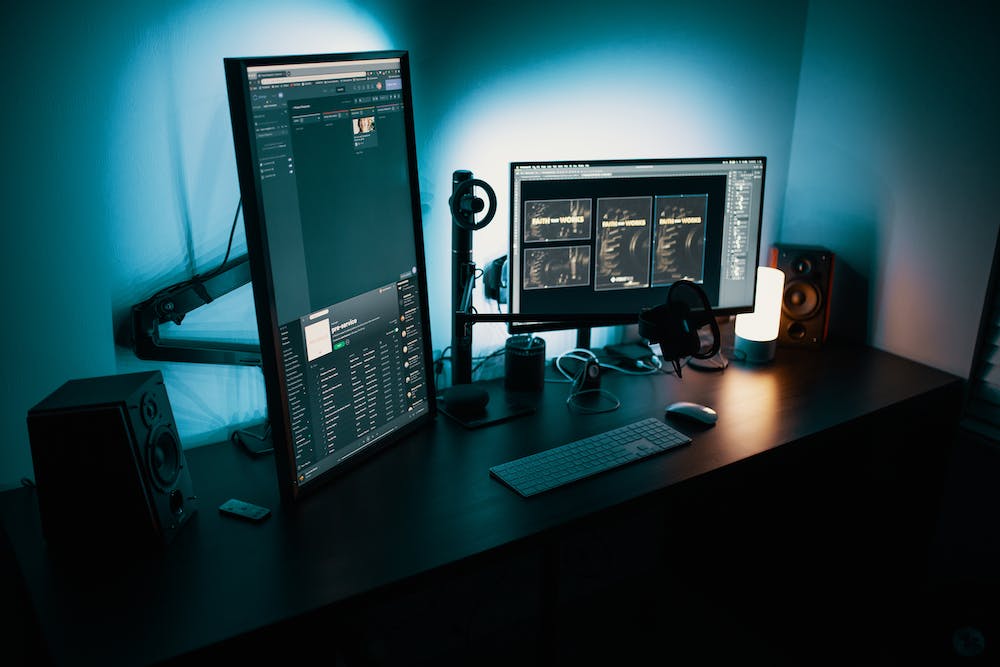
Laravel Faker is a fantastic library that simplifies the process of creating realistic dummy data in Laravel applications. IT provides a wide range of methods to generate data for various data types such as names, email addresses, addresses, phone numbers, and even random text. This article will guide you through the process of using Laravel Faker to generate realistic dummy data and provide answers to common questions that developers often have.
Getting Started with Laravel Faker
To begin using Laravel Faker, you need to install IT as a dependency in your Laravel project. Open your terminal and navigate to your project directory. Then, run the following command to install Faker using Composer:
composer require fakerphp/faker
Once Faker is installed, you need to import IT into your PHP file. Open the file where you want to generate dummy data and add the following line at the top:
use Faker\Factory;
Generating Dummy Data
With Laravel Faker imported, you can now start generating dummy data using its various methods. Let’s say you want to create dummy users for testing purposes. You can use the name
, email
, and password
methods to generate random values for these fields. Here’s an example:
$faker = Factory::create();
$name = $faker->name;
$email = $faker->email;
$password = $faker->password;
The name
method generates a random name, the email
method generates a random email address, and the password
method generates a random password. You can then use these generated values to populate your database or any other use case you have.
Customizing Generated Data
Often, you’ll need to generate specific types of data. Laravel Faker allows you to customize the generated data to fit your needs. For example, if you want to generate random paragraphs of text instead of just random words, you can use the paragraph
method:
$faker = Factory::create();
$paragraph = $faker->paragraph;
Similarly, you can customize the number of words or sentences in the generated text by passing the desired count as a parameter:
$faker = Factory::create();
$threeWords = $faker->words(3);
$twoSentences = $faker->sentences(2);
Common FAQs
1. Can I generate dummy data for non-English languages?
Yes, Laravel Faker supports generating data in various languages. You can use the locale
method to set the desired language. For example, to generate data in French, you can do the following:
$faker = Factory::create('fr_FR');
2. How can I generate dummy images?
Laravel Faker provides a method called image
that generates a URL to a random placeholder image. You can specify the desired width and height for the generated image as parameters. Here’s an example:
$faker = Factory::create();
$imageUrl = $faker->image(400, 300);
3. Can I generate dummy data for specific fields, such as dates?
Yes, Laravel Faker provides methods for generating data for specific fields, such as date
or dateTime
. You can pass a date format as a parameter to generate a date in the desired format. Here’s an example:
$faker = Factory::create();
$date = $faker->date('Y-m-d');
$dateTime = $faker->dateTime('now');
In conclusion, Laravel Faker is a powerful tool that simplifies the generation of realistic dummy data in Laravel applications. IT provides a wide range of methods to generate data for various data types and allows customization to fit your specific requirements. Incorporating Laravel Faker into your development workflow can greatly speed up the process of testing and populating your database with relevant data.