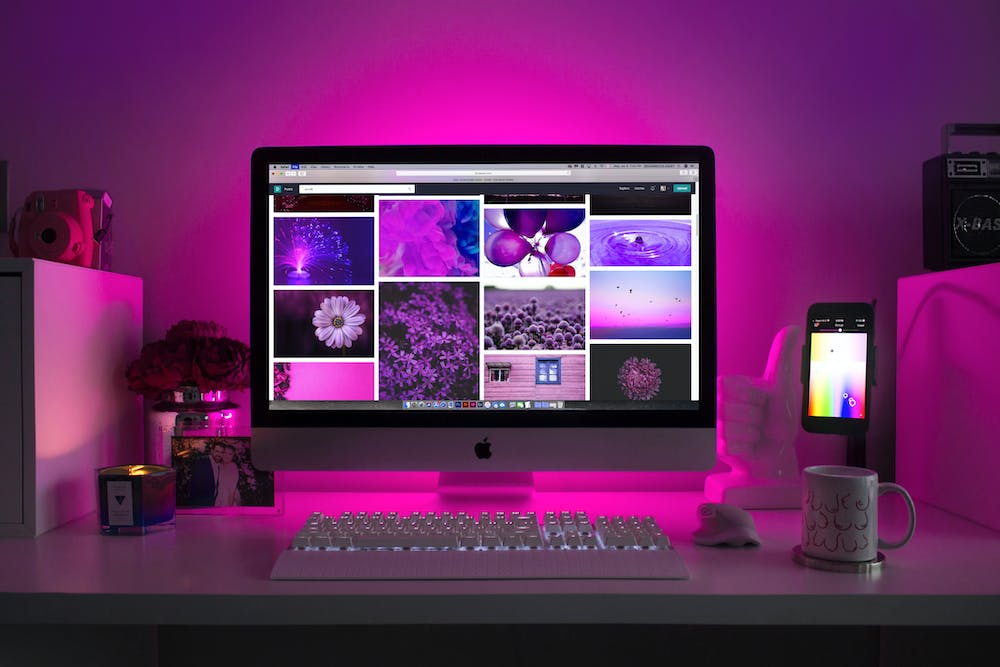
Algorithms are the backbone of computer programming. They are a set of instructions or rules that a computer follows to complete a task. Whether IT‘s sorting a list of numbers, searching for a specific item, or solving a complex mathematical problem, algorithms are at the heart of IT all.
What is an Algorithm?
In simple terms, an algorithm can be defined as a step-by-step procedure to solve a problem or perform a specific task. IT‘s like a recipe that you follow to cook a meal – IT tells you what ingredients to use, how to prepare them, and the order in which to mix and cook them. Similarly, in computer programming, an algorithm outlines the precise steps that need to be taken to achieve a certain outcome.
Let’s consider an example of a sorting algorithm. When you have a list of unsorted numbers, you can use an algorithm to arrange them in ascending or descending order. There are various sorting algorithms, such as bubble sort, selection sort, and merge sort, each with its own set of rules and procedures to accomplish the task.
Types of Algorithms
Algorithms can be categorized based on their functionality and application. Some common types of algorithms include:
- Searching Algorithms: These algorithms are used to find a specific item within a collection of items. Examples include linear search and binary search.
- Sorting Algorithms: These algorithms are used to arrange a list of items in a specific order. Examples include bubble sort, insertion sort, and quicksort.
- Graph Algorithms: These algorithms are used to solve problems related to graphs, such as finding the shortest path between two nodes or determining if a graph is connected.
- Recursive Algorithms: These algorithms are based on the concept of recursion, where a function calls itself to solve smaller instances of the same problem.
Understanding Algorithm Complexity
One important aspect of algorithms is their complexity, which refers to the amount of time and resources required to execute them. The complexity of an algorithm can be analyzed in terms of its time complexity and space complexity.
Time complexity measures the amount of time IT takes for an algorithm to complete its task, based on the size of the input. IT is often expressed using Big O notation, which provides an upper bound on the growth rate of an algorithm’s running time.
Space complexity, on the other hand, measures the amount of memory space an algorithm requires to execute. IT evaluates the usage of memory as the input size increases and provides insights into how efficient the algorithm is in terms of memory usage.
How to Program Algorithms
Programming algorithms involves translating the step-by-step procedures into code that a computer can understand and execute. This is typically done using a programming language such as Python, Java, C++, or JavaScript.
Let’s take the example of a simple sorting algorithm, such as bubble sort, and see how IT can be implemented in Python:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
In this Python code, we define a function called bubble_sort
that takes an array arr
as input. The function then iterates through the array and compares adjacent elements, swapping them if they are in the wrong order. This process is repeated until the entire array is sorted.
IT‘s important to note that algorithms can be implemented in different ways and using different programming languages, so there is often more than one way to achieve the same result.
Conclusion
Algorithms are an essential part of computer programming, and understanding them is crucial for anyone looking to delve into the world of programming. Whether you’re a beginner or an experienced programmer, having a solid grasp of algorithms and their complexities can help you write more efficient and optimized code.
By breaking down complex problems into smaller, more manageable steps, algorithms enable us to solve a wide range of computational problems and build software applications that are reliable and scalable. So, the next time you’re faced with a programming challenge, remember that algorithms are your best friends in finding the most effective solution.
FAQs
1. What is the importance of algorithms in programming?
Algorithms form the foundation of computer programming by providing a systematic approach to solving problems and performing tasks. They enable programmers to write efficient and optimized code, leading to better-performing software applications.
2. Are there any resources to learn more about algorithms?
Yes, there are plenty of resources available for learning about algorithms, including online courses, books, and tutorials. Websites such as Coursera, Udemy, and Khan Academy offer comprehensive courses on algorithms and data structures.
3. Can algorithms be implemented in any programming language?
Yes, algorithms can be implemented in a wide variety of programming languages. Each language may offer its own set of features and syntax to write and execute algorithms, but the fundamental principles remain the same across languages.