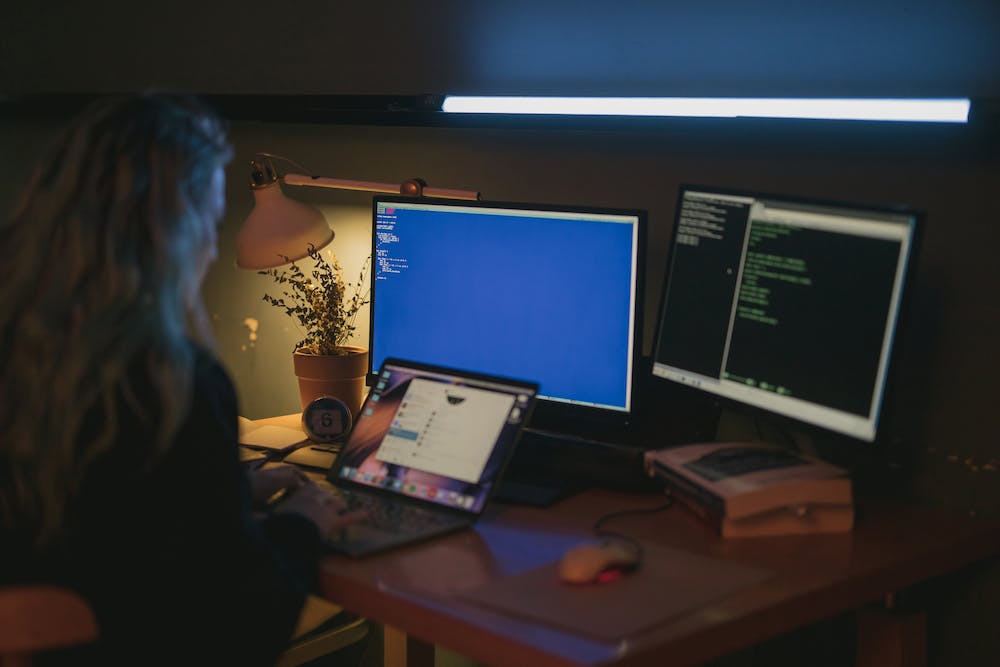
Creating user-interactive web pages using PHP and HTML is an essential skill for web developers. PHP is a server-side scripting language that is used to create dynamic web pages, while HTML is a markup language used to structure content on the web. By combining these two technologies, developers can create web pages that respond to user actions and input, providing a rich and engaging user experience.
Getting Started with PHP and HTML
To create user-interactive web pages, you’ll need a basic understanding of PHP and HTML. PHP is a scripting language that runs on the server, allowing you to generate dynamic content, interact with databases, and handle forms. HTML, on the other hand, is used to create the structure and layout of web pages, including text, images, and other media.
When creating user-interactive web pages, you’ll typically use HTML to define the content and structure of the page, and then use PHP to add dynamic functionality. This can include processing form submissions, updating content based on user input, and interacting with databases to retrieve or store information.
Adding User-Interactive Elements with PHP and HTML
One of the most common ways to create user-interactive web pages is by using forms. HTML provides the