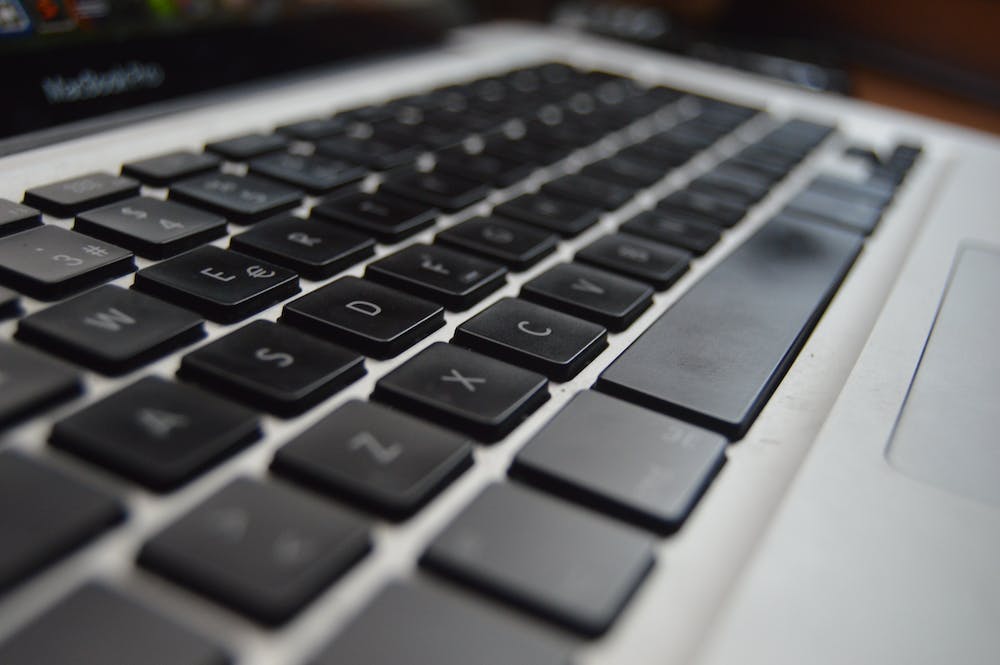
Python is a widely-used programming language known for its simplicity and readability. Python source code, written in .py files, contains the instructions that are executed by the Python interpreter. In this article, we will take a deeper look at Python source code and discuss how to effectively read, understand, and decode IT.
Understanding Python Source Code
Python source code is composed of statements that instruct the Python interpreter on how to perform specific tasks. These statements can include variable assignments, function definitions, control structures, and more. Let’s take a closer look at some of the key components of Python source code:
1. Variables and Data Types
Variables in Python are used to store data that can be accessed and manipulated throughout the program. The data stored in variables can be of various types, such as integers, floating-point numbers, strings, lists, tuples, dictionaries, and more. Here’s an example of Python source code that demonstrates variable assignments and data types:
# Variable assignments
name = "John"
age = 25
height = 5.11
# Data types
print(type(name)) # Output: <class 'str'>
print(type(age)) # Output: <class 'int'>
print(type(height)) # Output: <class 'float'>
2. Functions and Control Structures
Functions in Python are reusable blocks of code that perform specific tasks. They can take input arguments, process them, and return results. Control structures, such as if-else statements, loops, and exception handling, are used to control the flow of execution in a Python program. Here’s an example of Python source code that demonstrates function definitions and control structures:
# Function definition
def greet(name):
return "Hello, " + name + "!"
# Control structures
if age >= 18:
print(greet(name)) # Output: Hello, John!
else:
print("You are too young!")
Reading and Decoding Python Source Code
Reading and understanding Python source code is essential for both beginners and experienced developers. Here are some effective strategies for decoding Python source code:
1. Follow the Flow of Execution
When reading Python source code, it’s important to follow the flow of execution to understand how the program behaves. Start from the top of the file and track how the program progresses through different statements, functions, and control structures.
2. Pay Attention to Indentation
Python uses indentation to define blocks of code, such as function bodies, loops, and conditional statements. Pay close attention to the indentation level to understand the structure of the program and how different code blocks are nested within each other.
3. Use Descriptive Variable Names
Descriptive variable names can greatly enhance the readability of Python source code. When decoding Python, look for variable names that provide insight into the data they represent and the purpose they serve within the program.
4. Understand Function Definitions and Calls
Functions are the building blocks of Python programs. When decoding Python source code, it’s important to understand how functions are defined, called, and utilized to perform specific tasks within the program.
Optimizing Python Source Code
Optimizing Python source code involves making it more efficient, readable, and maintainable. Here are some best practices for optimizing Python source code:
1. Write Readable and Maintainable Code
Follow the PEP 8 style guide for Python code to ensure consistent and readable formatting. Use descriptive variable and function names, and include comments where necessary to explain complex logic or algorithms.
2. Leverage Built-in Functions and Libraries
Python provides a rich set of built-in functions and standard libraries that can be leveraged to perform common tasks without reinventing the wheel. Utilize these resources to optimize your source code and reduce unnecessary complexity.
3. Employ Data Structures and Algorithms Effectively
Choose the most appropriate data structures and algorithms to accomplish the desired functionality in an efficient and maintainable manner. Understanding the strengths and weaknesses of different data structures and algorithms is key to optimizing Python source code.
4. Profile and Benchmark Your Code
Use profiling and benchmarking tools to identify performance bottlenecks and areas for optimization in your Python source code. By analyzing the execution time and memory usage of different code segments, you can prioritize optimization efforts effectively.
Conclusion
In conclusion, Python source code is the fundamental building block of Python programs, and understanding how to read, decode, and optimize it is essential for becoming a proficient Python developer. By following best practices and leveraging effective strategies, you can improve the readability, efficiency, and maintainability of your Python source code, ultimately leading to better software development outcomes.
FAQs
Q: Why is it important to understand Python source code?
A: Understanding Python source code is crucial for writing, maintaining, and troubleshooting Python programs. It allows developers to comprehend the logic and flow of the code, make informed modifications, and identify and fix bugs effectively.
Q: How can I improve my ability to decode Python source code?
A: Improving your ability to decode Python source code involves practicing reading and analyzing different types of Python programs, learning from experienced developers, and seeking feedback on your own code. Additionally, familiarizing yourself with common Python idioms and patterns can enhance your decoding skills.
Q: What tools can I use to optimize Python source code?
A: There are various tools and resources available for optimizing Python source code, including code linters, static analyzers, profiling and benchmarking tools, and performance testing frameworks. These tools can help identify areas for improvement and guide optimization efforts effectively.